How to Identify & Solve Binary Search Problems?
Last Updated :
18 Jan, 2024
We all know that Binary search is the most efficient search algorithm as of now, and it has multiple applications in the programming domain for the same reason. But very often the problems do not have any information about applying Binary Search or even any hint on using a Searching algorithm altogether. So it becomes very important to have an understanding of how to identify Binary Search Problems, how to solve Binary Search problems and what are the most common interview questions that have a Binary Search solution involved. In this post, we have curated the topics to do just that.
Binary search is a searching technique to search an ordered list of data based on the Divide and Conquer technique which repeatedly divides the search space by half in every iteration.
Possible types of problems and in which type Binary Search can be applied or not:
1) When Input is sorted:
In this case, Identification as a binary search problem is very straightforward as we can easily deduce which half needs to be removed from the search space.
2)When Input is unsorted but the problem follows a monotonic nature:
In this case also binary search can be applied as the problem follows a monotonic nature which means the function can be either increasing or decreasing with an increase in a parameter so that one-half can be removed from search space.
3)When the Expected answer is ordered:
Whenever we can identify that the answer to the problem lies between in a range L to R and there is a Monotonic Behaviour of the answer in range L to R then we can think to apply binary search on the answer.
4)When Neither input is sorted nor the problem follows monotonic behavior:
In this case we can not apply binary search.
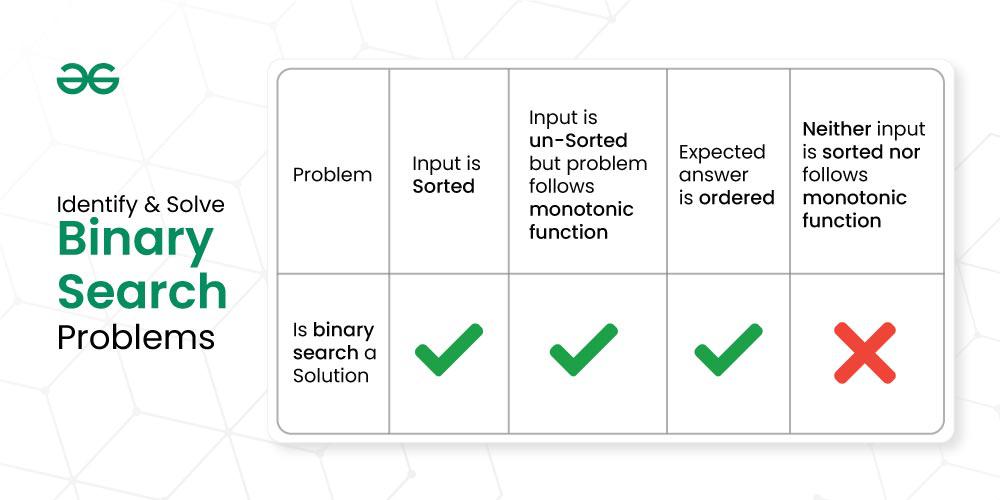
Identify and Solve Binary Search Problems
Now here is the general technique to Identify and Solve the Binary search problems.
How to Identify Binary Search Problems?
Based on the number of problems involving Binary Search, we can safely assume the scenario when Binary search is involved in a problem, as mentioned below:
Binary search can only be applied to a problem if and only if the Problem is monotonic in nature.
When a Problem is considered to be Monotonic?
Suppose we are given a problem f() and the expected solution to this problem is y, then we can define the problem as
y = f(n)
Now if this problem show a behaviour such that it either only increases or only decreases with the change in parameter. Then it is said that the problem shows Monotonic (only single direction) behaviour.
In other words, A function f(n1) is said to be monotonic if and only if:
- for any n1, if f(n1) returns true, then for any value of n2 (where n2 > n1), f(n2) should also return true,
- and similarly, if for a certain value of n1 for which f(n1) is false, then for any value n2 (n2 < n1) the function f(n2) should also return false.
The same is shown in the graph below:
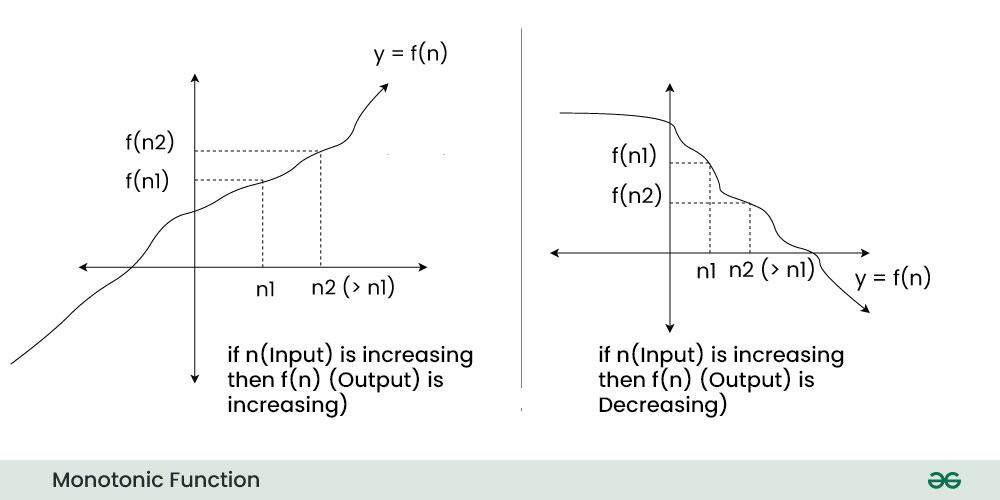
Monotonic Functions
Now if this problem shows a monotonic behavior, generally this tells you that you can apply a binary search here.
How to Solve Binary Search Problems?
- Define a search space by pointers let’s say low to high.
- Calculate mid value of search space,and check mid value is true or false.
- Considering above resut figure out where expected answer should lie, In the left half or right half Then update the search space accordingly.
- Repeat the above steps till there is search space left to search.
Example to show How to Identify & Solve a Problem using Binary search:
Problem Statement : Given an array arr[] of integers and a number x, the task is to find the minimum length of subarray with a sum greater than the given value k.
Example:
Input: arr[] = {1, 4, 45, 6, 0, 19}, x = 51
Output: 3
Explanation:Minimum length subarray is {4, 45, 6}
Identification as a Binary Search Problem:
Let n1 is size of subarray and f(n1) is true i.e. there is a subarray of size n1 whose sum is greater than x.
Take any n2 (n2>n1) i.e at least one more element than previous subarray size then it is guaranteed that f(n2) must hold true because there is subarray of size n1 has sum greater than x, adding at least one non-negative integer increases the sum.
Problem is monotonic in nature and can be solved using binary search.
Solution of the Problem using Binary search:
- Define search space
- low = 0,as minimum size of subarray can be zero.
- high = length of the array, as subarray size can be up to length of array.
- Calculate mid value as (low+high)/2 and check f(mid) returns true or false.
- Check sum of every subarray of size mid is greater than x or not using sliding window.
- if there is a subarray present then f(mid) is true.
- else f(mid) is false.
- Update the search space:
- if f(mid) is true and minimal length to be find then expected answer should lie in left half (high = mid-1) as in the right half of mid all the value are true, store the mid value as best possible answer till now.
- if f(mid) is false i.e. there is no subarray of size mid,so size need to be increased to increase the sum.Means expected answer should lie in the right half(low =mid+1).
- Repeat the above steps with updated search space till there is search space to search.
Implementation of the above solution:
C++
#include <bits/stdc++.h>;
using namespace std;
bool f( int mid, int arr[], int n, int x)
{
bool issubarray = false ;
int i = 0, j = 0, sum = 0;
for (j = 0; j < mid; j++) {
sum += arr[j];
}
while (j < n) {
if (sum > x) {
issubarray = true ;
}
sum -= arr[i];
sum += arr[j];
i++;
j++;
}
if (sum > x) {
issubarray = true ;
}
return issubarray;
}
int smallestSubWithSum( int arr[], int n, int x)
{
int low = 0, high = n, ans = INT_MAX;
while (low <= high) {
int mid = (low + high) / 2;
if (f(mid, arr, n, x)) {
ans = mid;
high = mid - 1;
}
else {
low = mid + 1;
}
}
return (ans == INT_MAX) ? 0 : ans;
}
int main()
{
int arr[] = { 1, 4, 45, 6, 0, 19 };
int n = 6;
int x = 51;
cout << smallestSubWithSum(arr, n, x);
return 0;
}
|
Java
public class SmallestSubarray {
static boolean isSubarrayPresent( int mid, int [] arr, int n, int x) {
boolean isSubarray = false ;
int i = 0 , j = 0 , sum = 0 ;
for (j = 0 ; j < mid; j++) {
sum += arr[j];
}
while (j < n) {
if (sum > x) {
isSubarray = true ;
}
sum -= arr[i];
sum += arr[j];
i++;
j++;
}
if (sum > x) {
isSubarray = true ;
}
return isSubarray;
}
static int smallestSubarrayWithSum( int [] arr, int n, int x) {
int low = 0 , high = n, ans = Integer.MAX_VALUE;
while (low <= high) {
int mid = (low + high) / 2 ;
if (isSubarrayPresent(mid, arr, n, x)) {
ans = mid;
high = mid - 1 ;
} else {
low = mid + 1 ;
}
}
return (ans == Integer.MAX_VALUE) ? 0 : ans;
}
public static void main(String[] args) {
int [] arr = { 1 , 4 , 45 , 6 , 0 , 19 };
int n = 6 ;
int x = 51 ;
System.out.println(smallestSubarrayWithSum(arr, n, x));
}
}
|
Python3
def f(mid, arr, n, x):
is_subarray = False
i, j, summation = 0 , 0 , 0
for j in range (mid):
summation + = arr[j]
while j < n:
if summation > x:
is_subarray = True
summation - = arr[i]
summation + = arr[j]
i + = 1
j + = 1
if summation > x:
is_subarray = True
return is_subarray
def smallestSubWithSum(arr, n, x):
low, high, ans = 0 , n, float ( 'inf' )
while low < = high:
mid = (low + high) / / 2
if f(mid, arr, n, x):
ans = mid
high = mid - 1
else :
low = mid + 1
return ans if ans ! = float ( 'inf' ) else 0
arr = [ 1 , 4 , 45 , 6 , 0 , 19 ]
n = 6
x = 51
print (smallestSubWithSum(arr, n, x))
|
C#
using System;
public class SmallestSubarray
{
static bool IsSubarrayPresent( int mid, int [] arr, int n, int x)
{
bool isSubarray = false ;
int i = 0, j = 0, sum = 0;
for (j = 0; j < mid; j++)
{
sum += arr[j];
}
while (j < n)
{
if (sum > x)
{
isSubarray = true ;
}
sum -= arr[i];
sum += arr[j];
i++;
j++;
}
if (sum > x)
{
isSubarray = true ;
}
return isSubarray;
}
static int SmallestSubarrayWithSum( int [] arr, int n, int x)
{
int low = 0, high = n, ans = int .MaxValue;
while (low <= high)
{
int mid = (low + high) / 2;
if (IsSubarrayPresent(mid, arr, n, x))
{
ans = mid;
high = mid - 1;
}
else
{
low = mid + 1;
}
}
return (ans == int .MaxValue) ? 0 : ans;
}
public static void Main( string [] args)
{
int [] arr = { 1, 4, 45, 6, 0, 19 };
int n = 6;
int x = 51;
Console.WriteLine(SmallestSubarrayWithSum(arr, n, x));
}
}
|
Javascript
function f(mid, arr, n, x) {
let issubarray = false ;
let i = 0, j = 0, sum = 0;
for (j = 0; j < mid; j++) {
sum += arr[j];
}
while (j < n) {
if (sum > x) {
issubarray = true ;
}
sum -= arr[i];
sum += arr[j];
i++;
j++;
}
if (sum > x) {
issubarray = true ;
}
return issubarray;
}
function smallestSubWithSum(arr, n, x) {
let low = 0, high = n, ans = Number.MAX_SAFE_INTEGER;
while (low <= high) {
let mid = Math.floor((low + high) / 2);
if (f(mid, arr, n, x)) {
ans = mid;
high = mid - 1;
} else {
low = mid + 1;
}
}
return (ans === Number.MAX_SAFE_INTEGER) ? 0 : ans;
}
let arr = [1, 4, 45, 6, 0, 19];
let n = 6;
let x = 51;
console.log(smallestSubWithSum(arr, n, x));
|
Time Complexity:O(NlogN),N is the size of the subarray
Auxiliary space: O(1)
Most Common Interview Problems on Binary Search
Share your thoughts in the comments
Please Login to comment...