A Complete Guide to Learn Kotlin For Android App Development
Last Updated :
07 Jul, 2021
Kotlin is a statically typed, cross-platform, general-purpose programming language for JVM developed by JetBrains. This is a language with type inference and fully interoperable with Java. Kotlin is concise and expressive programming as it reduces the boilerplate code. Since Google I/O 2019, Android development has been Kotlin-first. Kotlin is seamlessly integrated with the Android Studio and many companies are moving the entire code base from Java to Kotlin. Asynchronous tasks are seamlessly implemented in Kotlin using coroutines. So here’s the complete guide to learn Kotlin, specifically for Android Application Development.
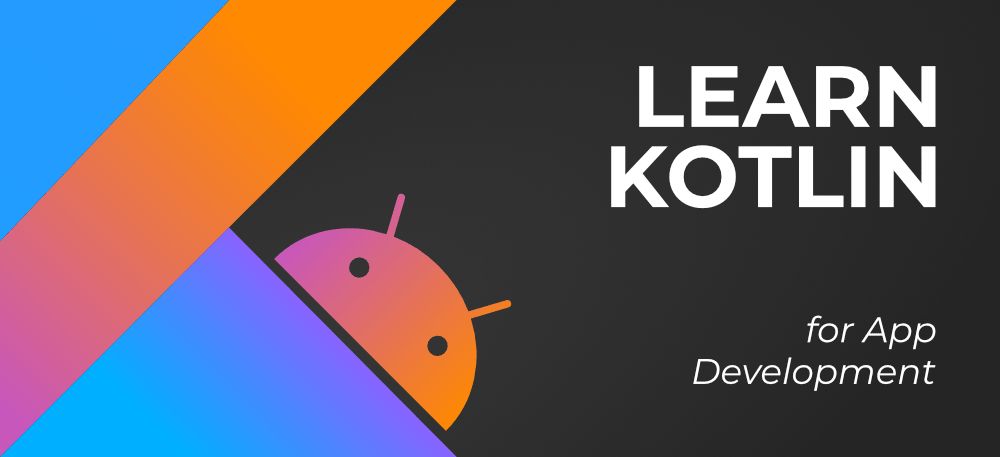
So in this article, we have covered the following things:
- Basics Of Kotlin Programming Language
- Conditional Statements(Control Flow) in Kotlin
- Functional Programming in Kotlin
- Collections in Kotlin Programming Language
- Object-Oriented Programming Concepts of Kotlin
- Kotlin Exception Handling
- Kotlin Null Safety
- Kotlin Scope Functions
- Kotlin Interoperability with Java
- Kotlin Coroutines
- Miscellaneous
- Complete Kotlin Tutorial
Step-by-Step Guide to Learn Kotlin for Android App Development
Basics Of Kotlin Programming Language
fun main(args: Array<String>) {
println("Hello, World!")
}
- Kotlin Data Types – Fundamental data type in Kotlin is a Primitive data type and all others are reference types like array and string.
- Kotlin Variables – The mutable and immutable data types in Kotlin.
Conditional Statements(Control Flow) in Kotlin
Functional Programming in Kotlin
- Kotlin functions – Basics of functions and their declaration in Kotlin.
- Kotlin | Default and Named argument – Make the parameters of the function optional. i.e pass an argument or not while calling a function.
- Lambdas Expressions and Anonymous Functions – Syntax of Kotlin lambdas is similar to Java Lambdas. A function without a name is called an anonymous function.
- Kotlin Inline functions – Interesting inline keyword which ultimately requests the compiler to not allocate memory and simply copy the inlined code of that function at the calling place.
- Kotlin infix function notation – A function marked with infix keyword can also be called using infix notation, which means calling without using parenthesis and dots.
- Kotlin Higher-Order Functions – Kotlin functions can be stored in variables and data structures, passed as arguments to, and returned from other higher-order functions.
Collections in Kotlin Programming Language
- Kotlin Collections – A collection usually contains a number of objects of the same type and these objects in the collection are called elements or items.
- Kotlin list : Arraylist – Dynamic array states that we can increase or decrease the size of an array as pre-requisites.
- Kotlin list : listOf() – Kotlin has two types of lists, immutable lists (cannot be modified) and mutable lists (can be modified).
- Kotlin Set : setOf() – Kotlin Set interface is a generic unordered collection of elements, and it does not contain duplicate elements.
- Kotlin mutableSetOf() – setOf() is immutable, meaning it supports only read-only functionalities and mutableSetOf() is mutable, meaning it supports both read and write.
- Kotlin hashSetOf() It implements the set interface. hashSetOf() is a function that returns a mutable hashSet, which can be both read and written.
- Kotlin Map : mapOf() – Map holds the data in the form of pairs which consist of a key and a value.
- Kotlin Hashmap – Kotlin Hash Table-based implementation of the MutableMap interface. It stores the data in the form of key and value pairs.
Object-Oriented Programming Concepts of Kotlin
- Kotlin Class and Objects – Class and Objects are the basic concepts of an object-oriented programming language. These support the OOPs concepts of inheritance, abstraction, etc.
- Kotlin Nested class and Inner class – A class is declared within another class, then it is called a nested class.
- Kotlin Setters and Getters – setter is used to set the value of any variable and getter is used to get the value. Getter and Setter are auto-generated in the code.
- Kotlin | Class Properties and Custom Accessors – So, accessor methods – a getter and a setter are provided to let the clients of the given class access the data.
- Kotlin constructor – The primary constructor initializes the class, while the secondary constructor is used to initialize the class and introduce some extra logic.
- Kotlin Visibility Modifiers – Visibility modifiers are used to restrict the accessibility of classes, objects, interfaces, constructors, functions, properties, and their setters to a certain level.
- Kotlin Inheritance – Inheritance enables code re-usability. It allows inheriting the features from an existing class(base class) to a new class(derived class).
- Kotlin Interfaces – Interfaces are custom types provided by Kotlin that cannot be instantiated directly.
- Kotlin Data Classes – We often create classes to hold some data in them. In such classes, some standard functions are often derivable from the data.
- Kotlin Sealed Classes – As the word sealed suggests, sealed classes conform to restricted or bounded class hierarchies.
- Kotlin Abstract class – An abstract class can not instantiate. It means we can not create objects for the abstract class.
- Enum classes in Kotlin – enum has its own specialized type, indicating that something has a number of possible values. Unlike Java enums, Kotlin enums are classes.
- Kotlin extension function – When a function is added to an existing class it is known as an Extension Function.
- Kotlin generics – Allow defining classes, methods, and properties that are accessible using different data types while keeping a check of the compile-time type safety.
Kotlin Exception Handling
Kotlin Null Safety
- Kotlin Null Safety – Kotlin’s type system is aimed to eliminate the jeopardy of null reference from the code because it is a billion-dollar mistake.
- Kotlin | Type Checking and Smart Casting – It is a way of checking the type of the variable at runtime.
- Kotlin | Explicit type casting – Kotlin also provides a facility of typecasting using safe cast operator as. If casting is not possible, it returns null instead of throwing a ClassCastException exception.
Kotlin Scope Functions
Kotlin Interoperability with Java
Kotlin Coroutines
Miscellaneous
- Kotlin annotations – Annotations are a feature of Kotlin that allows the programmer to embed supplemental information into the source file.
- Kotlin Reflection – Along with Java reflection API, Kotlin also provides its own set of reflection API, in a simple functional style.
- Delegation in Kotlin – Delegation controls the allocation of power/authority from one instance to another for any object.
- Delegated Properties in Kotlin – Delegation is defined as the granting of any authority or power to another person (Boss assigning tasks to its employees) to carry out different work.
For a complete Kotlin Tutorial, you may refer to this article: Kotlin Programming Language
Share your thoughts in the comments
Please Login to comment...