Build a Calculator with React, Tailwind, and Django
Last Updated :
23 Jan, 2024
This article will guide you in creating a calculator using React and Tailwind with the Django Framework. We’ll explore the integration of Django, React, and Tailwind, and go through the step-by-step process of implementing the calculator.
What is a Calculator?
A calculator is a device or tool designed for performing mathematical calculations. It typically consists of numerical buttons, mathematical operators (such as addition, subtraction, multiplication, and division), and a display screen. Calculators are used to quickly and accurately perform various mathematical operations, ranging from simple arithmetic to more complex calculations.
Calculator using React, Tailwind, and Django Framework
Here, is the step-by-step implementation of Calculator using React, Tailwind, and Django Framework. Here, we will cover the article into 2 parts, frontend and then backend. To install Django follow these steps.
Backend Using Django
To start the project and app use this command
django-admin startproject calculator_backend
cd calculator_backend
python manage.py startapp calculator
Now add this app to the ‘settings.py’
INSTALLED_APPS = [
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"calculator",
'corsheaders',
]
For install the corsheaders run the below command
pip install django-cors-headers
File Strcutrue :
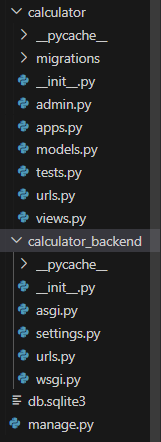
Setting Necessary Files
views.py : In below code `calculate` view function in this Django code handles POST requests containing a JSON payload with a mathematical expression. The function evaluates the expression using the `eval` function and returns the result as a JSON response. If there’s an error during evaluation, it returns an error message.
Python3
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
import json
@csrf_exempt
def calculate(request):
if request.method = = 'POST' :
data = json.loads(request.body)
expression = data.get( 'expression' , '')
try :
result = str ( eval (expression))
return JsonResponse({ 'result' : result})
except Exception as e:
return JsonResponse({ 'error' : str (e)})
else :
return JsonResponse({ 'error' : 'Invalid request method' })
|
project/urls.py: here, Django code configures a single URL route, linking the ‘calculate/’ endpoint to the ‘calculate’.
Python3
from django.contrib import admin
from django.urls import path,include
from .views import *
urlpatterns = [
path( 'calculate/' , calculate, name = 'calculate' ),
]
|
app/urls.py: Here, Django code defines URL patterns, routing requests to the Django admin interface at ‘/admin/’ and including additional patterns from the ‘calculator.urls’ .
Python3
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path( 'admin/' , admin.site.urls),
path(' ', include(' calculator.urls'))
]
|
settings.py : In settings.py we added the crosheaders Middleware and also the some allowing host for integrating react.
Python3
MIDDLEWARE = [
............................... ......................... ...............
............................ ......................... .....................
'corsheaders.middleware.CorsMiddleware' ,
]
CORS_ALLOWED_ORIGINS = [
]
CORS_ALLOW_CREDENTIALS = True
|
Frontend Using React + Tailwind
To start the project in react use this command
npx create-react-app calculator_frontend
cd calculator_frontend
Install the necessary library tailwindcss using the below command
npm install tailwindcss
File Structure :
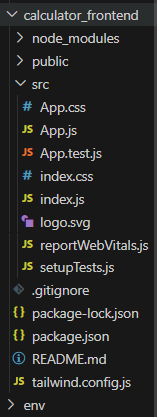
Creating User InterFace
App.css: In below CSS code styles a React application, centering text, setting a rotating animation for the logo, styling the header, and defining link colors. The design is responsive, featuring a rotating logo with reduced motion support.
CSS
.App {
text-align : center ;
}
.App-logo {
height : 40 vmin;
pointer-events: none ;
}
@media (prefers-reduced-motion: no-preference) {
.App-logo {
animation: App-logo-spin infinite 20 s linear;
}
}
.App-header {
background-color : #282c34 ;
min-height : 100 vh;
display : flex;
flex- direction : column;
align-items: center ;
justify- content : center ;
font-size : calc( 10px + 2 vmin);
color : white ;
}
.App-link {
color : #61dafb ;
}
@keyframes App-logo-spin {
from {
transform: rotate( 0 deg);
}
to {
transform: rotate( 360 deg);
}
}
|
App.js: This React code defines a calculator app using Django, React, and TailwindCSS. It utilizes state hooks for managing user input and displaying the calculation result. Functions handle button clicks, clearing input, and triggering calculations through a Django backend. The JSX renders a visually appealing UI with input, buttons, and dynamic styling using TailwindCSS, creating an interactive calculator with integrated frontend and backend functionality.
Javascript
import React, { useState } from 'react' ;
import './App.css' ;
function App() {
const [expression, setExpression] = useState( '' );
const [result, setResult] = useState( '' );
const handleButtonClick = (value) => {
setExpression((prev) => prev + value);
};
const clear = () => {
const newExpression = expression.slice(0, -1);
setExpression(newExpression);
}
const clearAll = () => {
setExpression( '' );
}
const handleCalculate = async () => {
try {
method: 'POST' ,
headers: {
'Content-Type' : 'application/json' ,
},
body: JSON.stringify({ expression }),
});
const data = await response.json();
setResult(data.result);
} catch (error) {
console.error( 'Error calculating:' , error);
}
};
return (
<div className= "App max-w-3xl mx-auto" >
<div>
<h1 className= 'mt-4 mb-4' >Django + React + TailwindCSS Calculator</h1>
<div className= 'border border-b-2 p-12 relative rounded-md shadow-sm' >
{ }
<input
type= "text"
value={expression}
className= "absolute focus:outline-none left-10 text-lg"
/>
{ }
<div className= 'flex space-x-4 absolute bottom-4 right-4' >
<button onClick={clear} className= 'bg-red-400 text-sm text-white border rounded-full px-4 py-2' >Clear</button>
<button onClick={clearAll} className= 'bg-red-400 text-sm text-white border rounded-full px-4 py-2' >Clear All</button>
</div>
{ }
<div className= 'absolute top-3 right-6' >
<h1>{result}</h1>
</div>
</div>
</div>
{ }
<div className= "grid grid-cols-4 gap-10 border bg-gray-300 rounded-md p-12 shadow-xl" >
{ }
{[7, 8, 9, '/' ].map((value) => (
<button className= 'border p-4 bg-white rounded-full' key={value} onClick={() => handleButtonClick(value)}>
{value}
</button>
))}
{[4, 5, 6, '*' ].map((value) => (
<button className= 'border p-4 bg-white rounded-full' key={value} onClick={() => handleButtonClick(value)}>
{value}
</button>
))}
{[1, 2, 3, '-' ].map((value) => (
<button className= 'border p-4 bg-white rounded-full' key={value} onClick={() => handleButtonClick(value)}>
{value}
</button>
))}
{[0, '.' , '=' , '+' ].map((value) => (
<button className={`${value === '=' && 'border-red-300 border-8' } border p-4 bg-white rounded-full`}
key={value}
onClick={value === '=' ? handleCalculate : () => handleButtonClick(value)}
>
{value}
</button>
))}
</div>
</div>
);
}
export default App;
|
App.test.js: This test script is checking whether the “learn react” link is rendered in the React component specified by the `App` import. It uses the `render` function from `@testing-library/react` to render the component, and then it uses `screen.getByText` to find an element containing the text “learn react.”.
Javascript
import { render, screen } from '@testing-library/react' ;
import App from './App' ;
test( 'renders learn react link' , () => {
render(<App />);
const linkElement = screen.getByText(/learn react/i);
expect(linkElement).toBeInTheDocument();
});
|
index.css : Below, syntax is configuring Tailwind CSS in a single file, including base styles, components, and utilities to apply the framework’s styling to the project.
CSS
@tailwind base;
@tailwind components;
@tailwind utilities;
|
Deployement of the Project
Run the server with the help of following command:
python3 manage.py runserver
npm start
Output :
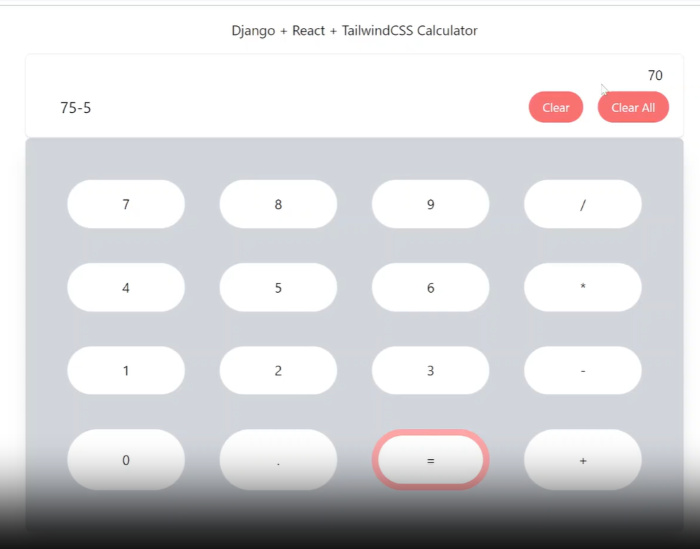
Video Demonstration
Share your thoughts in the comments
Please Login to comment...