Browser Object Model
Last Updated :
22 Jan, 2024
Browser Object Model (BOM) is a programming interface JavaScript tool for working with web browsers. This enables access & manipulation of the browser window, frames, and other browser-related objects by facilitating the JavaScript code.
The main object is “window,” which helps to interact with the browser. By default, you can call window functions directly.
window.alert(" Hey Geeks");Â
// Same as
alert(" Hey Geeks ");Â Â
Note: It is not recommended always to explicitly mention “window” because it’s the default. So, the alert(” Hey Geeks “); is equivalent to the longer version.
Browser Object Model Types
Window Object Model |
Represents the browser window and serves as the main tool for interaction within the Browser Object Model (BOM). |
History Object Model |
Enables navigation control by keeping track of the user’s browsing history in the Browser Object Model (BOM). |
Screen Object Model |
Offers information about the user’s screen, like its size, within the Browser Object Model (BOM). |
Navigator Object Model |
Provides details about the user’s browser and system characteristics within the Browser Object Model (BOM). |
Location Object Model |
Manages the current web address (URL) and allows changes within the Browser Object Model (BOM). |
Window Object
The ‘window object in JavaScript represents your web browser’s window. Everything you create in JavaScript, like variables and functions, is automatically part of this window.
Global variables are like items in the window, and global functions are tools you can use from anywhere. The “document” object, which deals with HTML content, is also a special tool in the window. So, think of the “window” as a big container holding all your JavaScript stuff.
The HTML DOM Objects can be treated as the window object’s property
window.document.getElementById("header");
which is the same as
document.getElementById("header");
Window Size
You can use two properties to find out the dimensions of the browser window, and both provide the measurements in pixels:
window.innerHeight |
This gives you the vertical size or height of the browser window in pixels. |
window.innerWidth |
This gives you the horizontal size or width of the browser window in pixels. |
Other Window Methods
window.open() |
This method is used to open a new tab or window with the specified URL and name. |
window.close() |
This method is used for closing a certain window or tab of the browser that was previously opened by the window.open( ) method. |
window.moveTo() |
This method is used in the window to move the window from the left and top coordinates. |
window.resizeTo() |
This method is used to resize a window to the specified width and height |
Example: Implementation to show current window size.
HTML
<!DOCTYPE html>
< html >
< head >
< style >
h1 {
color: green;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< p id = "geeks" ></ p >
< script >
document.getElementById("geeks").innerHTML =
"Browser inner window width: "
+ window.innerWidth + "px< br >" +
"Browser inner window height: "
+ window.innerHeight + "px";
</ script >
</ body >
</ html >
|
Output:
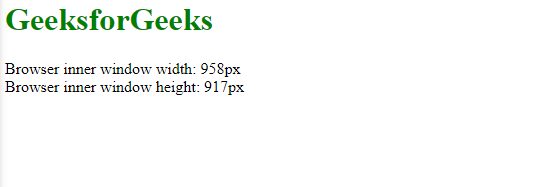
 Advantages & Disadvantages of BOM
Advantages
- User Interaction: BOM allows websites to show alerts, prompts, and confirmations, making them more interactive.
- Navigation Control: It also helps to manage going back or forward in your browsing history using tools like ‘history.’
- Browser Details: BOM gives information about your browser through the ‘navigator’ tool, helping to adjust content for different browsers and systems.
- Location Management: Using the ‘location’ tool, BOM lets websites change web addresses for dynamic content and easy redirection.
Disadvantages
- Browser Compatibility: It may work differently in various browsers, causing compatibility issues.
- Security Concerns: Some BOM features, like pop-ups, can be exploited for security risks.
- Client-Side Dependency: It relies heavily on the client side, hence affecting performance.
- Limited Standardization: It lacks a standardized specification, leading to variations in implementation.
Share your thoughts in the comments
Please Login to comment...