Render dynamic content in Node using Template Engines
Last Updated :
07 Mar, 2024
In Node.js, the rendering of dynamic content using Templates like EJS, Handlebars, and Pug consists of embedding dynamic data and logic directly into the HTML files. In this article, we will use the EJS Template Engine to render dynamic content in Node.js using Templates.
We will explore the practical demonstration of the concept in terms of example and output.
Why Use Templates?
- Templates mainly allow us to separate the HTML structure from the data and logic.
- Using Templates, the reuse components and layouts across multiple pages and also reduces redundancy and makes it easier to update the UI of the application.
- By using the Templates, we can easily inject the dynamic content into our HTML, this allows us to display the information which changes according to the user input or database queries.
- Templates also provide a consistent structure of the HTML, which enhances the look and feel throughout the various pages of the application.
Popular Template Engines for Node.js
- EJS (Embedded JavaScript): Lightweight and easy-to-use Template. This is similar to plain HTML with embedded JavaScript. EJS supports control flow structures and includes.
- Handlebars: Handlebars provide simple syntax with curly braces, and also support partials and helpers. Handlebars mainly focus on simplicity and ease of use.
- Pug (Jade): Pug uses indentation instead of HTML tags for structure. Pug has concise and expressive syntax. Pug supports mixins for reusable components.
- Mustache: Mustache is minimalistic and language-agnostic. It has simple placeholders for variable substitution. Mustache supports logic-less templates.
- Nunjucks: Nunjucks is based on Jinja2 (Python templating engine). Supports template inheritance and macros. Rich feature set with filters and extensions.
Steps to render dynamic content in Node.js using Template Engine:
Step 1: Create a backend server using the following command in your project folder.
npm init -y
Step 2: Install the necessary package in your server using the following command.
npm i express ejs
Folder Structure:
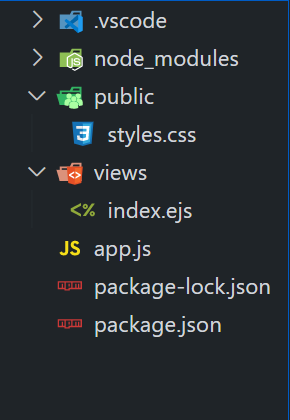
The updated dependencies in package.json file will look like:
"dependencies": {
"express": "^4.18.2",
"ejs": "^3.1.9",
}
Approach to render dynamic content using Template Engine:
- The below example uses Express and EJS templates to create a dynamic web application.
- It defines a route to render an HTML template that includes a form for user input.
- When the user submitst the form, the server processes the input, performs calculations (square and cube), and dynamically updates the rendered content with the results, demonstrating the feature of EJS for injecting dynamic data into the HTML template.
Example: Below is an example of rendering dynamic content in Node.js using templates.
HTML
<!DOCTYPE html>
< head >
< link rel = "stylesheet" href = "/styles.css" >
< title >Node Template App</ title >
</ head >
< body >
< h1 style = "color: green;" >GeeksforGeeks</ h1 >
< h3 >Render dynamic content in Node.js using templates </ h3 >
< form action = "/calculate" method = "post" >
< label for = "number" >Enter a number:</ label >
< input type = "number" id = "number" name = "number" required>
< button type = "submit" >Calculate</ button >
</ form >
<% if (result) { %>
< p >Square: <%= result.square %></ p >
< p >Cube: <%= result.cube %></ p >
<% } %>
</ body >
</ html >
|
CSS
body {
font-family : Arial , sans-serif ;
text-align : center ;
margin : 40px ;
}
form {
margin-top : 20px ;
}
p {
font-weight : bold ;
color : green ;
}
|
Javascript
const express = require( 'express' );
const bodyParser = require( 'body-parser' );
const path = require( 'path' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
app.use(express.static(path.join(__dirname, 'public' )));
app.use(bodyParser.urlencoded({ extended: false }));
app.get( '/' , (req, res) => {
res.render( 'index' , { result: null });
});
app.post( '/calculate' , (req, res) => {
const number = parseInt(req.body.number);
const square = Math.pow(number, 2);
const cube = Math.pow(number, 3);
res.render( 'index' , { result: { square, cube } });
});
app.listen(port, () => {
console.log(`Server is running at http:
});
|
To run the application, we need to start the server by using the below command.
node app.js
Output:
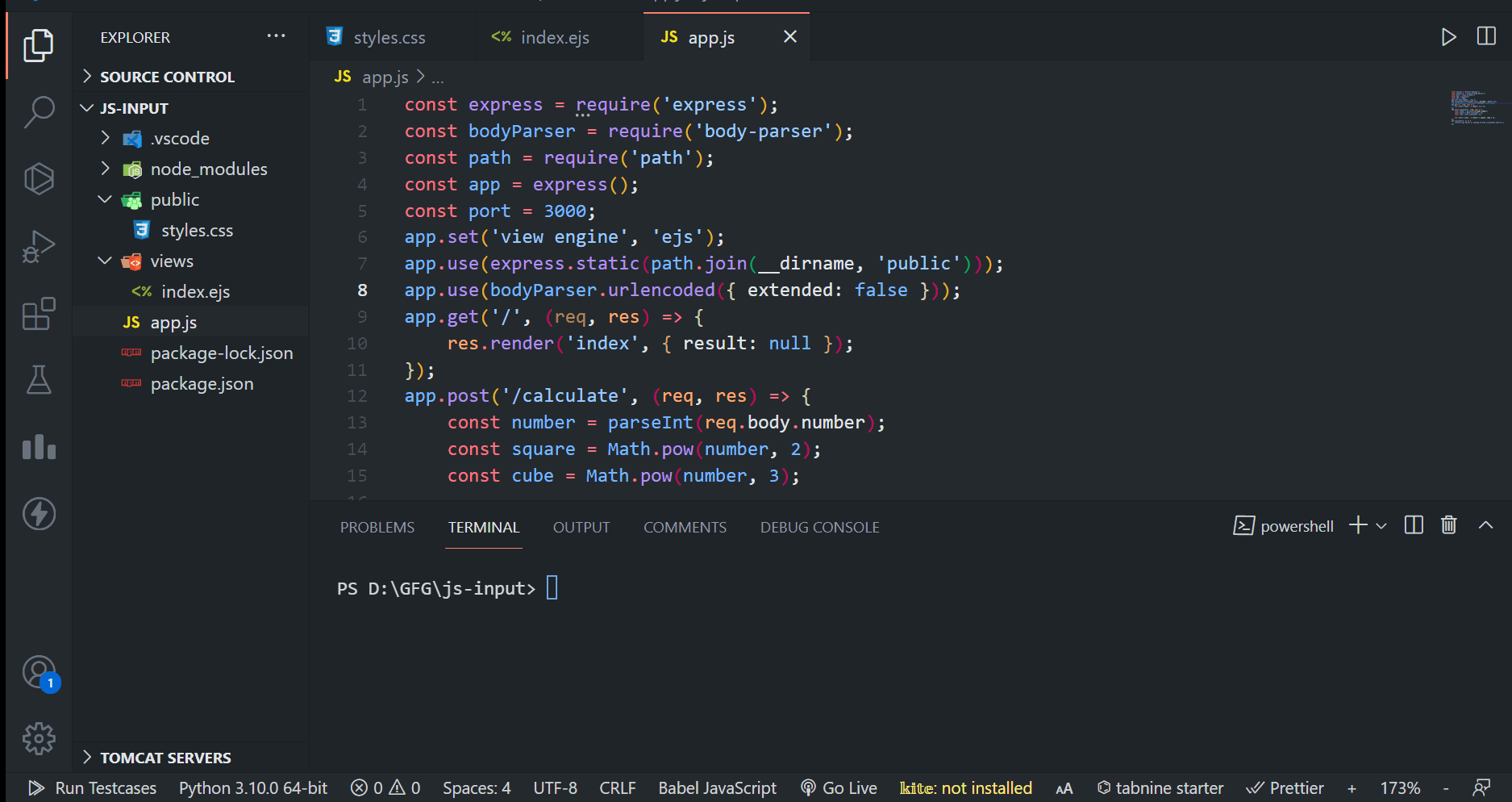
Share your thoughts in the comments
Please Login to comment...