Bootstrap Carousel
Last Updated :
08 Apr, 2024
Bootstrap Carousel enables slideshow functionality for images or content. Easily customizable with CSS and JavaScript. Supports responsive design and touch gestures, enhancing user experience in web development projects.
It can be included in your webpage using “bootstrap.js” or “bootstrap.min.js”. Carousels are not supported properly in Internet Explorer, this is because they use CSS3 transitions and animations to achieve the slide effect.
This is how we can create an image slideshow using the Bootstrap carousel.
Examples of Bootstrap Carousel
Example 1:Â In this example, we include a Bootstrap carousel with indicators, images, and navigation controls for the previous and next slides. It utilizes Bootstrap CSS and JavaScript libraries for styling and functionality.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Carousel</title>
<meta charset="utf-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1"
/>
<link
rel="stylesheet"
href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css"
/>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
</head>
<body>
<h1 class="text-success">
GeeksforGeeks
</h1>
<h4>Bootstrap Carousel</h4>
<div
id="myCarousel"
class="carousel slide"
data-ride="carousel"
>
<!-- Indicators -->
<ol class="carousel-indicators">
<li
data-target="#myCarousel"
data-slide-to="0"
class="active"
></li>
<li
data-target="#myCarousel"
data-slide-to="1"
></li>
<li
data-target="#myCarousel"
data-slide-to="2"
></li>
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner">
<div class="item active">
<img
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190603152813/ml_gaming.png"
/>
</div>
<div class="item">
<img
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190528184201/gateexam.png"
/>
</div>
<div class="item">
<img
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190507183137/Embedded-System-Programming-Languages.png"
/>
</div>
</div>
<!-- Left and right controls -->
<a
class="left carousel-control"
href="#myCarousel"
data-slide="prev"
>
<span
class="glyphicon glyphicon-chevron-left"
></span>
<span class="sr-only"
>Previous</span
>
</a>
<a
class="right carousel-control"
href="#myCarousel"
data-slide="next"
>
<span
class="glyphicon glyphicon-chevron-right"
></span>
<span class="sr-only">Next</span>
</a>
</div>
</body>
</html>
Output:
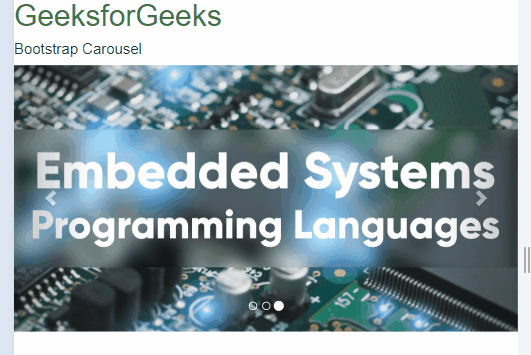
Bootstrap Carousel Example Output
Explanation
- Wrapper for slides: Div with class=”carousel-inner” contains slides, each specified within a div class=”item”.
- Outermost div: Div with class=”carousel” indicates the carousel, with data-ride=”carousel” for automatic sliding.
- Indicators: Ordered list with class=”carousel-indicators” provides dots indicating slides.
- Left and right controls: Buttons with data-slide attribute allow manual navigation.
- Adding captions: Use class=”carousel-caption” within each div class=”item”.
Example 2: In this example we features a Bootstrap carousel with two slides, each containing an image and a caption. Navigation controls allow users to move between slides. It uses Bootstrap for styling and functionality.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap Carousel</title>
<meta charset="utf-8" />
<meta
name="viewport"
content="width=device-width, initial-scale=1"
/>
<link
rel="stylesheet"
href=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/css/bootstrap.min.css"
/>
<script src=
"https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js">
</script>
<script src=
"https://maxcdn.bootstrapcdn.com/bootstrap/3.4.0/js/bootstrap.min.js">
</script>
</head>
<body>
<div
id="myCarousel"
class="carousel slide"
data-ride="carousel"
>
<!-- Indicators -->
<ol class="carousel-indicators">
<li
data-target="#myCarousel"
data-slide-to="0"
class="active"
></li>
<li
data-target="#myCarousel"
data-slide-to="1"
></li>
</ol>
<!-- Wrapper for slides -->
<div class="carousel-inner">
<div class="item active">
<img
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190603152813/ml_gaming.png"
/>
<div class="carousel-caption">
<b>
<h1
class="text-success"
>
GeeksforGeeks
</h1>
<p>
Join Geeks Classes
</p>
</b>
</div>
</div>
<div class="item">
<img
src=
"https://media.geeksforgeeks.org/wp-content/cdn-uploads/20190507183137/Embedded-System-Programming-Languages.png"
/>
<div class="carousel-caption">
<b>
<h1
class="text-primary"
>
GeeksforGeeks
</h1>
<p>
Join Geeks Classes
</p>
</b>
</div>
</div>
</div>
<!-- Left and right controls -->
<a
class="left carousel-control"
href="#myCarousel"
data-slide="prev"
>
<span
class="glyphicon glyphicon-chevron-left"
></span>
<span class="sr-only"
>Previous</span
>
</a>
<a
class="right carousel-control"
href="#myCarousel"
data-slide="next"
>
<span
class="glyphicon glyphicon-chevron-right"
></span>
<span class="sr-only">Next</span>
</a>
</div>
</body>
</html>
Output:
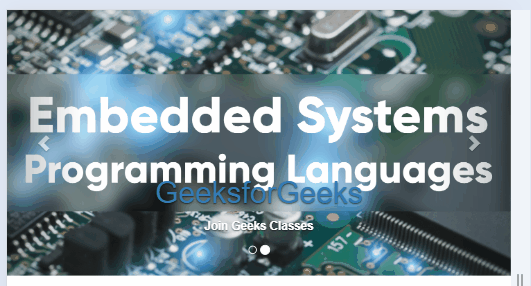
Bootstrap Carousel Example Output
- CYCLE: It cycles through the carousel from left to right.
Example:Â
Javascript
<script type="text/javascript">
$(document).ready(function(){
$(".start-slide").click(function(){
$("#myCarousel").carousel('cycle');
});
});
</script>
- PAUSE: Stops the carousel from moving wherever you want.
Example:Â
Javascript
<script type="text/javascript">
$(document).ready(function(){
$(".pause-slide").click(function(){
$("#myCarousel").carousel('pause');
});
});
</script>
- NUMBER: It cycles the carousel according to a particular frame(starting from 0, just like in an array).
Example:
Javascript
<script type="text/javascript">
$(document).ready(function(){
$(".slide-three").click(function(){
$("#myCarousel").carousel(3);
});
});
</script>
- PREV: Cycles the carousel to its previous image, it’s just like we did earlier in the bootstrap part.
Example:
Javascript
<script type="text/javascript">
$(document).ready(function(){
$(".prev-slide").click(function(){
$("#myCarousel").carousel('prev');
});
});
</script>
- NEXT: Cycles the carousel to its next image, it’s the same as we did in the bootstrap part of the carousel.
Example:Â
Javascript
<script type="text/javascript">
$(document).ready(function(){
$(".next-slide").click(function(){
$("#myCarousel").carousel('next');
});
});
</script>
Â
Supported Browsers:
Share your thoughts in the comments
Please Login to comment...