What will happen if a print() statement is written inside a if() such as if(print())
Last Updated :
13 Sep, 2021
Pre-requisite: if-else
This article focuses on discussing what happens when the print statement is used inside the if-else conditional statement.
For example: Consider the below code and predict the output.
C
#include <stdio.h>
int main()
{
if ( printf ( "I'm Awesome!\n" ))
main();
else if ( printf ( "I'm Not Awesome\n" ))
main();
}
|
C++
#include <iostream>
using namespace std;
int main()
{
if ( printf ( "I'm Awesome!\n" ))
main();
else if ( printf ( "I'm Not Awesome\n" ))
main();
return 0;
}
|
Java
class GFG {
public static void main(String[] args) {
if (System.out.printf( "I'm Awesome!\n" ) != null )
main( null );
else if (System.out.printf( "I'm Not Awesome\n" ) != null )
main( null );
}
}
|
Python3
if ( print ( "I'm Awesome!" )):
def main():
elif ( print ( "I'm Not Awesome" )):
def main():
|
C#
using System;
class GFG {
public static void Main(String[] args) {
if (Console.WriteLine( "I'm Awesome!\n" ))
Main( null );
else if (Console.Write( "I'm Not Awesome\n" ))
Main( null );
}
}
|
Javascript
<script>
function main() {
if (document.write( "I'm Awesome!\n" ))
main();
else if (document.write( "I'm Not Awesome\n" ))
main();
}
main();
</script>
|
Guessing the Expected Output: Most general guess for the output of this program is some sort of ERROR.
Actual Output: But its actual output is a bit strange and not the expected one.
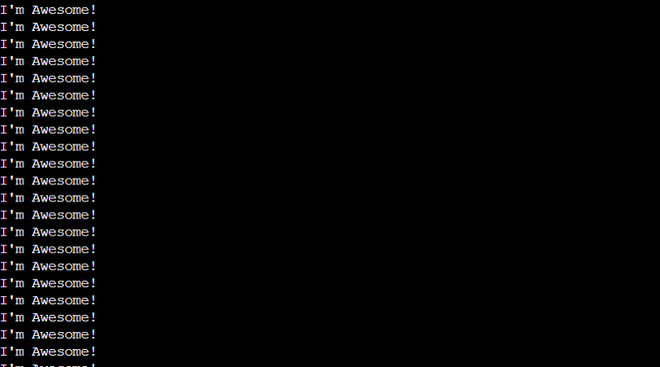
Explanation: Let’s discuss the reason.
- As if condition is being satisfied every time and due to recursive call of the main() function, this function call will ideally go on infinitely but in the real scenario, it’ll stop once the allowed function call stack will be filled.
- So, first of all, let’s understand why the print function inside if condition is not giving error and how it’s working internally.
- This behavior is based on a simple fact that print function like printf in C/C++ etc returns an integer value equal to the number of characters it has printed successfully.
Let’s understand this by a program below-
C
#include <stdio.h>
int main()
{
printf ( "%d" , printf ( "GFG!\n" ));
return 0;
}
|
C++
#include <iostream>
using namespace std;
int main()
{
printf ( "%d" , printf ( "GFG!\n" ));
return 0;
}
|
Java
import java.util.*;
class GFG{
public static void main(String[] args)
{
System.out.printf( "%s" , System.out.printf( "GFG!\n" ));
}
}
|
Python3
C#
using System;
class GFG{
public static void Main(String[] args)
{
Console.Write( "GFG!\n" );
}
}
|
Javascript
<script>
document.write( "GFG!\n" );
</script>
|
Explanation: When the printf statement is encountered it goes to the outer printf function and tries to print its value and when the program tries to print its value then it encounters another printf and it goes to inner printf (like a recursive call) to execute it and then it executes it and that inner printf function returns 5 because that function has successfully printed 5 characters (‘G’, ‘F’, ‘G’, ‘!’, and ‘\n’) and hence return an integer value equal to 5 in this case.
Will print() used inside if always return true?
From the above example, it can be easily understood how printf functions are working inside if statements and how the condition is being satisfied. The condition will always satisfy until and unless an empty string is printed like as shown in the below code-
The only idea behind this example is to demonstrate if the printf function returns “0” only then the if condition would turn out to be false. In all other cases, it’ll be true whether the length of the string is 1 or 9999.
C
#include <stdio.h>
int main()
{
if ( printf ( "" ))
printf ( "Condition is True" );
else
printf ( "Condition is False" );
return 0;
}
|
C++
#include <iostream>
using namespace std;
int main()
{
if ( printf ( "" ))
printf ( "Condition is True" );
else
printf ( "Condition is False" );
return 0;
}
|
Java
import java.io.*;
class GFG
{
public static void main (String[] args)
{
if (System.out.print( "" ))
System.out.print( "Condition is True" );
else
System.out.print( "Condition is False" );
}
}
|
Python3
if ( print ("")):
print ( "Condition is True" )
else :
print ( "Condition is False" )
|
C#
using System;
class GFG
{
public static void Main (String[] args)
{
if (Console.Write( "" ))
Console.Write( "Condition is True" );
else
Console.Write( "Condition is False" );
}
}
|
Javascript
<script>
if (document.write( "" ))
document.write( "Condition is True" );
else
document.write( "Condition is False" );
</script>
|
Share your thoughts in the comments
Please Login to comment...