Animating Scatter Plots in Matplotlib
Last Updated :
08 Dec, 2023
An animated scatter plot is a dynamic records visualization in Python that makes use of a series of frames or time steps to reveal data points and exchange their positions or attributes over time. Each body represents a second in time, and the scatter plot is up to date for each frame, allowing you to peer traits, fluctuations, or moves in the information that may not be obvious in a static plot.
Why Use Animated Scatter Plots?
- Temporal Insights: Animated scatter plots are particularly useful when dealing with time-series data or data that changes over time. They help reveal temporal patterns, trends, and correlations that static plots might miss.
- Dynamic Phenomena: When studying dynamic phenomena such as stock market prices, weather patterns, or biological processes, animated scatter plots can provide a more intuitive and insightful view of how variables interact.
- Engaging Presentations: Animated scatter plots can make presentations and reports more engaging and informative. They allow you to convey complex information in a visually appealing way.
Animate a Scatter Plot in Python
Step 1: Install Required Libraries
Ensure you have the necessary libraries installed. You can install them using pip if you haven’t already.
Python3
pip install matplotlib
pip install numpy
pip install celluloid
|
Step 2: Import Libraries
Import the required libraries at the beginning of your Python script:
Python3
import matplotlib.pyplot as plt
from matplotlib import cm
import numpy as np
from celluloid import Camera
|
Step 3: Generate Initial Data
Define the number of data points (numpoints) and generate random initial data points and colors for the scatter plot:
Python3
numpoints = 10
points = np.random.random(( 2 , numpoints))
colors = cm.rainbow(np.linspace( 0 , 1 , numpoints))
|
Step 4: Create a Camera Object
Create a Camera object from the celluloid library to capture frames for the animation.
Step 5: Animation Loop
Create a loop to generate frames for the animation. In each iteration:
- Update the points array to simulate movement (e.g., by adding random displacements).
- Create a scatter plot using plt.scatter.
- Capture the frame using camera.snap().
Python3
points + = 0.1 * (np.random.random(( 2 , numpoints)) - 0.5 )
plt.scatter( * points, c = colors, s = 100 )
camera.snap()
|
Step 6: Create the Animation
After the loop, use the captured frames from the camera object to create the animation:
Python3
anim = camera.animate(blit = True )
|
Step 7: Save the Animation
Save the animation as an MP4 video or another desired format:
Step 8: Display or Use the Animation (Optional)
If you want to display the animation within your script, you can do so using plt.show():
Full Code Implementation
Python3
import matplotlib.pyplot as plt
from matplotlib import cm
import numpy as np
from celluloid import Camera
numpoints = 10
points = np.random.random(( 2 , numpoints))
colors = cm.rainbow(np.linspace( 0 , 1 , numpoints))
camera = Camera(plt.figure())
for _ in range ( 100 ):
points + = 0.1 * (np.random.random(( 2 , numpoints)) - . 5 )
plt.scatter( * points, c = colors, s = 100 )
camera.snap()
anim = camera.animate(blit = True )
anim.save( 'scatter.mp4' )
|
Output
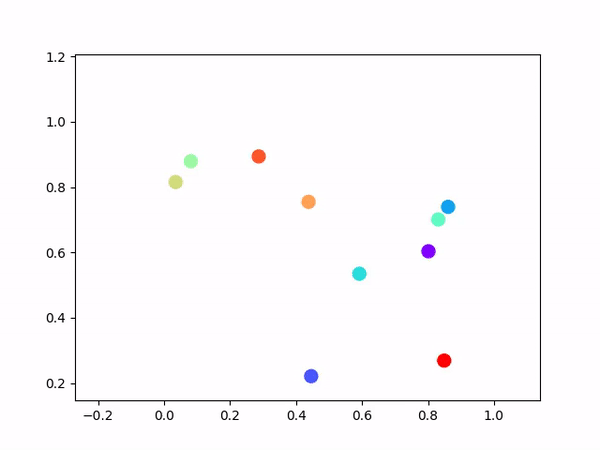
Image Contributed By Gowroju Mani.
Share your thoughts in the comments
Please Login to comment...