GPA Calculator using React
Last Updated :
22 Sep, 2023
GPA Calculator is an application that provides a user interface for calculating and displaying a student’s GPA(Grade Point Average). Using functional components and state management, this program enables users to input course information, including course name, credit hours and earned grades and adds them to a list dynamically. Users can also delete an individual list item from the course list. This application is implemented using Reactjs and provides a simple and responsive user interface to users.
Preview of Final Output:
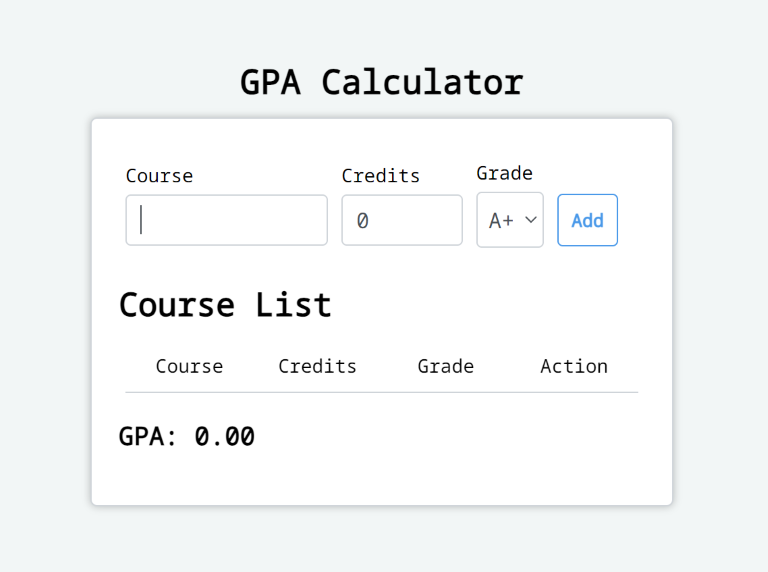
GPA Calculator using Reactjs Preview image
Prerequisites and Technologies:
Approach:
Utilizes ReactJS functional components and state managements to create an interactive web-based GPA Calculator. This application begins by capturing the input course details including course name, credits and earned grades and add them into a dynamic list which is visible to the user and user can also delete the individual entry for that list. The GPA is continuously updated and displayed on the interface with precision up to two decimal places.
Steps to create the application:
Step 1: Set up React project using the command
npx create-react-app <<name of project>>
Step 2: Navigate to the project folder using
cd <<Name_of_project>>
Step 3: Create a folder “components” and add four new files in it and name them as CourseForm.js, and CourseList.js, GPACalculator.js and GPACalculator.css
Project Structure:
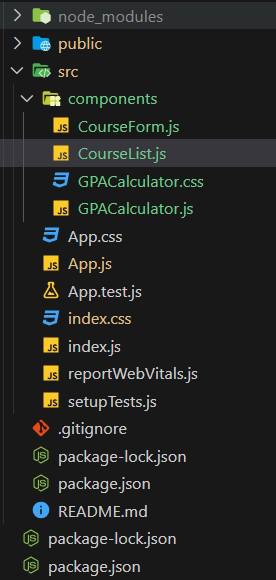
Project Structure
The updated dependencies in package.json will look like this:
{
"name": "GPACalculator",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.17.0",
"@testing-library/react": "^13.4.0",
"@testing-library/user-event": "^13.5.0",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4"
}
}
Example: Write the following code in respective files
- App.js: This file imports the GPACalculator components and exports it.
- GPACalculator.js: This file is the main component of a GPA calculator application built with React. It manages the state for course data and rendering of the user interface.
- CourseForm.js: This file defines a React component responsible for rendering and handling user input for adding new courses to the GPA calculator. It includes fields for course name, credit hours, and grade selection.
- CourseList.js: This file contains a React component responsible for displaying the list of added courses and calculating the GPA based on the entered grades and credit hours in the GPA calculator application.
- GPACalculator.css: This file contains the design of the GPACalculator elements.
Javascript
import './App.css' ;
import GPACalculator from './components/GPACalculator' ;
function App() {
return (
<div className= "App" >
<GPACalculator />
</div>
);
}
export default App;
|
Javascript
import React, { useState } from 'react' ;
import './GPACalculator.css' ;
import CourseForm from './CourseForm' ;
import CourseList from './CourseList' ;
const gradePoints = {
'A+' : 4.0,
'A' : 4.0,
'A-' : 3.7,
'B+' : 3.3,
'B' : 3.0,
'B-' : 2.7,
'C+' : 2.3,
'C' : 2.0,
'C-' : 1.7,
'D+' : 1.3,
'D' : 1.0,
'D-' : 0.7
};
const GPACalculator = () => {
const [courses, setCourses] = useState([]);
const handleAddCourse = (newCourse) => {
setCourses([...courses, newCourse]);
};
const handleDeleteCourse = (index) => {
const updatedCourses = courses.filter((course, i) => i !== index);
setCourses(updatedCourses);
};
const calculateGPA = () => {
let totalGradePoints = 0;
let totalCreditHours = 0;
courses.forEach((course) => {
totalGradePoints += gradePoints[course.grade] * course.creditHours;
totalCreditHours += course.creditHours;
});
return totalCreditHours === 0 ? 0 : totalGradePoints / totalCreditHours;
};
return (
<div className= 'container' >
<h1>GPA Calculator</h1>
<div className= "section" >
<CourseForm onAddCourse={handleAddCourse} />
<CourseList courses={courses} onDeleteCourse={handleDeleteCourse} calculateGPA={calculateGPA} />
</div>
</div>
);
};
export default GPACalculator;
|
Javascript
import React, { useState } from 'react' ;
const CourseForm = ({ onAddCourse }) => {
const [courseName, setCourseName] = useState( '' );
const [creditHours, setCreditHours] = useState(0);
const [grade, setGrade] = useState( 'A+' );
const handleAddCourse = () => {
if (courseName && creditHours > 0 && grade) {
const newCourse = {
courseName,
creditHours,
grade,
};
onAddCourse(newCourse);
setCourseName( '' );
setCreditHours(0);
setGrade( 'A+' );
} else {
alert( 'Please enter valid course details.' );
}
};
return (
<div className= "section1" >
<div>
<p>Course</p>
<input
type= "text"
value={courseName}
onChange={(e) => setCourseName(e.target.value)}
/>
</div>
<div>
<p>Credits</p>
<input
type= "number"
value={creditHours}
onChange={(e) => setCreditHours(Number(e.target.value))}
/>
</div>
<div>
<p>Grade</p>
<select value={grade} onChange={(e) => setGrade(e.target.value)}>
<option value= "A+" >A+</option>
<option value= "A" >A</option>
<option value= "A-" >A-</option>
<option value= "B+" >B+</option>
<option value= "B" >B</option>
<option value= "B-" >B-</option>
<option value= "C+" >C+</option>
<option value= "C" >C</option>
<option value= "C-" >C-</option>
<option value= "D+" >D+</option>
<option value= "D" >D</option>
<option value= "D-" >D-</option>
</select>
</div>
<div>
<p style={{ opacity: 0 }}>-</p>
<button onClick={handleAddCourse}>Add</button>
</div>
</div>
);
};
export default CourseForm;
|
Javascript
import React from 'react' ;
const CourseList = ({ courses, onDeleteCourse, calculateGPA }) => {
return (
<div className= "section2" >
<div>
<h2>Course List</h2>
<ul style={{ borderBottom: '1px solid #ced4da' , paddingBottom: '10px' }}>
<li>Course</li>
<li>Credits</li>
<li>Grade</li>
<li>Action</li>
</ul>
{courses.map((course, index) => (
<ul key={index}>
<li>{course.courseName}</li>
<li>{course.creditHours}</li>
<li>{course.grade}</li>
<li><button onClick={() => onDeleteCourse(index)}>Delete</button></li>
</ul>
))}
</div>
<div>
<h3>GPA: {calculateGPA().toFixed(2)}</h3>
</div>
</div>
);
};
export default CourseList;
|
CSS
*{
box-sizing: border-box;
font-family : 'Noto Sans Mono' , monospace ;
}
body{
padding : 0 ;
margin : 0 ;
min-height : 100 vh;
display : flex;
justify- content : center ;
align-items: center ;
background-color : #f1f6f6 ;
}
.container {
max-width : 650px ;
margin : 5px ;
width : calc( 100% - 10px );
}
.container h 1 {
margin : 0 ;
margin-bottom : 10px ;
text-align : center ;
font-size : 25px ;
}
.section{
border : 1px solid #ced4da ;
border-radius: 5px ;
padding : 20px ;
border : 1px solid #ced4da ;
background : #fff ;
box-shadow: 0 0 6px rgba( 0 , 0 , 0 , 0.25 );
}
.section 1 {
display : flex;
justify- content : space-between;
align-items: center ;
width : 100% ;
}
.section 1 div{
margin : 5px ;
}
.section 1 div:first-child input{
max-width : 150px ;
text-align : left ;
}
.section 1 select{
width : 100% ;
font-size : 1 rem;
padding : 8px 4px ;
font-weight : 400 ;
line-height : 1.5 ;
color : #495057 ;
outline : none ;
background-color : #fff ;
background- clip : padding-box;
border : 1px solid #ced4da ;
border-radius: 0.25 rem;
}
.section 1 div:nth-child( 2 ) input{
max-width : 90px ;
text-align : left ;
}
.section 1 div:nth-child( 3 ){
width : 50px ;
text-align : left ;
}
.section 1 div:nth-child( 4 ){
width : 60px ;
text-align : left ;
}
.section 1 p{
margin : 5px 5px 5px 0px ;
text-align : left ;
font-size : 14px ;
}
input{
width : 100% ;
font-size : 1 rem;
padding : 6px 10px ;
font-weight : 400 ;
line-height : 1.5 ;
color : #495057 ;
outline : none ;
background-color : #fff ;
background- clip : padding-box;
border : 1px solid #ced4da ;
border-radius: 0.25 rem;
}
.section button{
padding : 9.5px ;
outline : none ;
background-color : #fff ;
color : #1d9bf0 ;
border : 1px solid #1d9bf0 ;
border-radius: 4px ;
cursor : pointer ;
font-weight : bold ;
transition: 0.5 s all ;
}
.section button:hover{
color : white ;
background-color : #1d9bf0 ;
border-color : #1d9bf0 ;
border-width : 1px ;
}
.section 2 ul{
font-size : 14px ;
list-style-type : none ;
padding-inline-start: 0px ;
display : grid;
align-items: center ;
margin : 5px ;
grid-template-columns: 1 fr 1 fr 1 fr 1 fr;
text-align : center ;
}
|
Steps to run the application:
Step 1: Type the following command in terminal.
npm start
Step 2: Open web-browser and type the following URL
http://localhost:3000/
Output:
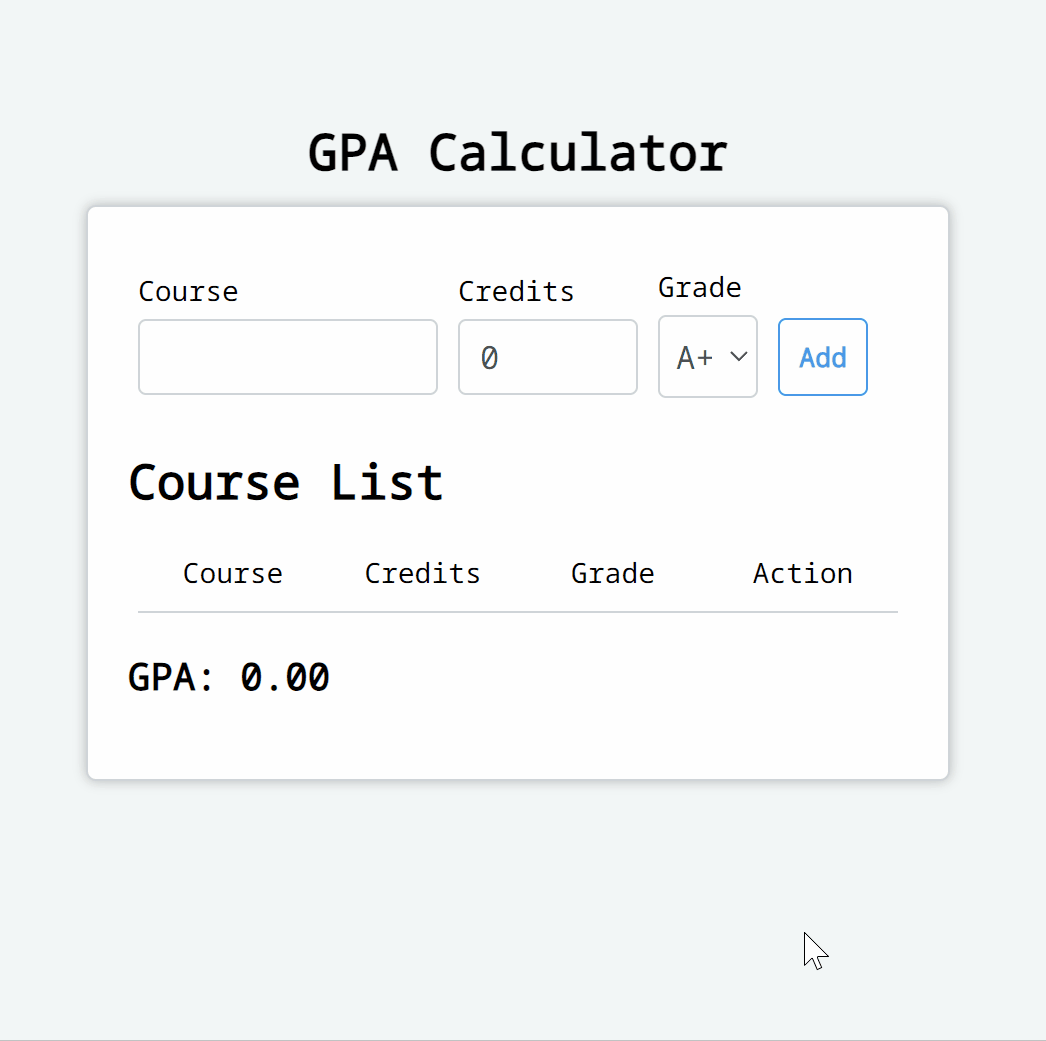
GPA Calculator using Reactjs GIF
Share your thoughts in the comments
Please Login to comment...