Words to Numbers Converter Tool in Python
Last Updated :
22 Mar, 2024
In this tutorial, we will guide you through the process of building a Word-to Numbers converter using Python. To enhance the user experience, we will employ the Tkinter library to create a simple and intuitive Graphical User Interface (GUI). This tool allows users to effortlessly convert words into numbers by inputting the desired word, clicking a single button, and witnessing the automatic conversion.
Words to Numbers Converter Tool in Python
Below is the step-by-step implementation of the Words to Numbers Converter tool in Python:
Step 1: Create the Virtual Environment
Firstly, Create the virtual environment using the below commands
python -m venv env
.\env\Scripts\activate.ps1
Step 2: Install Necessary Library
Using the command below, install the tkinter, and word2number converter libraries to convert words into numbers.
pip install tk
pip install word2number
File Structure
Make a file named as main.py and our file structure will look like this..
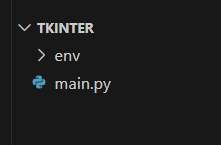
File Structure
Step 3: Writing Python Tkinter Code
main.py: In this example, below Python code uses the tkinter library to create a simple graphical user interface for a words-to-numbers converter. The application window includes a label prompting the user to enter a numeric word, an entry field for input, a “Convert” button, and a label to display the result. The `convert_to_number` function is triggered when the button is pressed, extracting the input from the entry field, attempting to convert it using the word2number library, and updating the result label accordingly.
Python3
import tkinter as tk
from word2number import w2n
def convert_to_number():
input_text = entry.get()
try:
result = w2n.word_to_num(input_text)
result_label.config(text=f"Result: {result}")
except ValueError:
result_label.config(
text="Invalid input, please enter a valid numeric word.")
# Create the main window
app = tk.Tk()
app.title("Words to Numbers Converter")
# Create and place widgets
label = tk.Label(app, text="Enter a numeric word:")
label.pack(pady=10)
entry = tk.Entry(app, width=30)
entry.pack(pady=10)
convert_button = tk.Button(app, text="Convert", command=convert_to_number)
convert_button.pack(pady=10)
result_label = tk.Label(app, text="Result: ")
result_label.pack(pady=10)
# Run the application
app.mainloop()
Output
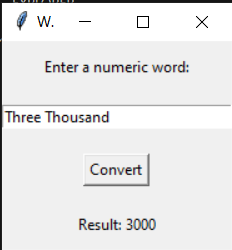
Converted 3000
Video Demonstration
Conclusion
In conclusion, the “Words to Numbers Converter” is a Python program utilizing the tkinter library to create a user-friendly graphical interface. Users can input a numeric word, and upon pressing the “Convert” button, the application leverages the word2number library to convert the word into its numeric equivalent. The concise and intuitive design of the converter allows for easy interaction, making it a practical tool for converting words to numbers.
Share your thoughts in the comments
Please Login to comment...