What does <%= mean in EJS Template Engine ?
Last Updated :
06 Mar, 2024
EJS or Embedded Javascript Templating is a templating engine used by Node.js. Template engine helps to create an HTML template with minimal code. Also, it can inject data into an HTML template on the client side and produce the final HTML. EJS is a simple templating language that is used to generate HTML markup with plain JavaScript. It also helps to embed JavaScript into HTML pages.Â
What is <%= %> in EJS?
In EJS, the <%= %>
tag is used to embed JavaScript code that evaluates to a value, which is then inserted into the rendered HTML output. This tag is commonly referred to as the “output” tag in EJS, as it outputs the result of the JavaScript expression to the HTML document.
Syntax of <%= %> in EJS:
<p>Hello, <%= username %></p>
The JavaScript expression enclosed within the <%= %>
tags can be any valid JavaScript code. This value will be inserted into the HTML output when the EJS template is rendered.
Working of <%= %> tag in EJS
When the EJS template is rendered by the server-side code, the <%= %>
tags are processed, and the JavaScript expressions within them are executed. The result of the expression is then concatenated into the final HTML output sent to the client’s browser.
Key features and Benefits of EJS tag:
- <%= %> tags are used to embed dynamic content or variables within HTML templates or code.
- They induce the interpolation of values from server-side variables or expressions into the HTML or template.
- The content inside <%= %> is \an expression that gets evaluated, and the result is inserted into the template.
- It allows for the creation of dynamic and data-driven web pages by injecting server-side data into the client-side page.
- Enables the reuse of code snippets or dynamic values across different parts of a web application.
Node
import express from "express";
import bodyParser from "body-parser";
import axios from "axios";
const ejs = require("ejs");
const app = express();
const port = 3000;
const API_URL = "http://localhost:4000";
app.use(express.static("public"));
app.use(bodyParser.urlencoded({ extended: true }));
app.use(bodyParser.json());
// Route to render the main page
app.get("/", async (req, res) => {
try {
const response = await axios.get(`${API_URL}/posts`);
console.log(response);
res.render("home.ejs", { posts: response.data });
} catch (error) {
res.status(500).json({ message: "Error fetching posts" });
}
});
In the EJS file , home.ejs, deliver the date and content of the JSON response as an output to the dynamic web page by making use of the ejs tag <%=. We use them under the try catch block to display the error message and to check whether what had went wrong while fetching and rendering the response.
Steps to create EJS Apllication :
Step 1: Initialize the Node application by running the following command..
npm init -y
Step 2: Install the required dependencies:
npm install ejs
Folder structure:
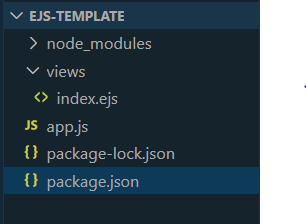
The updated dependencies in package.json file will look like:
"dependencies": {
"ejs": "^3.1.9",
"express": "^4.18.3"
}
Code Example: Create views folder and the following code.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Welcome Page</ title >
</ head >
< body >
< h1 >Welcome, <%= username %>!</ h1 >
</ body >
</ html >
|
Javascript
const express = require( 'express' );
const app = express();
const port = 3000;
app.set( 'view engine' , 'ejs' );
app.get( '/' , (req, res) => {
res.render( 'index' , { username: 'John' });
});
app.listen(port, () => {
console.log(`Server is running on http:
});
|
Output:

Output
Share your thoughts in the comments
Please Login to comment...