What is onerror() Method in JavaScript?
Last Updated :
18 Apr, 2024
The onerror() method in JavaScript is an event for error handling that occurs during the execution of code. It provides the mechanism to capture and handle runtime errors ensuring graceful error handling and debugging in the web applications.
You can implement the onerror() method using the below methods:
Using Inline Event Handler
In this approach, we attach the onerror() event handler directly to the specific elements or objects in the HTML markup as an attribute.
Syntax:
<HTMLElement onerror="eventHandlerMethod()">
// Element Content
</HTMLElement>
Example: The below code will explain how you can attach onerror method using inline event handler.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
The onerror() Method Example
</title>
</head>
<body style="text-align: center;">
<h2>
Click the below button to remove
the src of image <br> to trigger the
onerror() event method.
</h2>
<img onerror="handleError()" src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240401152503/javascript.jpg">
<br><br>
<button onclick="removeSrc()">
Remove Image src
</button>
<script>
const image = document.querySelector('img');
function removeSrc(){
image.setAttribute('src', "");
}
function handleError() {
alert('Error loading image!');
}
</script>
</body>
</html>
Output:
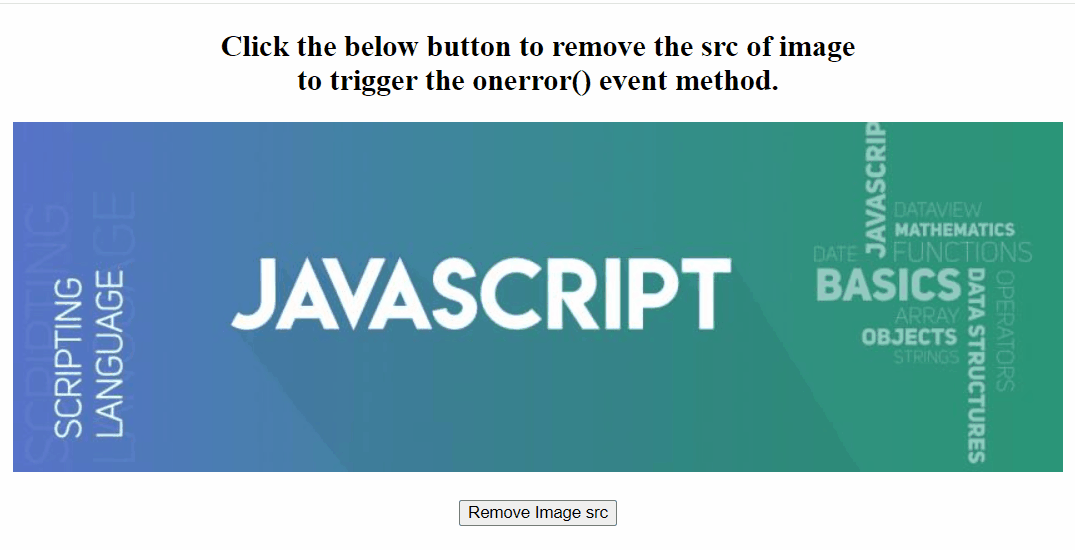
Using addEventListener() method
This approach involves attaching an event listener to the target element using addEventListener() method.
Syntax:
document.getElementById('image').addEventListener('error', handleError);
Example: The below code explains the use of addEventListener() method to attach the onerror() event.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content=
"width=device-width, initial-scale=1.0">
<title>
The onerror() Method Example
</title>
</head>
<body style="text-align: center;">
<h2>
Click the below button to remove
the src of image <br> and trigger the
onerror() event method.
</h2>
<img src=
"https://media.geeksforgeeks.org/wp-content/uploads/20240401152503/javascript.jpg">
<br><br>
<button>
Remove Image src
</button>
<script>
const image = document.querySelector('img');
const btn = document.querySelector('button');
image.addEventListener('error', handleError);
btn.addEventListener('click', removeSrc);
function removeSrc(){
image.setAttribute('src', "");
}
function handleError() {
alert('Error loading image!');
}
</script>
</body>
</html>
Output:
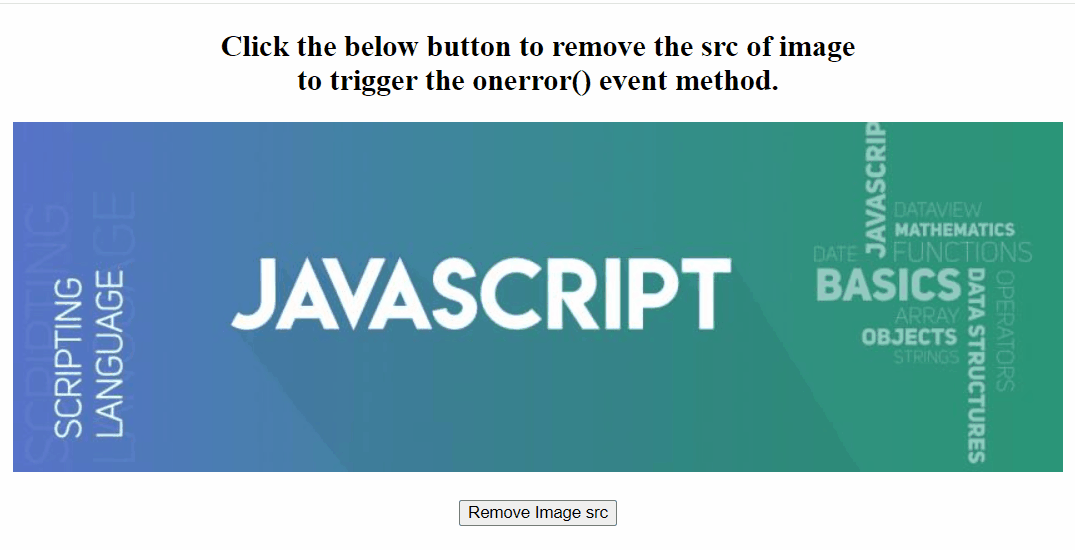
Share your thoughts in the comments
Please Login to comment...