Gfact | Why accumulate function in C++ gives wrong answer for large numbers?
Last Updated :
21 Sep, 2023
The C++ accumulate() function calculates the sum of numerical values under a certain range. It can also be used to effectively iterate and compute the sum of an entire array or a vector with ease.
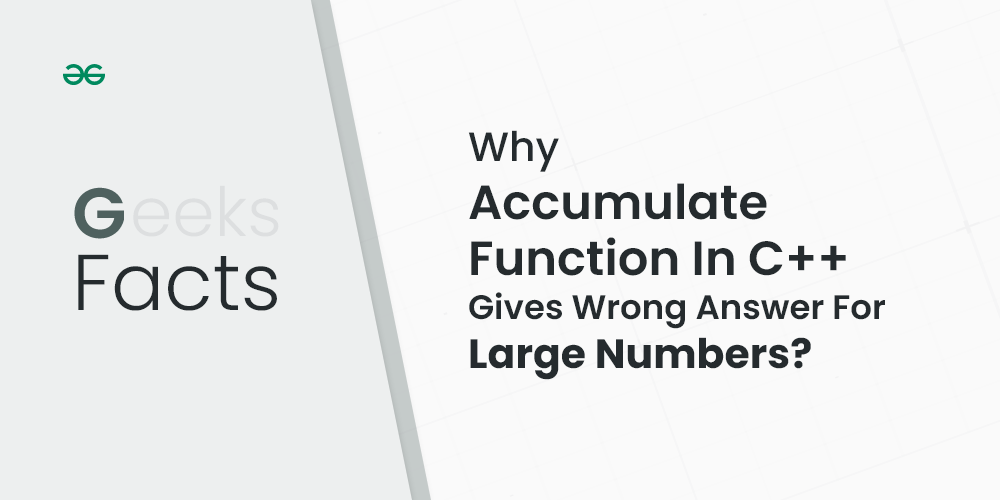
Syntax of C++ accumulate() function:
accumulate(startRange, endRange, initialValue);
Parameters of C++ accumulate() function:
- startRange: specifies the position in the series from which we want the operation to start
- end-range: specifies the position in the series up to which we want to perform the specific operation
- initialValue: the initial value is given to the accumulate() function, it stores the result of the operation in initialValue.
Why accumulate function in C++ gives wrong answer for large numbers?
The reasons why the accumulate function may give wrong answers for large numbers include:
- Data Type Limitations: The data type used for accumulation may have a limited range. When the sum of elements exceeds this range, integer overflow occurs, and the result becomes incorrect.
- Mismatched Data Types: If there is a mismatch between the data type of the initial value provided to accumulate and the data type of elements in the container, it can lead to incorrect results.
- Precision Loss (Floating-Point): When working with floating-point numbers, precision limitations can result in small inaccuracies in the final result.
To work with large numbers without encountering these issues, it’s crucial to choose appropriate data types, ensure consistency in data types, and be mindful of integer overflow and precision concerns.
Code to predict output:
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
long long val = 1000000000000;
vector< long long > v({ val, val, val });
long long sum = accumulate(v.begin(), v.end(), 0);
cout << sum << '\n' ;
return 0;
}
|
Expected Output: 3000000000000
Actual Output : 2112827392
Why this problem occurs?
The issue in your code is due to integer overflow. If the sum of the numbers being accumulated exceeds the maximum value representable by the data type (e.g., int or long long), overflow occurs, and the result becomes incorrect.
- In C++, the accumulate function sums up the elements using the same data type as the elements in the container (in this case, long long), but the initial value you provided, 0, is of type int so the sum also returns as int, as int cannot store large values like 3e12.
- This mismatch in data types can lead to unexpected results, especially when working with large values.
Solution to this issue:
To fix this issue, you should ensure that the initial value provided to accumulate is of the same data type as the elements in the vector (long long in this case).
Here’s the modified code:
C++
#include <bits/stdc++.h>
using namespace std;
int main()
{
long long val = 1e12;
vector< long long > v({ val, val, val });
long long sum
= accumulate(v.begin(), v.end(), ( long long )0);
cout << sum << '\n' ;
return 0;
}
|
Share your thoughts in the comments
Please Login to comment...