Complete Guide on Redis Strings
Last Updated :
28 Sep, 2023
Redis String is a sequence of bytes that can store a sequence of bytes, including text, Object, and binary arrays. which can store a maximum of 512 megabytes in one string. Redis String can also be used like a Redis Key for mapping a string to another string. String Data Types are useful in different types of use cases like caching HTML fragments or different pages.
Important Topics on Redis Strings
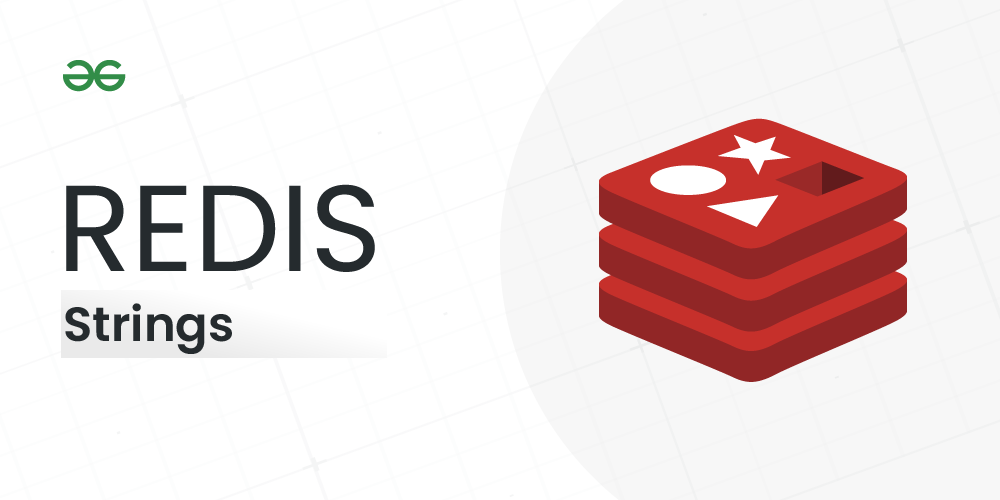
SET and GET Command
- SET command is used to set the string with key = name and value = “GeeksForGeeks”.
- GET command will print the value associated with the key name, which is GeeksForGeeks.
Syntax:
SET key = “value”
GET value
Example:
SET name = “Aditya”
GET name
Output:
“Aditya”
Time Complexity of SET and GET Command: O(1)
Basic Command Used in String Data Structure in Redis:
1. SET
This command will store a string value.
Syntax:
SET Key Value
Example:
SET User:1 GFG
Time Complexity: O(1)
2. SETNX
This command will stores a string value only if the key doesn’t already exist. Useful for implementing locks.
Syntax:
SETNX key value
Example:
SETNX key “Hello”
Time Complexity: O(1)
3. GET
This command will retrieves a string value.
Syntax:
GET key
Example:
GET user:123:name
Time Complexity: O(1)
4. MSET
This command will stores multiple values in just a command.
Syntax:
MSET key value [key value …]
Example:
MSET key1 “Hello” key2 “World”
Time Complexity: O(N)
5. MGET
This command retrieves multiple string values in a single operation.
Syntax:
MGET key [key …]
Example:
MGET key1 key2
Time complexity: O(N)
6. INCR
This command will parse a string to integer, increment by one.
Syntax:
INCR key
Example:
INCR mykey
Time complexity: O(1)
7. APPEND
This command will use to append a value to an existing string.
Syntax:
APPEND key value
Example:
APPEND mykey “Hello”
Time complexity: O(1)
8. GETRANGE
This command will use to print the substring of a string.
Syntax:
GETRANGE key start end
Example:
GETRANGE mykey 0 3
Time complexity: O(N)
9. GETSET
This command will sets the string value of a string and returns its old value.
Syntax:
GETSET key value
Example:
GETSET mycounter “0”
Time complexity: O(1)
10. GETBIT
This command will set the string value of a key and return its old value.
Syntax:
GETBIT key offset
Example:
GETBIT mykey 0
Time complexity: O(1)
11. STRLEN
This command will return the length of the string.
Syntax:
STRLEN key
Example:
STRLEN mykey
Time complexity: O(1)
12. DECR
This command will parse a string to integer, decrease by one.
Syntax:
DECR key
Example:
DECR mykey
Time complexity: O(1)
13. INCRBY
This command will Increments the integer value of a key by the given amount.
Syntax:
INCRBY key increment
Example:
INCRBY mykey 5
Time complexity: O(1)
14. INCRBYFLOAT
This command will Increments the float value of a key by the given amount.
Syntax:
INCRBYFLOAT key increment
Example:
INCRBYFLOAT mykey 0.1
Time complexity: O(1)
15. DECRBY
This command will Decrements the integer value of a key by the given number
Syntax:
DECRBY key decrement
Example:
DECRBY mykey 3
Time complexity: O(1)
16. MSETNX
This command will set multiple keys to multiple values, only if none of the keys exist.
Syntax:
MSETNX key value [key value …]
Example:
MSETNX key1 “Hello” key2 “there”
Time complexity: O(N)
17. SETEX
This command will sets the value with the expiry of a key.
Syntax:
SETEX key seconds value
Example:
SETEX mykey 10 “Hello”
Time complexity: O(1)
18. SETNX
This command will set the value of a key, only if the key does not exist.
Syntax:
SETNX key value
Example:
SETNX mykey “World”
Time complexity: O(1)
19. SETRANGE
This command will Overwrites the part of a string at the key starting at the specified offset.
Syntax:
SETRANGE key offset value
Example:
SETRANGE key1 6 “Redis”
Time complexity: O(1)
20. PSETEX
This command will sets the value and expiration in milliseconds of a key.
Syntax:
PSETEX key milliseconds value
Example:
PSETEX mykey 1000 “Hello”
Time complexity: O(1)
Redis strings typically have a size limit of 512 MB.
Example of Redis String:
Example1:
MSET user: 1 “GFG” user: 2 “Noida” user: 3 “UP”
MGET user:1 user: 2 user: 3
Output: GFG
Noida
UP
Example 2:
SET user:1 “GFG”
APPEND user: 1 “isTheBest”
GET user: 1
Output: GFGisTheBest
Redis Strings as Counters
We can use Redis strings as counters, we can initialize a Redis variable, increment the value, and decrement the value by using INCR and DECR commands. we can use counters in order to achieve the counter for tracking the number of website visitors. we can also use INCRBY command to increase the integer by a specific value.
Example:
SET total_users 0
INCR total_users ( 0+1 =1 )
GET total_users
Output: 1
INCR total_users (1+1 =2)
DECR total_users ( 2 – 1 = 1)
GET total_users
Output: 1
INCRBY total_users 20
GET total_users
Output: 21
How to manage counters?
We can mange the Redis counters using different commands and by using those commands you are able to increment and decrement numeric values.
By using INCR and DECR commands:
Redis provides two simple commands, INCR and DECR, to increment and decrement a numeric value stored in a key.
Example:
SET user:id dozen 12
INCR dozen
GET dozen
Output: 13
By using SET command using NX:
This command is used for initialization of the strings basically.
By using INCRBYFLOAT:
This command is used to increment the floating values.
SET counter= 3.6
INCRBYFLOAT counter 2.5
GET counter
Output: 6.1
Most of the Operations Performed in the strings can be done in O(1) time. But, SUBSTR, GETRANGE, SETRANGE commands can be performed in O(N) time.
Alternatives of String in Redis
There are different alternatives of string in Redis:
- List: Lists in Redis are ordered collections of strings. They support operations like appending to the end, pushing to the front, popping elements, and more.
- Sets: Sets in Redis are collections of unique strings. They are used for membership testing and managing unique items.
- Hashes: Hashes in Redis are like dictionaries or maps, where strings can be associated with a value.
Redis strings are a fundamental building block in Redis and are widely used for caching, session management, and storing various forms of data that need to be accessed quickly.
Share your thoughts in the comments
Please Login to comment...