Android development has made a significant contribution to the world of mobile development. For Android development choosing the correct language is paramount. Kotlin and Java are the languages used for Android development. Both languages have their own Pros and Cons. Kotlin is a modern language developed by JetBrains and declared as the official language for Android Development.
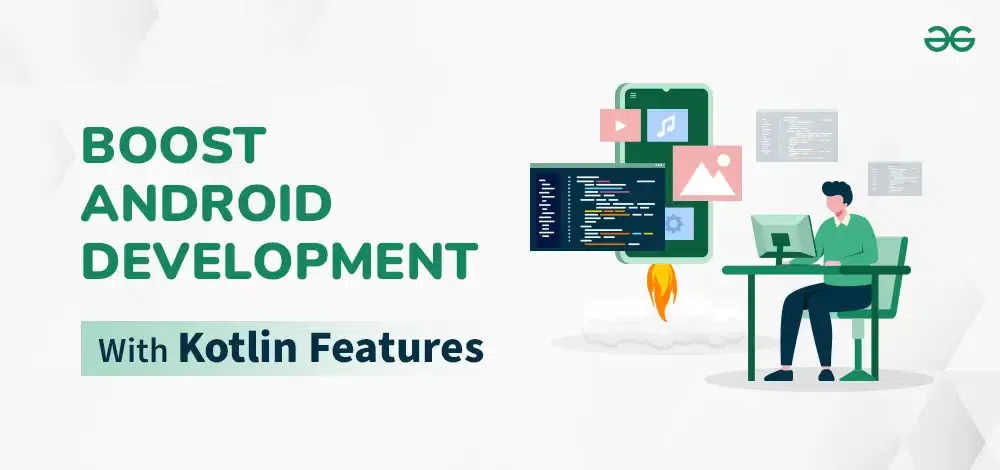
Today, in this article we will look into 10 features of Kotlin that can help Developers to boost Android development. Whether it is a Null safety feature, Extension function, coroutine, Android KTX, or Scope function each plays a unique role in making development faster compared with Java. A strong grip on these features can make a substantial difference in your productivity, code quality, and overall development workflow.
10 Features of Kotlin to Boost Android Development
Kotlin has emerged as a game-changer, offering a powerful and concise alternative to traditional Java. If you’re looking to supercharge your Android app development, here are 10 must-know Kotlin features that will elevate your coding experience and streamline your projects.
1. View Binding – With Binding Class
To use views defined in XML layouts in Activity or Fragments developers have to use the FindViewById method to map view from XML to View variable defined in Fragment of Activity. The View Binding feature in Android makes it easier to interact with Views defined in XML. It creates a Binding class for each XML layout file.
View binding is Null-safe and Eliminates the need for the findViewById() method.
Steps to use View Binding
- Create a Binding class Instance
- Define and Initilize root view
- Pass the root view to the setContentView() to make it available to use in the entire class.
Let’s Understand with the use of an Example
XML
<? xml version = "1.0" encoding = "utf-8" ?>
< androidx.constraintlayout.widget.ConstraintLayout
android:layout_width = "match_parent"
android:layout_height = "match_parent"
tools:context = ".ui.MainActivity"
android:layout_margin = "20dp" >
< androidx.appcompat.widget.AppCompatButton
android:id = "@+id/btn_open_single_api"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:text = "@string/single_api_call"
app:layout_constraintEnd_toEndOf = "parent"
app:layout_constraintStart_toStartOf = "parent"
app:layout_constraintTop_toTopOf = "parent" />
< androidx.appcompat.widget.AppCompatButton
android:id = "@+id/btn_multi_single_api"
android:layout_width = "match_parent"
android:layout_height = "wrap_content"
android:text = "@string/multi_api_call"
app:layout_constraintEnd_toEndOf = "parent"
app:layout_constraintStart_toStartOf = "parent"
app:layout_constraintTop_toBottomOf = "@id/btn_open_single_api" />
</ androidx.constraintlayout.widget.ConstraintLayout >
</ layout >
|
Kotlin
class MainActivity: AppCompatActivity() {
var dataBinding: ActivityMainBinding ? = null
override fun onCreate(savedInstanceState: Bundle ? ) {
super .onCreate(savedInstanceState)
dataBinding = DataBindingUtil.setContentView( this , R.layout.activity_main)
dataBinding?.btnOpenSingleApi?.setOnClickListener {
startActivity(Intent( this @MainActivity , SingleAPICallActivity:: class .java))
}
dataBinding?.btnMultiSingleApi?.setOnClickListener {
startActivity(Intent( this @MainActivity , MultipleAPICallActivity:: class .java))
}
}
}
|
In Above example we can see that buttons btn_multi_single_api and btn_open_single_api are being accessed in MainActivity class using data binding without using findViewById() method.
2. Null Safety
One of the most significant features of the Kotlin language is the Null safety system. Variables in Kotlin are by default non Nullable, that eliminates the most Annoying NullPointerException that often plague Java Developers. By just making nullability explicit with the ‘?’ operator, Kotlin helps developers to write more safer and reliable code.
This feature also eliminates the need of adding Null checks in code which make code more clean and easy to read.
Let’s loot at example to understand it well.
Kotlin
var name: String ? = null
name?.toUpperCase()
if (name != null )
name.toUpperCase()
else
null
|
3. Extension Functions
Extension functions allow developers to use existing classes without using their source code. Extension functions behave like regular member functions of the class that are defined outside of the class. In short we can say it enhances the code without Inheritance. Extension function helps to reuse code and decreases code redundancy.
Let’s understand Extension function using example:
Kotlin
fun String.capitalizeFirstLetter(): String {
return
if (isNotEmpty()) {
this [ 0 ].toUpperCase() + substring( 1 )
} else {
this
}
}
fun main() {
val sentence = "hello, gfg!"
val capitalizedSentence = sentence.capitalizeFirstLetter()
println(capitalizedSentence)
}
|
4. Scope Function
Scope functions are one of the most used and essential features, scope functions are defined as extension functions on objects. It makes code more concise and readable. There are five types of Scope functions available with Kotlin. ‘let’, ‘with’, ‘apply’, ‘apply’ and ‘run’.
let – It executes the code block and returns the result of the last expression in the block. It also helps to prevent null pointer exceptions. Let is extension function.
Kotlin
val result = "Hello, GFG" .let {
it.length
}
|
run – Run is similar to let but it operates on objects not on its properties. It takes ‘this’ as a context object and returns lambda result. Run is extension function.
Kotlin
val result = "Hello,GFG" .run {
length
}
|
apply – Apply is similar to run, but returns a receiver object. Mostly it is used for Object initilization. Apply is extension function.
Kotlin
val player = Employee().apply {
name = "Sachin"
age = 35
}
|
with – With takes object and lambda expression as parameter and returns last expression in block.With is not extension function.
Kotlin
val result = with( "Hello, GFG" ) {
length
}
|
also – Also helps to perform additional operations on the object and returns the original object. Also is extension function.
Apply and Also returns the context Object.
let, run and with returns the lambda results.
5. Data Classes
In Java we use POJO – Model classes frequently to hold data, where we declare class properties and its getter-setter methods. Kotlin has introduced data classes that serve the same purpose. Developers can directly access the property without any need of getter or setter methods. When we use data classes it automatically overrides below methods.
Data classes can be created by just preceding class name with data keyword. Properties of data classes can be defined directly in primary constructors.
- equals()
- hashCode()
- toString()
- copy()
Kotlin
data class Student(val name: String, val rollNumber: Int)
val student = Student(“Sehwag”, 1 )
println(student.name)
println(student.rollNumber)
|
6. Sealed Classes
Sealed classes in Kotlin are used to restrict class hierarchies. Specifically useful when you want to represent a finite set of classes. It is very similar to Enum. Most common use case of sealed class is to manage API success and failure response, let’s understand with an example.
Kotlin
sealed class APIResponse {
data class Success(val data: String): APIResponse()
data class Error(val message: String): APIResponse()
}
|
7. Coroutines
Coroutines were introduced in Kotlin to manage code execution asynchronously. Its framework that manages the threads. It provides a suspend method that can be suspended and resumed whenever needed.
Below are key features of Coroutines.
- Lightweight
- Less memory leaks
- Cancellation support
- Jetpack Integration
Coroutine comes up with scope that can define Coroutine scope – in which Coroutine can be executed. Below are scopes of coroutine.
- Globle Scope – Coroutine with this scope long live as application does. So scope will remain until application is live.
- LifeCycle Scope – LifeCycle scope is also similar to the Global Scope but the difference is LifeCycle scope live as long as Activity does, So once activity is destroyed, this scope will also destroyed.
- ViewModel Scop – Same aas LifeCycle and Global Scope, ViewModel will be live until view model is alive.
Kotlin
suspend fun fetchData(userId: Int): String {
delay( 1000 )
return "Data to be returned"
}
runBlocking {
val data = async {
fetchData( 1 )
}
}
|
8. Lambda Expressions
Kotlin provides concise syntax for creating anonymous functions using Lambda Expressions. Specifically for handling callback functions this feature is very useful. Syntax of lambda expressions in Kotlin is very similar to java lambdas. In Kotlin Lambda expressions are also passed as function parameters which are called Higher Order Functions.
Let’s have a look at an Example.
Kotlin
val sum: (Int, Int) -> Int = { parameter1, parameter2 -> parameter1 + parameter2 }
|
9. Higher order functions
Higher order functions are a very powerful feature of Kotlin, Its function that takes function as parameter and returns a function.It helps developers to write concise and expressive code. Higher order functions provide Code Reusability, Abstraction and Flexibility in writing code to developers. Initially its bit hard to understand the Higher Order Functions, but once you have good grip over it will help to reduce code redundancy and make code more readable then before.
Let’s look at Example of Higher Order Functions.
Kotlin
fun calculateSum(x: Int, y: Int, operation: (Int, Int) -> Int): Int {
return operation(x, y)
}
fun add(x: Int, y: Int): Int {
return x + y
}
val result1 = calculate( 10 , 15 , ::add)
println( "Addition: $result1" )
|
10. Android KTX
Google has announced android-ktx, a set of Kotlin extensions for Android application development. It is very helpful in rapid development. The KTX tool kit is divided into a number of different packages so you can import only those which you need in your project.
To use Android KTX, developers just need to add required dependency in app’s build.gradle file.
- Core KTX
- Fragment KTX
- SQLite KTX
- Collection KTX
Example:
Kotlin
/ Without KTX
val uri = Uri.parse(myUriString)
val uri = myUriString.toUri()
sharedPreferences.edit()
.putBoolean( "key" , value)
.apply()
sharedPreferences.edit {
putBoolean( "key" , value)
}
|
Along with Extension function KTX also provides Extension properties, but to use properties we have to provide explicitly getter.
Conclusion
Using above listed features helps developers a lot to make development easier and faster. Each feature has its own advantages, like Null Safety can take care of NullPointerException, Coroutine can help in making async operation more efficient, Data classes helps to reduce boilerplate code and Scope functions make object operations easy. Android KTX has an exceptional contribution in making code faster and concise with keeping good quality of code.
By adopting those features in routine coding can help in faster development with concise code. Start using them in your projects.
Share your thoughts in the comments
Please Login to comment...