AbstractSequentialList in Java with Examples
Last Updated :
14 Feb, 2023
The AbstractSequentialList class in Java is a part of the Java Collection Framework and implements the Collection interface and the AbstractCollection class. It is used to implement an unmodifiable list, for which one needs to only extend this AbstractList Class and implement only the get() and the size() methods.
This class provides a skeletal implementation of the List interface to minimize the effort required to implement this interface backed by a “sequential access” data store (such as a linked list). For random access data (such as an array), AbstractList should be used in preference to this class.
Class Hierarchy:
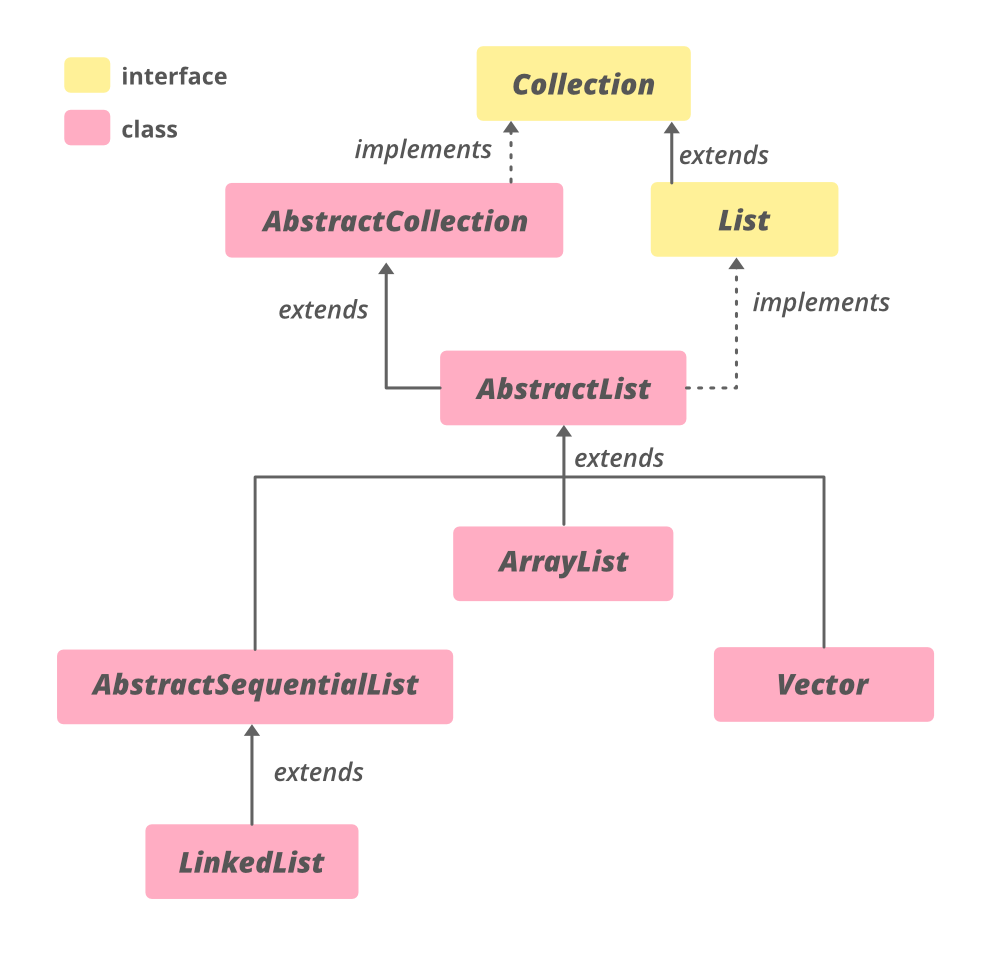
Declaration:
public abstract class AbstractSequentialList<E>
extends AbstractList<E>
Where E is the type of element maintained by this List.
It implements Iterable<E>, Collection<E>, List<E> interfaces. LinkedList is the only direct subclass of AbstractSequentialList.
Constructor: protected AbstractSequentialList() – The default constructor, but being protected, it doesn’t allow to create an AbstractSequentialList object.
AbstractSequentialList<E> asl = new LinkedList<E>();
Example 1: AbstractSequentialList is an abstract class, so it should be assigned an instance of its subclass such as LinkedList.
Java
import java.util.*;
public class GfG {
public static void main(String[] args)
{
AbstractSequentialList<Integer> absl
= new LinkedList<>();
absl.add( 5 );
absl.add( 6 );
absl.add( 7 );
System.out.println(absl);
}
}
|
Output:
[5, 6, 7]
Example 2:
Java
import java.util.*;
import java.util.AbstractSequentialList;
public class AbstractSequentialListDemo {
public static void main(String args[])
{
AbstractSequentialList<String>
absqlist = new LinkedList<String>();
absqlist.add( "Geeks" );
absqlist.add( "for" );
absqlist.add( "Geeks" );
absqlist.add( "10" );
absqlist.add( "20" );
System.out.println( "AbstractSequentialList: "
+ absqlist);
absqlist.remove( 3 );
System.out.println( "Final List: "
+ absqlist);
System.out.println( "The element is: "
+ absqlist.get( 2 ));
}
}
|
Output
AbstractSequentialList: [Geeks, for, Geeks, 10, 20]
Final List: [Geeks, for, Geeks, 20]
The element is: Geeks
Output:
AbstractSequentialList: [Geeks, for, Geeks, 10, 20]
Final List: [Geeks, for, Geeks, 20]
The element is: Geeks
Methods of AbstractSequentialList
METHOD
|
DESCRIPTION
|
add​(int index, E element) |
Inserts the specified element at the specified position in this list (optional operation). |
addAll​(int index, Collection<? extends E> c) |
Inserts all of the elements in the specified collection into this list at the specified position (optional operation). |
get​(int index) |
Returns the element at the specified position in this list. |
iterator() |
Returns an iterator over the elements in this list (in proper sequence). |
listIterator​(int index) |
Returns a list iterator over the elements in this list (in proper sequence). |
remove​(int index) |
Removes the element at the specified position in this list (optional operation). |
set​(int index, E element) |
Replaces the element at the specified position in this list with the specified element (optional operation). |
Methods Inherited From class java.util.AbstractList
METHOD
|
DESCRIPTION
|
add​(E e) |
Appends the specified element to the end of this list (optional operation). |
clear() |
Removes all of the elements from this list (optional operation). |
equals​(Object o) |
Compares the specified object with this list for equality. |
hashCode() |
Returns the hash code value for this list. |
indexOf​(Object o) |
Returns the index of the first occurrence of the specified element in this list, or -1 if this list does not contain the element. |
lastIndexOf​(Object o) |
Returns the index of the last occurrence of the specified element in this list, or -1 if this list does not contain the element. |
listIterator() |
Returns a list iterator over the elements in this list (in proper sequence). |
removeRange​(int fromIndex, int toIndex) |
Removes from this list all of the elements whose index is between fromIndex, inclusive, and toIndex, exclusive. |
subList​(int fromIndex, int toIndex) |
Returns a view of the portion of this list between the specified fromIndex, inclusive, and toIndex, exclusive. |
Methods Inherited From class java.util.AbstractCollection
METHOD
|
DESCRIPTION
|
addAll​(Collection<? extends E> c) |
Adds all of the elements in the specified collection to this collection (optional operation). |
contains​(Object o) |
Returns true if this collection contains the specified element. |
containsAll​(Collection<?> c) |
Returns true if this collection contains all of the elements in the specified collection. |
isEmpty() |
Returns true if this collection contains no elements. |
remove​(Object o) |
Removes a single instance of the specified element from this collection, if it is present (optional operation). |
removeAll​(Collection<?> c) |
Removes all of this collection’s elements that are also contained in the specified collection (optional operation). |
retainAll​(Collection<?> c) |
Retains only the elements in this collection that are contained in the specified collection (optional operation). |
toArray() |
Returns an array containing all of the elements in this collection. |
toArray​(T[] a) |
Returns an array containing all of the elements in this collection; the runtime type of the returned array is that of the specified array. |
toString() |
Returns a string representation of this collection. |
Methods Inherited From Interface java.util.Collection
METHOD
|
DESCRIPTION
|
parallelStream() |
Returns a possibly parallel Stream with this collection as its source. |
removeIf​(Predicate<? super E> filter) |
Removes all of the elements of this collection that satisfy the given predicate. |
stream() |
Returns a sequential Stream with this collection as its source. |
toArray​(IntFunction<T[]> generator) |
Returns an array containing all of the elements in this collection, using the provided generator function to allocate the returned array. |
Methods Inherited From Interface java.lang.Iterable
METHOD
|
DESCRIPTION
|
forEach​(Consumer<? super T> action) |
Performs the given action for each element of the Iterable until all elements have been processed or the action throws an exception. |
Methods Inherited From Interface java.util.List
METHOD |
DESCRIPTION |
addAll​(Collection<? extends E> c) |
Appends all of the elements in the specified collection to the end of this list, in the order that they are
returned by the specified collection’s iterator (optional operation).
|
contains​(Object o) |
Returns true if this list contains the specified element. |
containsAll​(Collection<?> c) |
Returns true if this list contains all of the elements of the specified collection. |
isEmpty() |
Returns true if this list contains no elements. |
remove​(Object o) |
Removes the first occurrence of the specified element from this list, if it is present (optional operation). |
removeAll​(Collection<?> c) |
Removes from this list all of its elements that are contained in the specified collection (optional operation). |
replaceAll​(UnaryOperator<E> operator) |
Replaces each element of this list with the result of applying the operator to that element. |
retainAll​(Collection<?> c) |
Retains only the elements in this list that are contained in the specified collection (optional operation). |
size() |
Returns the number of elements in this list. |
sort​(Comparator<? super E> c) |
Sorts this list according to the order induced by the specified Comparator. |
spliterator() |
Creates a Spliterator over the elements in this list. |
toArray() |
Returns an array containing all of the elements in this list in proper sequence (from first to last element). |
toArray​(T[] a) |
Returns an array containing all of the elements in this list in proper sequence (from first to last element);
the runtime type of the returned array is that of the specified array.
|
The AbstractSequentialList class in Java is a subclass of AbstractList that provides a skeletal implementation of the List interface, specifically for lists that allow sequential access to their elements. This means that elements can be accessed in a predictable order, such as first to last.
Here is an example of how you can use the AbstractSequentialList class in Java:
Java
import java.util.AbstractSequentialList;
import java.util.List;
import java.util.ListIterator;
public class MyList extends AbstractSequentialList<Integer> {
private int size;
public MyList( int size) {
this .size = size;
}
@Override
public ListIterator<Integer> listIterator( int index) {
return new ListIterator<Integer>() {
private int currentIndex = index;
@Override
public boolean hasNext() {
return currentIndex < size;
}
@Override
public Integer next() {
return currentIndex++;
}
@Override
public boolean hasPrevious() {
return currentIndex > 0 ;
}
@Override
public Integer previous() {
return currentIndex--;
}
@Override
public int nextIndex() {
return currentIndex + 1 ;
}
@Override
public int previousIndex() {
return currentIndex - 1 ;
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
@Override
public void set(Integer integer) {
throw new UnsupportedOperationException();
}
@Override
public void add(Integer integer) {
throw new UnsupportedOperationException();
}
};
}
@Override
public int size() {
return size;
}
public static void main(String[] args) {
List<Integer> list = new MyList( 5 );
for ( int i : list) {
System.out.println(i);
}
}
}
|
By extending the AbstractSequentialList class, you only need to implement the listIterator and size methods, which provides a basic implementation of a sequential list. This can save you a lot of time and code compared to implementing the List interface from scratch.
Advantages of using AbstractSequentialList in Java:
- Reduced code duplication: By using the AbstractSequentialList class as a base, you can reduce the amount of code that you need to write to implement a sequential list, since many of the common methods have already been implemented for you.
- Consistent behavior: Since the AbstractSequentialList class implements many of the methods in the List interface, you can be sure that your implementation will have consistent behavior with other sequential list implementations, like LinkedList.
Disadvantages of using AbstractSequentialList in Java:
- Limited functionality: Since the AbstractSequentialList class is an abstract class, it provides only a basic implementation of a sequential list. You may need to implement additional methods to provide the full functionality required by your application.
- Increased complexity: By extending the `AbstractSequential
Reference: https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/util/AbstractSequentialList.html
Share your thoughts in the comments
Please Login to comment...