Automate Renaming and Organizing Files with Python
Last Updated :
17 Jul, 2022
In this article, we are going to know how to automate renaming and organizing files with Python, hence, the article is divided into two sections: one teaches us how to organize files and the latter how to rename files.
Here we will learn about how to rename and organize files in Python. We will use different modules such as os and shutil. We will be automating the tasks of moving our downloaded files to the respective folders according to their file types.
What is OS module?
This module helps interact with the operating system and provides a way of using os dependent functionalities. It has many functions such startfile(), getcwd() and many more. In this article, we will use the listdr() and the path.isfile() functions.
What is shutil module?
This module offers a number of high-level operations on files and collection of files. Using it, we can move, copy and rename our files. It has many useful functions such as copyfile(), copymode(), etc. In this article, we will use the move() method of the shutil module to move our files from one place to another.
Note: os and shutil modules are come under Python standard library so, we do not have to install them by pip command we are just importing them in our program.
Syntax of shutil.move()
It recursively moves a file or directory from one place to another, then returns the final destination. Src is placed into the destination directory if it already exists. Depending on the semantics of os.rename(), a destination that already exists but is not a directory may be overwritten.
Syntax: shutil.move(source, destination, copy_function = copy2)
Parameters:
source: path of the source destination directory
destination: path of the destination directory
copy_function (optional): the default value of this parameter is copy2. We can use other copy function like copy, copytree, etc for this parameter.
Return Value: This method returns a string which represents the path of newly created file.
Automate organizing file using Python
To automate organizing files in python firstly we have to import the required modules which are os and shutil then we have to assign the directories we’ll be working to appropriate variables and then make categories of files with the help of tuples such as docs, images and software. after that we have to make a list of all files present in root directory(In our case root directory is Downloads folder) using for loop and methods provided in os module. Lastly we have to move all the files present in downloads folder into their respective folders using move function of shutil module.
Before running the code we’ll need to create 5 folders in the downloads folder: images, documents, software, others, and a Log folder that will store the Log.txt file generated by our Python program. After running the program, we can see that there is nothing but 5 folders that we created in the downloads folder, and inside these 5 folders are the respective files.
NOTE: Before running the script, change the directory to Downloads by typing cd Downloads in the terminal.
Code:
Python3
import os
from shutil import move
root_dir = "C:\\Users\\[Username]\\Downloads"
image_dir = "C:\\Users\\[Username]\\Downloads\\images"
documents_dir = "C:\\Users\\[Username]\\Downloads\\documents"
others_dir = "C:\\Users\\[Username]\\Downloads\\others"
software_dir = "C:\\Users\\[Username]\\Downloads\\softwares"
docs = ( '.docx' , '.doc' , '.txt' , '.pdf' ,
'.xls' , '.ppt' , '.xlsx' , '.pptx' )
images = ( '.jpg' , '.jpeg' , '.png' , '.svg' ,
'.tif' , '.tiff' , '.gif' ,)
softwares = ( '.exe' , '.dmg' , '.pkg' )
files = []
for f in os.listdir(root_dir):
if os.path.isfile(f) and not f.startswith( '.' ) and not f.__eq__(__file__):
files.append(f)
for file in files:
if file .endswith(docs):
move( file , documents_dir + "/" + file )
elif file .endswith(images):
move( file , image_dir + "/" + file )
elif file .endswith(softwares):
move( file , software_dir + "/" + file )
else :
move( file , others_dir + "/" + file )
|
Output:
Automate Renaming files using Python
To automate renaming files using python we have to import os module and then initialize the paths of the files into separate variable that will be given to the rename() function as argument and then Check whether the file with the ‘new name’ already exists with the help of path.isfile() function of the os module. If it does, print a message otherwise just rename the old file to the new file.
Syntax of os.rename()
This function belongs to the os module. It is used to rename files. The syntax is given below:
Syntax: os.rename(source, destination, *, src_dir_fd = None, dst_dir_fd = None)
Parameters:
source: A path-like object representing the file system path. This is the source file path which is to renamed.
destination: A path-like object representing the file system path.
src_dir_fd (optional): A file descriptor referring to a directory.
dst_dir_fd (optional): A file descriptor referring to a directory.
Return Type: This method does not return any value.
Code:
Python3
import os
old = "C:\\Users\\[USERNAME]\\Desktop\\old_name.txt"
new = "C:\\Users\\[USERNAME]\\Desktop\\newname.txt"
if os.path.isfile(new):
print ( "file already exists" )
else :
os.rename(old, new)
|
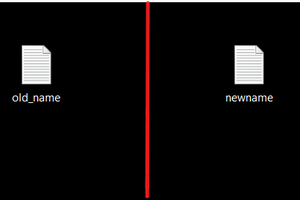
Before and after renaming of a file
Share your thoughts in the comments
Please Login to comment...