Redis cache in Java using Jedis
Last Updated :
11 Jan, 2024
Jedis is the client library for Java in Redis that provides all the Redis features in applications based on Java. It is thread-safe and supports all the data types that Redis supports. It also provides the Jedis pooling which helps in creating a 3pool of connections that can be reused to enhance the performance.
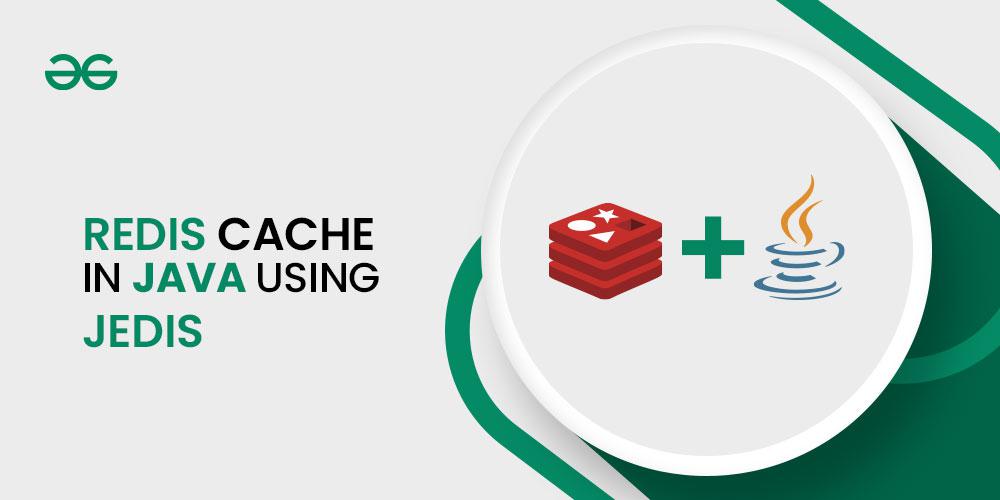
Redis cache is a popularly used in-memory cache that acts like a data structure server that is used by many applications in use cases like caching, real-time analytics, and session management to make high-performance data access and storage. It also persist data periodically to the disk but RAM is the primary access.
Download Redis based on the operating system and start the server:
In Windows, it gets started by running redis-server.exe, and in Linux/OS run the command redis-server to start the Redis server.
Importing Import Jedis into application by adding Maven Dependency in pom.xml:
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>4.4.4</version>
</dependency>
Initializing Jedis:
Jedis jedis = new Jedis(hostname, port)
Parameters:
hostname: The server address where the instance of Redis is running, by default localhost.
portname: The port number where the server of Redis is listening, by default 6379.
1. set() Method:
Creating a key and setting a value.
jedis.set(key,value)
Parameters: Key and Value can be given in bytes or string format.
Code Snippet :
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
jedis.set("key", "value");
}
}
|
2. get() method:
Reading a value of a key,
jedis.get(key)
Parameters: The key can be in value or bytes.
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
jedis.set("key", "value");
System.out.println("The value of the key is : " + jedis.get("key"));
}
}
|
Output :
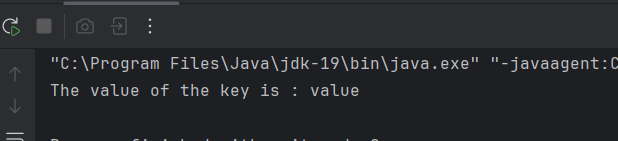
3. exists() method:
Checks the existence of a key.
jedis.exists(key)
Parameters: The key can be in value or bytes.
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
jedis.set("key", "value");
if (jedis.exists("key")) {
System.out.println("The key exists with value : " + jedis.get("key"));
}
else {
System.out.println("The key does not exists");
}
}
}
|
Output :

4. del() method :
Deletes the key from the cache.
jedis.del(key)
Parameters: The key can be in value or bytes, also we can pass of keys to be deleted.
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
jedis.set("key", "value");
jedis.del("key");
if (!jedis.exists("key")) {
System.out.println("The key is deleted");
}
}
}
|
Output:

5. Setting Ttl for the keys:
Ttl means Time to live, in the cache we can configure until how much time the key should be there in the cache and after the ttl ends the key automatically gets deleted from the cache memory.
Setting a key with Ttl :
jedis.setex(key,timeinsec,value)
Parameters: The key and value can be in bytes.
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
jedis.setex("key", 60 , "value");
}
}
|
Checking the key after 60 seconds:
Java
package org.geeksforgeeks;
import redis.clients.jedis.Jedis;
public class Main {
public static void main(String[] args) {
Jedis jedis = new Jedis();
if (!jedis.exists("key")) {
System.out.println("The key is deleted from cache after 60 seconds");
}
}
}
|
Output :
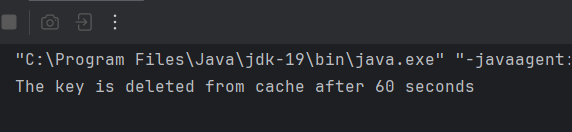
Share your thoughts in the comments
Please Login to comment...