Flyweight Design Pattern – JavaScript Design Pattern
Last Updated :
31 Oct, 2023
The Flyweight Design Pattern is a structural design pattern used in JavaScript to minimize memory usage by sharing the data as much as possible with the related objects.
Important Topics for Flyweight Design Pattern
The shared data typically consists of two types of properties:
- Intrinsic properties: The properties that do not change over time while shared among related objects are called Intrinsic properties
- Extrinsic properties: The properties that are unique to every, object and cannot be shared are called extrinsic properties.
Implementation of Flyweight Design Pattern in JavaScript:
Step 1: First, we must create a Flyweight object with intrinsic and extrinsic properties.
Javascript
class FlyweightDP {
constructor(sharedData) {
this .sharedData = sharedData;
}
operation(uniqueData) {
console.log(`Intrinsic Property: ${ this .sharedData}, Extrinsic Property: ${uniqueData}`);
}
}
|
Explanation:
In this step, we created a `FlyweightDP` class which contains the constructor to initialize the `sharedData`(Intrinsic properties) and the `operation` method takes the `uniqueData` (Extrinsic properties) and logs a message combining shared and unique data.
Step 2: Create a `FlyweightFactory` class which is used to manage and reuse the shared data and properties efficiently.
Javascript
class FlyweightFactory {
constructor() {
this .data = {};
}
getData(sharedData) {
if (! this .data[sharedData]) {
this .data[sharedData] = new FlyweightDP(sharedData);
}
return this .data[sharedData];
}
getFlyweightsCount() {
return Object.keys( this .data).length;
}
}
|
Explanation:
In this step, we created a `FlyweightFactory` which contains a constructor to initialize an empty object called `data` to store the instances of `FlyweightDP` class. The `getData` method takes the `sharedData` as a parameter and checks whether a flyweight(object/instance of `FlyweightDP` class) with the given `sharedData` exists in the `data` object or not.
If it doesn’t exists it create a new flyweight with that `sharedData` and add it to the `data` object and returns the flyweight associated with that `sharedData`. The `getFlyweightsCount` method returns the count of flyweights or instances of `FlyweightDP` class.
Step 3: Create an object for the `FlyweightFactory` class to understand the working of Flyweight design pattern.
Javascript
const factory = new FlyweightFactory();
const flyweight1 = factory.getData( 'A' );
flyweight1.operation( '1' );
const flyweight2 = factory.getData( 'B' );
flyweight2.operation( '2' );
const flyweight3 = factory.getData( 'A' );
flyweight3.operation( '3' );
console.log(`Number of flyweights created: ${factory.getFlyweightsCount()}`);
|
Explanation:
In this step we created an object called `factory` for the `FlyweightFactory` class and invoked the `getData` method with the parameter ‘A’ which is stored in `flyweight1` and invoked the `operation` method with parameter ‘1’. We invoked the method `getData` with the parameter ‘B’ which is stored in `flyweight2` and invoked `operation` method with parameter ‘2’. We invoked the method `getData` with the parameter ‘A’ again. now it will be not added because it is already present in the object and invoked the operation method with parameter ‘3’.
Output:
Intrinsic Property: A, Extrinsic Property: 1
Intrinsic Property: B, Extrinsic Property: 2
Intrinsic Property: A, Extrinsic Property: 3
Number of flyweights created: 2
Diagrammatic Representation:
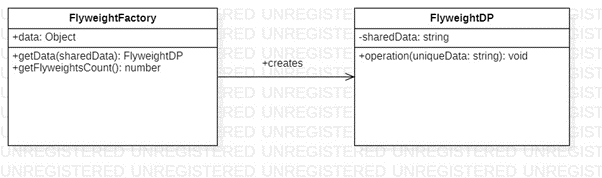
Flyweight Design Pattern
Advantages of Flyweight Design Pattern:
The Flyweight design pattern has serveral advantages including:
- Memory Efficiency: The Flyweight design pattern reduces the memory usage by allowing related objects to share the common state.
- Performance Improvement: The Flyweight design pattern improves the performance in terms of processing speed and initialization time.
- Resource utilization: The Flyweight design pattern allows efficient use of resources by minimizing the number of instances required to represent a similar object.
- Reduced Redundancy: The Flyweight design pattern helps to reduce the redundant code by extracting the common state.
Disadvantages of Flyweight Design Pattern:
The Flyweight design pattern has some disadvantages in terms of:
- Dependency on a Factory: The Flyweight design pattern often relies on a factory for creating flyweight objects, introducing a dependency on this factory.
- Increased Complexity: Implementing the Flyweight design pattern can introduce additional complexity to the codebase, especially if the logic for managing shared and unique states is intricate.
FAQ:
1. When do we use Flyweight design pattern?
A. In software development, we may rely on the Flyweight pattern when we need to manage a large number of similar objects efficiently, especially when the objects share common intrinsic (immutable) properties that can be shared among multiple instances.
2. Can you provide an example of a real-world use case for the Flyweight pattern?
A. In a text editor, characters in a document can be represented using the Flyweight pattern. Fonts, sizes, and styles are shared (intrinsic) properties, while character positions are unique (extrinsic) properties.
Share your thoughts in the comments
Please Login to comment...