Axes customization in R
Last Updated :
16 Apr, 2024
Data visualization is crucial for understanding data and sharing insights. In R Programming Language we can easily create visualizations using tools like ggplot2, lattice, and base R plotting functions. These tools offer many ways to customize the plots. However, focusing on customizing axes can really improve how clear and impactful our visualizations are. This level of customization helps ensure our visualizations are essential for exploring and sharing insights in R.
What is Axes?
Axes, in the context of data visualization, are the horizontal and vertical lines that define the boundaries of a plot. They represent the scales along which data points are plotted, providing essential information about the range and context of the data being presented. The horizontal axis (x-axis) typically represents the independent variable, while the vertical axis (y-axis) usually represents the dependent variable. Axes play a crucial role in interpreting and understanding the data visualized in plots and charts.
What is Customize Axes?
Customizing axes involves tailoring the appearance and labeling of these scales to better suit the data and enhance interpretability. Whether adjusting the range, formatting labels, or modifying tick marks, understanding axis customization in R gives user to create visualizations that effectively reflect their messages.
Setting Axis Limits
R
library(ggplot2)
# Example Data
data <- data.frame(
x = 1:10,
y = 1:10^2
)
# Basic Plot
basic_plot <- ggplot(data, aes(x, y)) +
geom_point() +
labs(title = "Basic Scatter Plot") +
theme_minimal()
# Customized Plot with Adjusted Axis Limits
customized_plot <- basic_plot +
xlim(3, 7) + # Adjusting x-axis limits
ylim(20, 80) + # Adjusting y-axis limits
labs(title = "Customized Scatter Plot with Adjusted Axis Limits")
# Display the Plot
print(customized_plot)
Output:
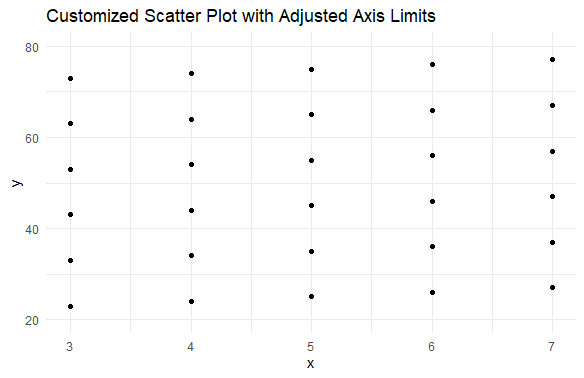
Axes customization in R
By setting custom axis limits, we focus on a specific region of interest within the data.
- We restrict the x-axis to the range 3 to 7 and the y-axis to the range 20 to 80, effectively zooming in on a subset of the data.
Modifying Axis Labels
R
library(ggplot2)
# Example Data
data <- data.frame(
x = 1:10,
y = 1:10^2
)
# Basic Plot
basic_plot <- ggplot(data, aes(x, y)) +
geom_point() +
labs(title = "Basic Scatter Plot") +
theme_minimal()
# Customized Plot with Modified Axis Labels
customized_plot <- basic_plot +
labs(x = "Custom X Label", y = "Custom Y Label",
title = "Customized Scatter Plot with Modified Axis Labels")
# Display the Plot
print(customized_plot)
Output:
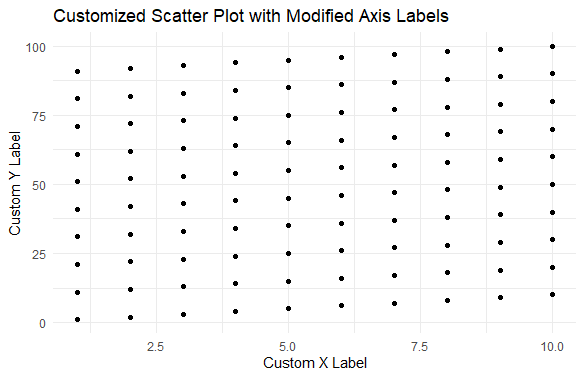
Axes customization in R
Modifying axis labels allows us to provide more descriptive information about the plotted variables.
- We change the x-axis label to “Custom X Label” and the y-axis label to “Custom Y Label” for better clarity and understanding of the data.
Adjusting Tick Marks
R
library(ggplot2)
# Example Data
data <- data.frame(
x = 1:10,
y = c(5, 8, 12, 15, 18, 22, 25, 28, 32, 35)
)
# Basic Plot
basic_plot <- ggplot(data, aes(x, y)) +
geom_point() +
labs(title = "Basic Scatter Plot") +
theme_minimal()
# Customized Plot with Adjusted Tick Marks
customized_plot <- basic_plot +
scale_x_continuous(breaks = seq(2, 10, by = 2)) + # Customizing x-axis tick marks
scale_y_continuous(breaks = seq(5, 35, by = 5)) + # Customizing y-axis tick marks
labs(title = "Customized Scatter Plot with Adjusted Tick Marks")
# Display the Plot
print(customized_plot)
Output:
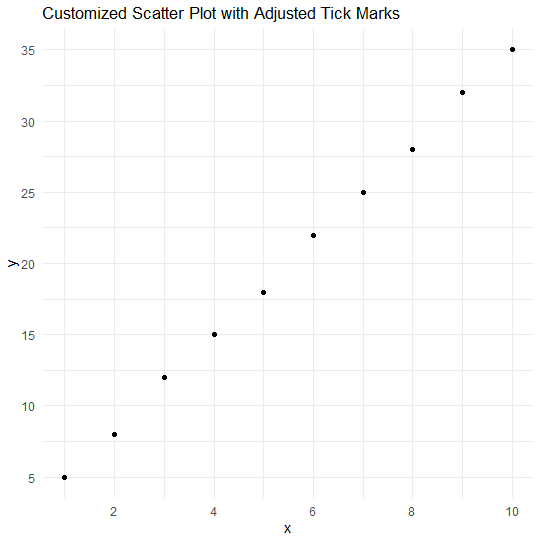
Customized Scatter Plot with Adjusted Tick Marks
Adjusting tick marks allows us to control the placement and frequency of ticks along the axes.
- Here, we specify custom breaks for both the x-axis and y-axis to better align with the data distribution.
Formatting Axis Text
R
library(ggplot2)
# Example Data
data <- data.frame(
x = 1:10,
y = c(5, 8, 12, 15, 17, 22, 25, 27, 32, 35)
)
# Basic Plot
basic_plot <- ggplot(data, aes(x, y)) +
geom_point() +
labs(title = "Basic Scatter Plot") +
theme_minimal()
# Customized Plot with Formatted Axis Text
customized_plot <- basic_plot +
theme(axis.text.x = element_text(angle = 45, hjust = 1, size = 10, color = "blue"),
axis.text.y = element_text(size = 10, color = "green")) +
labs(title = "Customized Scatter Plot with Formatted Axis Text")
# Display the Plot
print(customized_plot)
Output:
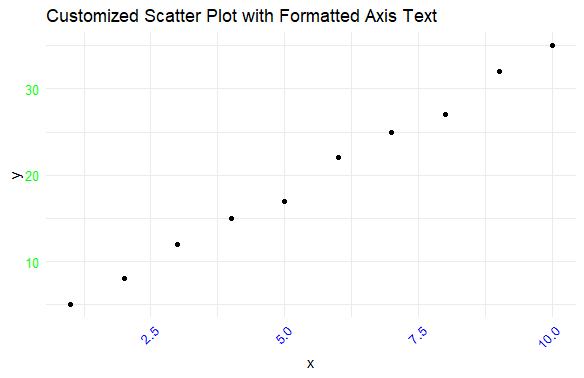
Axes customization in R
Specifically, it rotates the x-axis text by 45 degrees (angle = 45) and aligns it to the right (hjust = 1).
- It also sets the size of the x-axis text to 10 and changes its color to blue (size = 10, color = “blue”).
- Additionally, it adjusts the size of the y-axis text to 10 and changes its color to green (size = 10, color = “green”).
- The customized plot is then displayed.
Adding Secondary Axes
R
library(ggplot2)
# Example Data
data <- data.frame(
x = 1:10,
y1 = 1:10^2,
y2 = 1:10^3 # Secondary y-axis data (scaled up)
)
# Basic Plot
basic_plot <- ggplot(data, aes(x)) +
geom_line(aes(y = y1, color = "Variable 1")) +
geom_line(aes(y = y2, color = "Variable 2")) +
scale_color_manual(values = c("Variable 1" = "blue", "Variable 2" = "red")) +
labs(title = "Basic Line Plot with Two Variables") +
theme_minimal()
# Customized Plot with Secondary Axis
customized_plot <- basic_plot +
scale_y_continuous(sec.axis = sec_axis(~./10,name = "Secondary Y Axis(scaled down)"))+
labs(title = "Customized Line Plot with Secondary Axis")
# Display the Plot
print(customized_plot)
Output:
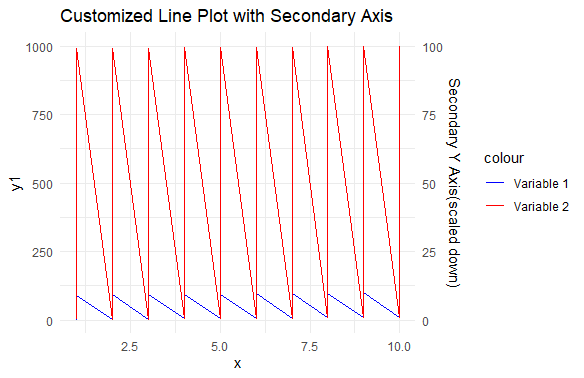
Axes customization in R
It plots a basic line plot with two variables.
- Adding a secondary axis allows us to overlay multiple scales on a single plot, facilitating comparison between variables with different units or scales.
- We add a secondary y-axis for the second variable, scaling it down by a factor of 10 to maintain meaningful interpretation alongside the primary y-axis.
Conclusion
Customizing axes in data visualization with R is essential for creating clear and impactful visualizations. By adjusting axis limits, labels, tick marks, and formatting text, we can better tailor plots to suit our data and enhance interpretability. These customization techniques empower us to highlight key insights and effectively communicate our message through visualizations.
Share your thoughts in the comments
Please Login to comment...