What is ‘pathmatch: full’ and what effect does it have in Angular?
Last Updated :
23 Jan, 2024
Routing in Angular allows the users to create a single-page application with multiple views and allows navigation between them. Users can switch between these views without losing the application state and properties.
pathmatch:full
In Angular, pathMatch: full is a configuration option used in route definitions to specify how the Angular Router should match a URL to a route. This configuration is part of the Route object in the Angular Router configuration.
When pathMatch is set to full, it means that Angular should only consider the route to be a match if the URL is equal to the specified path. In other words, the full path must match exactly for the route to be activated.
Steps for Installing & Configuring the Angular Application
Step 1: Create an Angular application using the following command.
ng new appname
Step 2: After creating your project folder i.e. appname, move to it using the following command.
cd appname
Step 3: Create the Home and About components. Go to src/app and execute commands in the terminal
ng g c home
ng g c about
Project Structure
It will look like the following:
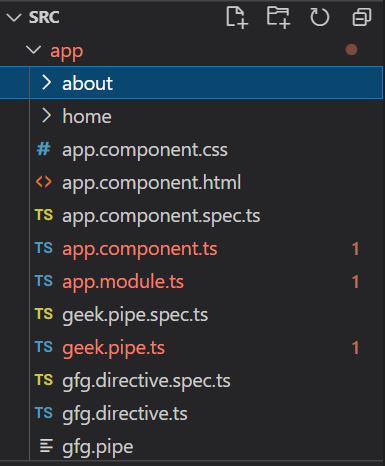
Example: Here, the /home will activate the HomeComponent. /about will activate the AboutComponent. An empty path (”) will redirect to /home because of the redirectTo: ‘/home’ configuration. The pathMatch: ‘full’ ensures that only an exact empty path match triggers the redirection.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >
What is 'pathmatch: full' and
what effect does it have in Angular?
</ h2 >
< nav >
< a routerLink = "/home"
pathMatch = "full" >Home
</ a >
< br >
< a routerLink = "/about"
pathMatch = "full" >About
</ a >
</ nav >
< router-outlet ></ router-outlet >
|
HTML
< h3 style = "color: indianred;" >
Hi Geek!! Please vote for me for
Geeks Premier League
</ h3 >
|
HTML
< h3 style = "color:violet" >
Hi Geek!! Use the like button to vote
</ h3 >
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule }
from '@angular/core' ;
import { AppComponent }
from './app.component' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { RouterModule, Routes }
from '@angular/router' ;
import { HomeComponent }
from './home/home.component' ;
import { AboutComponent }
from './about/about.component' ;
const routes: Routes = [
{ path: 'about' , component: AboutComponent },
{ path: 'home' , component: HomeComponent },
{ path: '' , redirectTo: '/home' , pathMatch: 'full' },
];
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent
],
imports: [
BrowserModule,
RouterModule.forRoot(routes)
]
})
export class AppModule { }
|
Output:
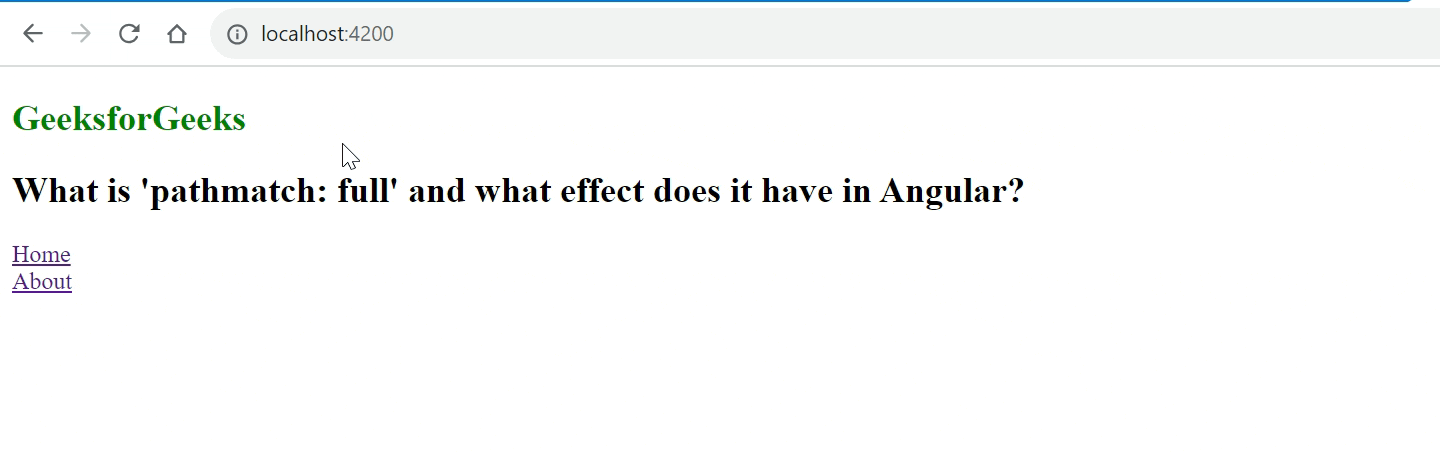
What effect will be there if we don’t use pathmatch:full ?
Without pathMatch: full, even a URL like /home/something could match the empty path and redirect to /home. Adding pathMatch: full ensures that only an exact match triggers the redirect.
Example: In this case, without pathMatch: ‘full’, an empty path (”) would match any URL that starts with an empty path. By default value of pathMatch is ‘prefix’. For example; /home would still activate the HomeComponent, /about would activate the AboutComponent, and/something would also redirect to /home, even though it doesn’t exactly match the empty path.
HTML
< h2 style = "color: green;" >GeeksforGeeks</ h2 >
< h2 >
What is 'pathmatch: full' and
what effect does it have in Angular?
</ h2 >
< nav >
< a routerLink = "/home" >Home</ a >
< br >
< a routerLink = "/about" >About</ a >
</ nav >
< router-outlet ></ router-outlet >
|
HTML
< h3 style = "color:violet" >
Hi Geek!! Use the like button to vote
</ h3 >
|
HTML
< h3 style = "color: indianred;" >
Hi Geek!! Please vote for me for
Geeks Premier League
</ h3 >
|
Javascript
import { Component } from '@angular/core' ;
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styleUrls: [ './app.component.css' ]
})
export class AppComponent { }
|
Javascript
import { NgModule }
from '@angular/core' ;
import { AppComponent }
from './app.component' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { RouterModule, Routes }
from '@angular/router' ;
import { HomeComponent }
from './home/home.component' ;
import { AboutComponent }
from './about/about.component' ;
const routes: Routes = [
{ path: 'about' ,
component: AboutComponent },
{ path: 'home' ,
component: HomeComponent },
{ path: '' , redirectTo: '/home' ,
pathMatch: 'prefix' },
];
@NgModule({
bootstrap: [
AppComponent
],
declarations: [
AppComponent
],
imports: [
BrowserModule,
RouterModule.forRoot(routes)
]
})
export class AppModule { }
|
Output:
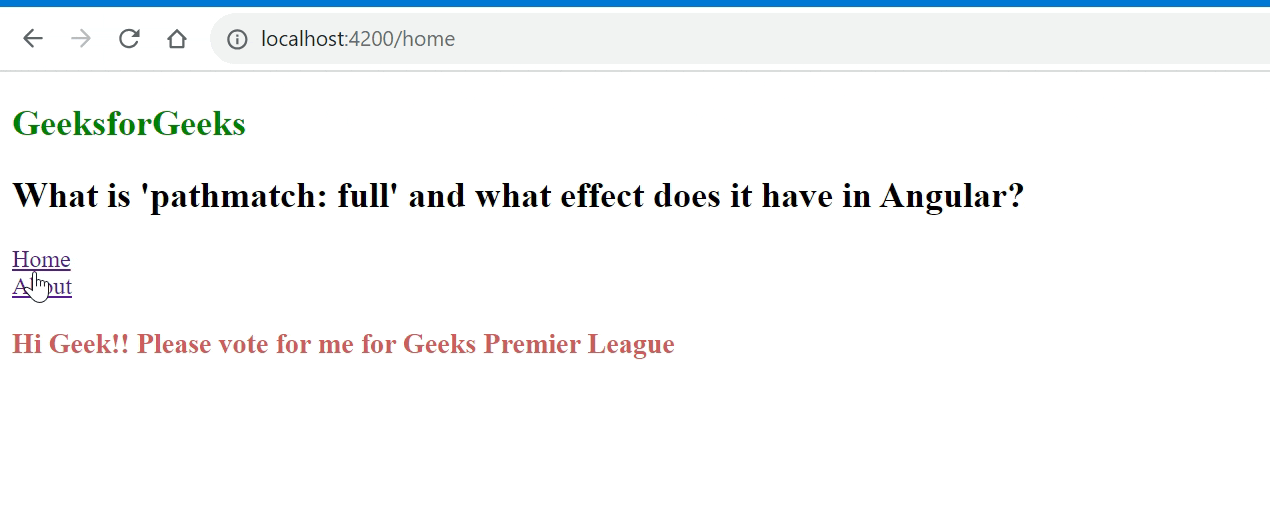
Conclusion
Using pathMatch: ‘full’ ensures that only an exact empty path match triggers the redirect, providing more precise control over route matching. When pathMatch is set to full, it means that Angular should only consider the route to be a match if the URL is equal to the specified path. In other words, the full path must match exactly for the route to be activated.
Share your thoughts in the comments
Please Login to comment...