Bloom Filters in Java Using Redis
Last Updated :
06 Mar, 2024
Bloom filters are the in-memory data structures used in applications to test whether a key belongs to a particular set or not, it’s like a Redis cache but where the Redis cache stores the entire data as it is rather than any optimization, but in Bloom filters the bitset or bitmap implementation where it provides a good memory optimization and find operation is very fast here each bit represents the presence of a particular element where each bit is hashed by different hash functions.
Bloom filters are much faster and more efficient for some use cases like membership testing i.e. particular elements belong to a set or not in the memory, compared to normal Redis caching.
Steps for Starting the Redis Stack to Start the Redis Bloom Server Using Docker
- Install the Docker from the official website.
- When the server starts the code will start working
Step 1: Adding the dependency on pom.xml after starting the Redis bloom server:
<dependency>
<groupId>com.redislabs</groupId>
<artifactId>jrebloom</artifactId>
<version>2.1.0</version>
</dependency>
Step 2: Initializing Bloom Filter Client in Java
Client clientVariable = new Client(hostAddress, port, timeOut , poolSize );
The parameters that can be given while initializing:
- host address: The server IP where the application is deployed.
- port: The port it is pointing to default is 6379.
- timeOut: The maximum time it will wait to get the response from the server.
- poolSize: The pool size of the Jedis pool.
Basic operations performed on Redis Bloom
Operations
|
Syntax
|
Parameters
|
Creating a Redis Bloom filter
|
clientVariable.createFilter( name, initCapacity, errorRate)
|
- name: The name of the Bloom Filter we want to Keep
- initCapacity: The size of the Bloom Filter that we want to keep
- errorRate : It is the expected rate or probability at which it gives incorrect result for the existence of the element in the set
|
Checking the info the Bloom filter
|
clientVariable.info(filterName)
|
- filterName: The name of the Bloom Filter
|
Adding the keys into the Filter
|
clientVarible.add(filterName, key)
|
- filterName: The name of the Bloom Filter
- key : The element that we want to add into that particular filter
|
Checking if it exists in the filter
|
clientVarible.exists(filterName, key)
|
- filterName: The name of the Bloom Filter
- key : The element that we want to check if exists in that particular filter
|
Deleting the bloomFilter
|
clientVariable.delete(filterName)
|
- filterName: The name of the Bloom Filter
|
Program of Bloom Filters in Java using Redis
Below is the implementation for Creating and Checking info Redis Bloom filter:
Example 1:
Java
package org.geeksforgeeks;
import io.rebloom.client.Client;
import java.util.Map;
public class Main {
public static void main(String args[])
{
Client bloomFilerClient = new Client( "localhost" , 6379 , 2000 , 20 );
bloomFilerClient.createFilter( "premium_users" , 200000000 , 0.01 );
bloomFilerClient.createFilter( "non_premium_users" , 500000000 , 0.01 );
Map<String, Object> mp_premium = bloomFilerClient.info( "premium_users" );
Map<String, Object> mp_non_premium = bloomFilerClient.info( "non_premium_users" );
System.out.println( "Premium Users Filter Info : " + mp_premium.toString());
System.out.println( "Non Premium Users Filter Info : " + mp_non_premium.toString());
}
}
|
Output:
Below in the output we can see the Redis Bloom Filter info has created.

Example 2:
Below is the implementation for Adding and Checking keys into the Filter:
Java
package org.geeksforgeeks;
import io.rebloom.client.Client;
public class Main {
public static void main(String[] args) {
Client bloomFilerClient = new Client( "localhost" , 6379 , 2000 , 20 );
bloomFilerClient.add( "premium_users" , "gfg1@gmail.com" );
bloomFilerClient.add( "non_premium_users" , "gfg2@gmail.com" );
if (bloomFilerClient.exists( "premium_users" , "gfg1@gmail.com" )) {
System.out.println( "gfg1@gmail.com is a premium user" );
}
if (bloomFilerClient.exists( "non_premium_users" , "gfg2@gmail.com" )) {
System.out.println( "gfg2@gmail.com is not a premium user" );
}
}
}
|
Output :
Below in the output we can see the keys added into the filter.
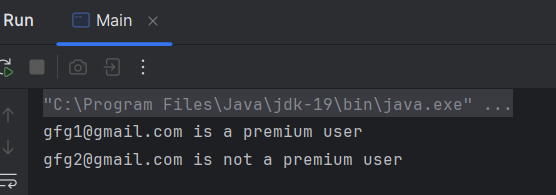
Example 3:
Below is the implementation of Deleting the bloomFilter:
Java
package org.geeksforgeeks;
import io.rebloom.client.Client;
public class Main {
public static void main(String[] args) {
Client bloomFilerClient = new Client( "localhost" , 6379 , 2000 , 20 );
bloomFilerClient.delete( "premium_users" );
if (!bloomFilerClient.exists( "premium_users" , "gfg1@gmail.com" )) {
System.out.println( "gfg1@gmail.com is no longer a premium user" );
}
}
}
|
Output :
Below in the output we can see the bloom filter has deleted.
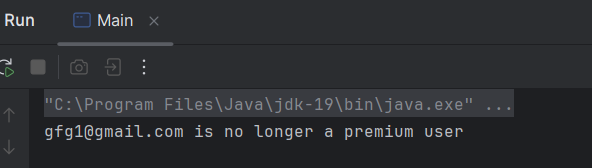
Limitations of Bloom Filter:
- They may produce false positives and false negatives very rarely.
- Limited operations can only be performed insertion and exists (membership check whether a particular key exists or not in a particular filter).
- The size of the bloom filter should be pre estimated.
- It cannot store exact data and also, we can’t retrieve.
- It doesn’t have the feature of single key deletion in a bloomFilter.
Share your thoughts in the comments
Please Login to comment...