Animations in AngularJS Applications
Last Updated :
01 Aug, 2023
AngularJS is the initial version of Angular Framework. As in the latest Angular2+ versions, there are different libraries that can be used to provide advanced animations to the application. Animations are the existing features given to the application that improve the user experiences and also add interaction to our web application. AngularJS does not have any inbuilt animation module through which we can add animation, but using JavaScript and CSS we can apply animations to AngularJS Applications.
In this article, we will understand the JavaScript animations in AngularJS, along with understanding their basic implementation with the help of examples.
Steps to configure AngularJs Animation:
The below steps will be followed for using JavaScript Animations in AngularJS Applications.
Step 1: Create a new folder for the project. As we are using the VSCode IDE, so we can execute the command in the integrated terminal of VSCode.
mkdir angularjs-animation
cd angularjs-animation
Step 2: Create the following files in the newly created folder:
- index.html
- app.js
- styles.css
Step 3: Include Angular.Js animate lib in the script tag as:
<script src=
”https://ajax.googleapis.com/ajax/libs/angularjs/1.8.2/angular-animate.js”
</script>
We will understand the above concept with the help of suitable approaches & will understand its implementation through the illustration.
Approach 1:
In this approach, we are using JavaScript Animation in an AngularJS application by creating an engaging and visually appealing animation for the elements in the user interface. This approach consists of using plain JavaScript and CSS transitions to animate specific components dynamically. Here, the combination of fade-in, pulse animations, etc is used to enhance the user experience.
Example: Below example demonstrates the implementation of Angular.Js animation using JavaScript.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< meta charset = "utf-8" >
< title >
JavaScript Animations in AngularJS
</ title >
< link rel = "stylesheet"
href = "styles.css" >
< script src =
</ script >
< script src = "app.js" ></ script >
</ head >
< body >
< div ng-controller = "myController" >
< div class = "container" >
< h1 id = "heading"
class="animate-fade-in
animate-rotate
animate-pulse">
Hello GeeksforGeeks!
</ h1 >
< h3 id = "paragraph" >
JavaScript Animations in
AngularJS Applications
</ h3 >
< button id = "button"
ng-click = "changeColor()" >
Click Me!
</ button >
</ div >
</ div >
</ body >
</ html >
|
Javascript
var myApp = angular.module( 'myApp' , []);
myApp.controller( 'myController' , function ($scope) {
$scope.changeColor = function () {
var button = document.getElementById( 'button' );
button.style.backgroundColor = getRandomColor();
};
var heading = document.getElementById( 'heading' );
var paragraph = document.getElementById( 'paragraph' );
var button = document.getElementById( 'button' );
fadeIn(heading);
slideIn(paragraph);
fadeIn(button);
});
function fadeIn(element) {
var opacity = 0;
var interval = setInterval( function () {
opacity += 0.05;
element.style.opacity = opacity;
if (opacity >= 1) clearInterval(interval);
}, 50);
}
function slideIn(element) {
var position = -100;
var interval = setInterval( function () {
position += 2;
element.style.transform = 'translateY(' + position + 'px)' ;
if (position >= 0) clearInterval(interval);
}, 10);
}
function getRandomColor() {
var letters = '0123456789ABCDEF' ;
var color = '#' ;
for ( var i = 0; i < 6; i++) {
color += letters[Math.floor(Math.random() * 16)];
}
return color;
}
|
CSS
.container {
text-align : center ;
margin-top : 50px ;
}
h 1 , p, button {
font-size : 24px ;
font-weight : bold ;
margin : 10px ;
padding : 10px ;
}
#heading {
color : green ;
}
.animate-fade-in {
opacity: 0 ;
transition: opacity 0.5 s ease-in-out;
}
.animate-rotate {
transform-origin: center ;
animation: rotateAnimation 3 s linear infinite;
}
.animate-pulse {
animation: pulseAnimation 1 s ease-in-out infinite;
}
@keyframes rotateAnimation {
0% {
transform: rotate( 0 deg);
}
100% {
transform: rotate( 360 deg);
}
}
@keyframes pulseAnimation {
0% {
transform: scale( 1 );
}
50% {
transform: scale( 1.2 );
}
100% {
transform: scale( 1 );
}
}
|
Output:
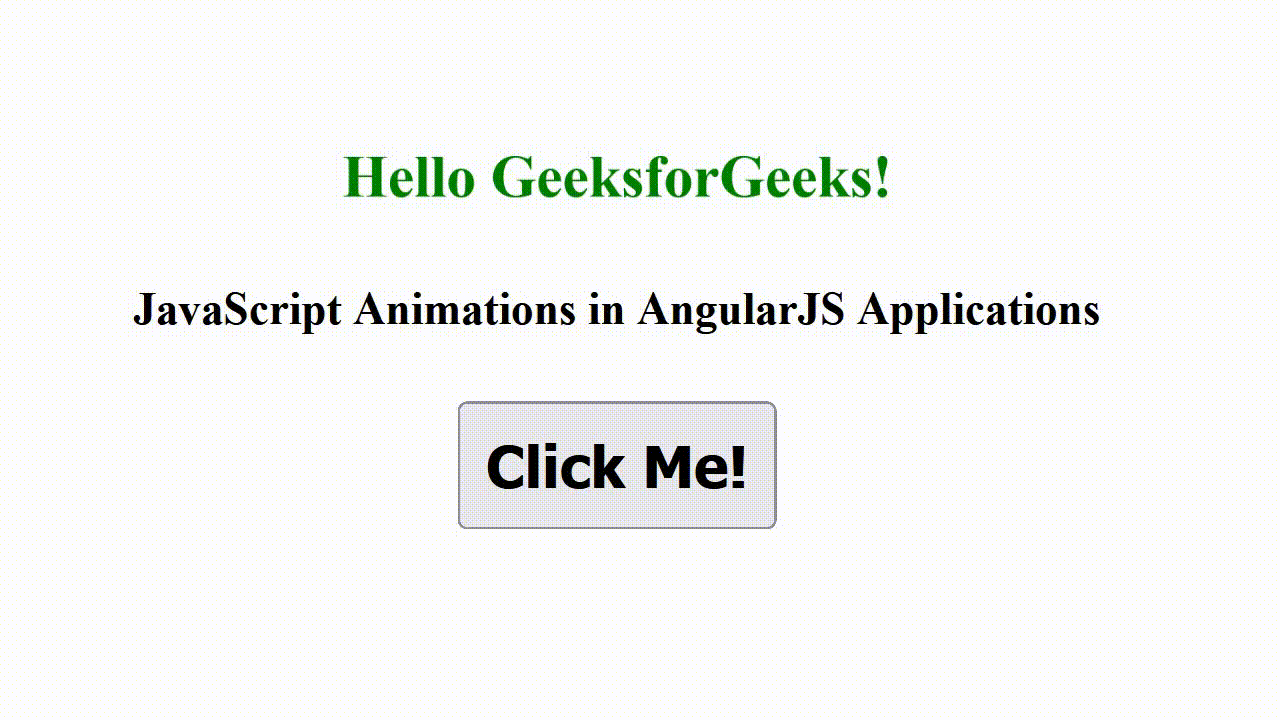
Approach 2:
In this approach, we will use the AngularJS directives to encapsulate and properly manage our JavaScript animations. The directive offers an efficient way to extend the HTML with custom behavior, by developing it for handling animations in an AngularJS application.
Example: This is another example that demonstrates the Angular.Js animation using JavaScript.
HTML
<!DOCTYPE html>
< html ng-app = "myApp" >
< head >
< meta charset = "utf-8" >
< title >
JavaScript Animations in AngularJS Applications
</ title >
< link rel = "stylesheet"
href = "styles.css" >
< script src =
</ script >
< script src = "app.js" ></ script >
</ head >
< body >
< div ng-controller = "myController" >
< div class = "container" >
< h1 id = "heading"
class = "animate-bounce" >
Hello GeeksforGeeks!
</ h1 >
< h3 class="animate-hover
animate-slide-in">
JavaScript Animations in
AngularJS Applications
</ h3 >
< button class = "animate-button"
ng-click = "animateButton()" >
Click Me!
</ button >
</ div >
</ div >
</ body >
</ html >
|
Javascript
var myApp = angular.module( 'myApp' , []);
myApp.directive( 'animateBounce' , function () {
return {
restrict: 'C' ,
link: function (scope, element) {
element.on( 'click' , function () {
element.addClass( 'animate-bounce-effect' );
setTimeout( function () {
element.removeClass( 'animate-bounce-effect' );
}, 500);
});
}
};
});
myApp.directive( 'animateHover' , function () {
return {
restrict: 'C' ,
link: function (scope, element) {
element.on( 'mouseover' , function () {
element.addClass( 'animate-hover-effect' );
});
element.on( 'mouseout' , function () {
element.removeClass( 'animate-hover-effect' );
});
}
};
});
myApp.directive( 'animateSlideIn' , function () {
return {
restrict: 'C' ,
link: function (scope, element) {
element.addClass( 'animate-slide-in-effect' );
}
};
});
myApp.directive( 'animateButton' , function () {
return {
restrict: 'C' ,
link: function (scope, element) {
element.on( 'click' , function () {
element.addClass( 'animate-button-effect' );
setTimeout( function () {
element.removeClass( 'animate-button-effect' );
}, 1000);
});
}
};
});
myApp.controller( 'myController' , function ($scope) {
$scope.animateButton = function () {
};
});
|
CSS
body {
font-family : Arial , sans-serif ;
background-color : #f0f0f0 ;
margin : 0 ;
padding : 0 ;
}
.container {
text-align : center ;
margin-top : 50px ;
}
h 1 ,
h 3 ,
button {
font-size : 24px ;
font-weight : bold ;
margin : 10px ;
padding : 10px ;
border : none ;
cursor : pointer ;
}
#heading {
color : #4CAF50 ;
}
.animate-bounce-effect {
animation: bounceAnimation 0.5 s;
}
.animate-hover-effect {
color : #E60000 ;
}
.animate-slide-in-effect {
animation: slideInAnimation 1 s;
}
.animate-button-effect {
animation: buttonAnimation 1 s;
}
@keyframes bounceAnimation {
0% ,
100% {
transform: translateY( 0 );
}
50% {
transform: translateY( -20px );
}
}
@keyframes slideInAnimation {
0% {
transform: translateY( -100% );
}
100% {
transform: translateY( 0 );
}
}
@keyframes buttonAnimation {
0% {
transform: scale( 1 );
box-shadow: 0 4px 8px rgba( 0 , 0 , 0 , 0.1 );
}
50% {
transform: scale( 1.2 );
box-shadow: 0 8px 16px rgba( 0 , 0 , 0 , 0.2 );
}
100% {
transform: scale( 1 );
box-shadow: 0 4px 8px rgba( 0 , 0 , 0 , 0.1 );
}
}
|
Output:
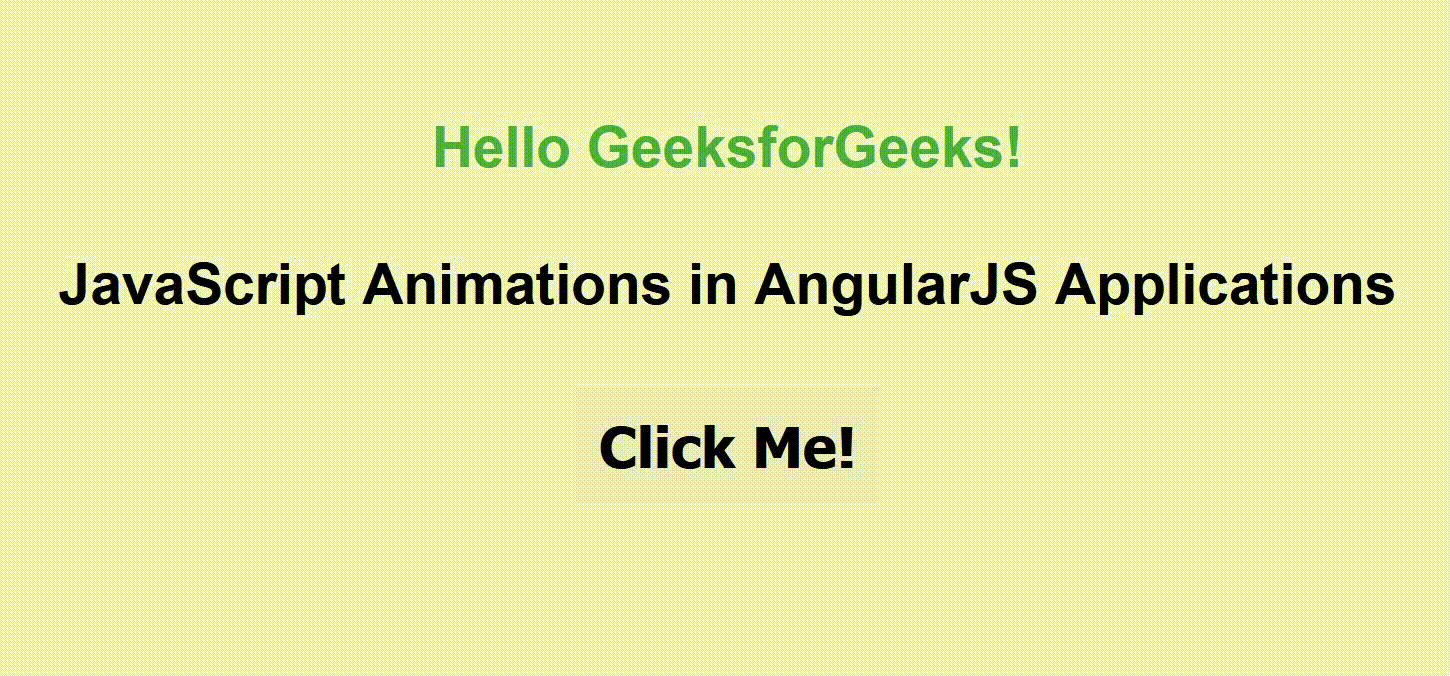
Share your thoughts in the comments
Please Login to comment...