GFact | Why is Circular Reference BAD in JavaScript?
Last Updated :
06 Oct, 2023
Circular referencing is an idea when an object directly or indirectly refers to itself back. It creates a closed loop. Like other programming languages, JavaScript also faces this problem of circular referencing.
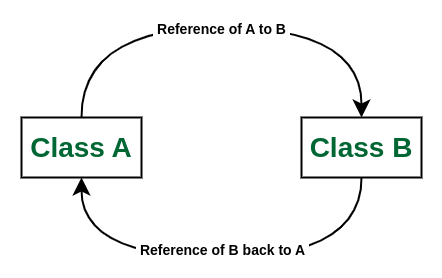
circular reference in javascript
Example:
Javascript
var user = {
first_name: 'Geeks'
};
user.self = user;
console.log( "The user:" , user);
console.log( "The user using self pointer:" , user.self);
console.log( "The user using double self pointer:" , user.self.self);
|
Output
The user: <ref *1> { first_name: 'Geeks', self: [Circular *1] }
The user using self pointer: <ref *1> { first_name: 'Geeks', self: [Circular *1] }
The user using double self pointer: <ref *1> { first_...
Why circular reference is bad ?
A circular reference occurs if two separate objects pass references to each other.
Older browsers especially IE6-7 were infamous for creating memory leaks as their garbage collector algorithm couldn’t handle couldn’t handle circular references between DOM objects and JavaScript objects. Sometimes faulty browser extensions might also be the cause of leaks. For example, FlashGot extension in firefox once created a memory leak.
However, from a pure design point of view, circular referencing is still a bad thing and a code smell. Circular referencing implies that the 2 objects referencing each other are tightly coupled and changes to one object may need changes in other as well.
How to Avoid circular references ?
There are a couple of ways you can solve this problem:
1) Use libraries
2) Implement a solution yourself.
3) Serialization : One major way to solve this is by using Serialization in JavaScript .
- This process involves serializing the object to remove some properties from an object before converting it to JSON.
- In this process, you can remove properties you are not interested in, or in our case, properties that can cause errors.
Below is the simple solution using serialization:
Javascript
const obj = {};
obj.itself = obj
function replacer(key, value) {
if (key === 'itself' ) {
return null
}
return value
}
const stringified = JSON.stringify(obj, replacer)
console.log(stringified)
|
What we’ve done here is use the replacer argument of JSON. Stringify to modify the itself property.
In the replacer function, we check for the key itself, and return the value null for that key. This way, JSON. Stringify replaces the circular reference value with null during stringifying, thereby avoiding infinite stringification.
Share your thoughts in the comments
Please Login to comment...