What is the purpose of callback function as an argument of setState event ?
Last Updated :
03 Nov, 2023
In React, the purpose of callback function as an argument of setState event is to execute the code after the data in the state is modified.
Purpose of callback function as an argument of setState
SetState is an asynchronous method. Asynchronous means that the remaining code will get executed while the current action is being performed. Whereas synchronous code will block the code execution while the current action is being performed. So, if you want an action to be performed only after the state has been updated you can make use of a call back function. This callback function is put as an argument to setState the method. This is the exact purpose of defining a callback function as an argument of setState.
Prerequisites:
Approach:
The callback function is passed as an argument in setState to execute the code immediately after the setState has completed the updation. The callback function will log the updated value on the console when the asynchronous action is completed.
Steps to create React Application:
Step 1: Create a react application by typing the following command in the terminal.
npx create-react-app project_name
Step 2: Now, go to the project folder i.e. project_name by running the following command.
cd project_nam
Project Structure:
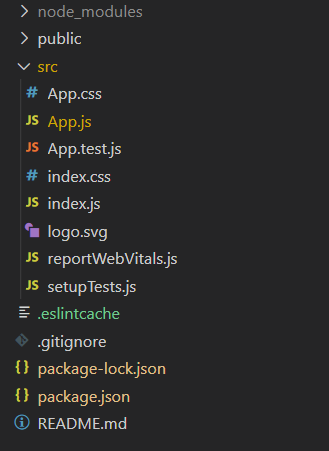
Project Structure
Example: This example uses callback function to asynchronously log the updated value on console immediately after the state is updated.
Javascript
import React from "react" ;
class App extends React.Component {
constructor() {
super ();
this .state = {
value: 0,
};
}
call() {
this .setState({ value: this .state.value + 1 }, () =>
console.log(
"Updated Value :" + this .state.value
)
);
}
render() {
return (
<div
style={{
textAlign: "center" ,
margin: "auto" ,
}}
>
<h1 style={{ color: "green" }}>
GeeksforGeeks
</h1>
<h3>
React Example for callback in setState
</h3>
<h4>Current state: { this .state.value}</h4>
<button
onClick={() => {
this .call();
}}
>
Click to update state!
</button>
</div>
);
}
}
export default App;
|
Step to Run Application: Run the application using the following command from the root directory of the project:
npm start
Output:This output will be visible on http://localhost:3000/ on the browser window.
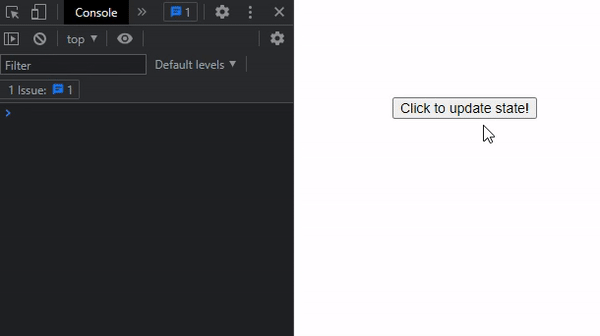
Set State with callback
Share your thoughts in the comments
Please Login to comment...