When to use an uncontrolled component in React?
Last Updated :
22 Feb, 2024
Uncontrolled Components are the components that are not controlled by the React state or any functions and are handled by the DOM (Document Object Model). So to access any value that has been entered we take the help of refs. In an uncontrolled component, the component manages its state internally. Common use cases for uncontrolled components include simple input fields or elements that don’t need control.
Approach to use Uncontrolled Components in React:
- Create a ref using useRef(): The useRef() hook creates a reference to a DOM element or a value. It returns an object with a current property that holds the reference.
- Attach this ref to the form element: Once you have the ref, attach it to a form element (e.g., input, select, textarea). Use the ref attribute to associate the ref with the element.
- Access the form element’s value: You can now directly interact with the form element using the ref. Access the element’s properties (e.g., value, checked, etc.) via inputRef.current.
Example 1: In this example we are creating a simple form that contains an input field with a label and a submit button with an onSubmit function that triggers when we submit the form. We are accessing the text we have filled in the input section using useRef.
Javascript
import React, { useRef } from 'react'
function App() {
const inputRef = useRef()
const handleSubmit = (event) => {
event.preventDefault()
alert(
`Submitted value:
${inputRef.current.value}
`)
}
return (
<form onSubmit={handleSubmit}>
<label>
Enter something:
</label>
<input type= "text" ref={inputRef} />
<button type= "submit" >Submit</button>
</form>
)
}
export default App
|
Output:
Example 2: In this example there is an input field to take file as an input and a button that triggers a function on click. We are accessing the name of the file we have filled in the input section using useRef.
Javascript
function App() {
const fileInputRef = useRef();
const handleFileUpload = () => {
const selectedFile =
fileInputRef.current.files[0];
alert(
`Submitted value: ${selectedFile.name}`
)
};
return (
<div>
<input type= "file"
ref={fileInputRef} />
<button onClick={handleFileUpload}>
Upload
</button>
</div>
);
}
export default App
|
Output:
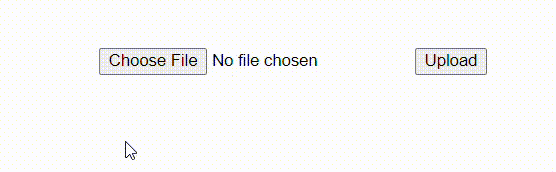
Advantages of using Uncontrolled Components:
- Simplicity and Flexibility: Uncontrolled components are straightforward to use. They don’t require managing state explicitly. You can integrate them easily with existing code or third-party libraries.
- Less Boilerplate Code: Since uncontrolled components don’t rely on React state, you avoid writing extra code for state management. This leads to cleaner and more concise code.
- Direct DOM Interaction: Uncontrolled components directly interact with the DOM, which can be useful for specific scenarios (e.g., file uploads). You can access form values using refs without involving React state.
Disadvantages of Uncontrolled Components:
- Limited Validation: Implementing validation logic becomes more challenging with uncontrolled components since you don’t have direct access to the component’s state.
- Debugging: Debugging issues related to form handling and state can be more complicated with uncontrolled components, as you might need to trace through DOM manipulation or event handling to understand what’s happening.
Share your thoughts in the comments
Please Login to comment...