What are the difference between Vuex store and Plain Global Object ?
Last Updated :
25 Sep, 2023
While working with JavaScript projects or applications, managing the application’s state properly becomes very important. This crucial work is been handled by Vuex Store and Plain Global Object. Both these methods aim to properly handle the state of the application, but they differ in some of the parameters. In this article, we will go through a detailed explanation of Vuex Store and Plain Global Object along with their example and lastly, we will explore the difference between these 2 approaches with unique and important parameters.
Vuex Store
Vuex Store in Vue.js is basically a state management module and library designed for Vue.js applications. This library offers a centralized store to properly manage the application’s behavior and state. We can properly manage and modularize the state logic efficiently. The Vuex Store is reactive, which means that any certain changes done to the state trigger updates throughout the entire application. Vuex Store has built-in mechanisms like a getter to modify and access the state in application and mutations. Vuex is most probably designed to integrate seamlessly with the Vue.js framework, which makes it a fitness suite for Vue.js framework projects and applications.
Steps to install a Vue App:
Step 1: Install Vue modules using the below npm command
npm install vue
Step 2: Use Vue JS through CLI. Open your terminal or command prompt and run the below command.
npm install --global vue-cli
Step 3: To use the Vuex, the following command will be used
npm install vuex@next --save
Step 4: Create the new project using the below command
vue init webpack myproject
Step 5: Change the directory to the newly created project
cd myproject
Project Structure:
The following project structure will be generated:
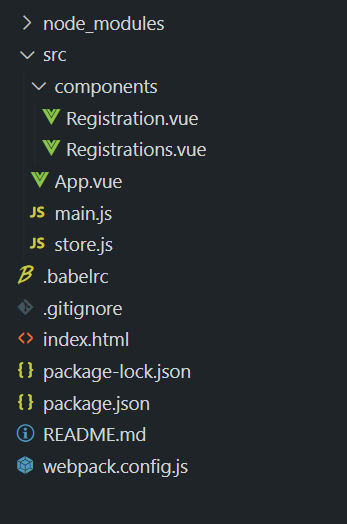
Example: Below is the example of the Vuex Store along with the Code Snippet and its Output.
HTML
< template >
< div id = "registration" >
< h3 >Register here</ h3 >
< hr >
< div class = "row"
v-for = "user in users" >
< h4 >{{ user.name }}</ h4 >
< button @ click = "registerUser(user)" >
Register
</ button >
</ div >
</ div >
</ template >
< script >
export default {
computed: {
users() {
return this.$store.state.users.filter(user => {
return !user.registered;
});
}
},
methods: {
registerUser(user) {
const date = new Date;
user.registered = true;
this.$store.state.registrations.push(
{userId: user.id,
name: user.name,
date: date.getMonth()+
'/' + date.getDay()});
}
}
}
</ script >
< style scoped>
#registration {
box-shadow: 1px 1px 2px 1px #ccc;
margin: 20px;
padding: 20px;
display: inline-block;
width: 300px;
vertical-align: top;
}
.row h4 {
display: inline-block;
width: 70%;
text-align: left;
margin: 0 0 10px 0;
}
button {
background-color: lightgreen;
border: none;
box-shadow: 1px 1px 1px black;
font-size: inherit;
text-align: right;
cursor: pointer;
}
button:hover {
background-color: green;
}
</ style >
|
HTML
< template >
< div id = "registrations" >
< div class = "summary" >
< h3 >Registrations</ h3 >
< h5 >Total: {{ total }}</ h5 >
</ div >
< hr >
< div class = "row"
v-for = "registration in registrations" >
< h4 >{{ registration.name }}</ h4 >
< span @ click = "unregister(registration)" >
(Unregister)
</ span >
< div class = "date" >
{{ registration.date }}
</ div >
</ div >
</ div >
</ template >
< script >
export default {
methods: {
unregister(registration) {
const user =
this.$store.state.users.find(user => {
return user.id == registration.userId;
});
user.registered = false;
this.$store.state.registrations.splice(
this.$store.state.registrations.indexOf(registration), 1);
}
},
computed: {
registrations() {
return this.$store.state.registrations;
},
total() {
return this.$store.state.registrations.length;
}
}
}
</ script >
< style scoped>
#registrations {
box-shadow: 1px 1px 2px 1px #ccc;
margin: 20px;
padding: 20px;
display: inline-block;
width: 300px;
vertical-align: top;
text-align: left;
}
.summary {
text-align: center;
}
.row h4 {
display: inline-block;
width: 30%;
margin: 0 0 10px 0;
box-sizing: border-box;
}
.row span {
width: 30%;
color: red;
cursor: pointer;
}
.row span:hover {
color: darkred;
}
.date {
display: inline-block;
width: 38%;
text-align: right;
box-sizing: border-box;
}
</ style >
|
HTML
< template >
< div id = "app" >
< app-registration ></ app-registration >
< app-registrations ></ app-registrations >
</ div >
</ template >
< script >
import Registration
from './components/Registration.vue';
import Registrations
from './components/Registrations.vue';
export default {
components: {
appRegistration: Registration,
appRegistrations: Registrations
}
}
</ script >
< style >
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
margin-top: 60px;
}
</ style >
|
Javascript
import Vue from 'vue'
import App from './App.vue'
import { store } from './store' ;
new Vue({
el: '#app' ,
store,
render: h => h(App)
})
|
Javascript
import Vue from 'vue' ;
import Vuex from 'vuex' ;
Vue.use(Vuex);
export const store = new Vuex.Store({
state: {
registrations: [],
users: [
{id: 1, name: 'Gaurav' , registered: false },
{id: 2, name: 'Anjali' , registered: false },
{id: 3, name: 'Ramesh' , registered: false },
{id: 4, name: 'GeeksforGeeks' , registered: false }
]
}
});
|
Explanation: The Vuex store in this example tracks the list of registered users and the list of all users. The unregister method is used to remove a user from the list of registered users, and the total computed property returns the number of registrations.
Output:
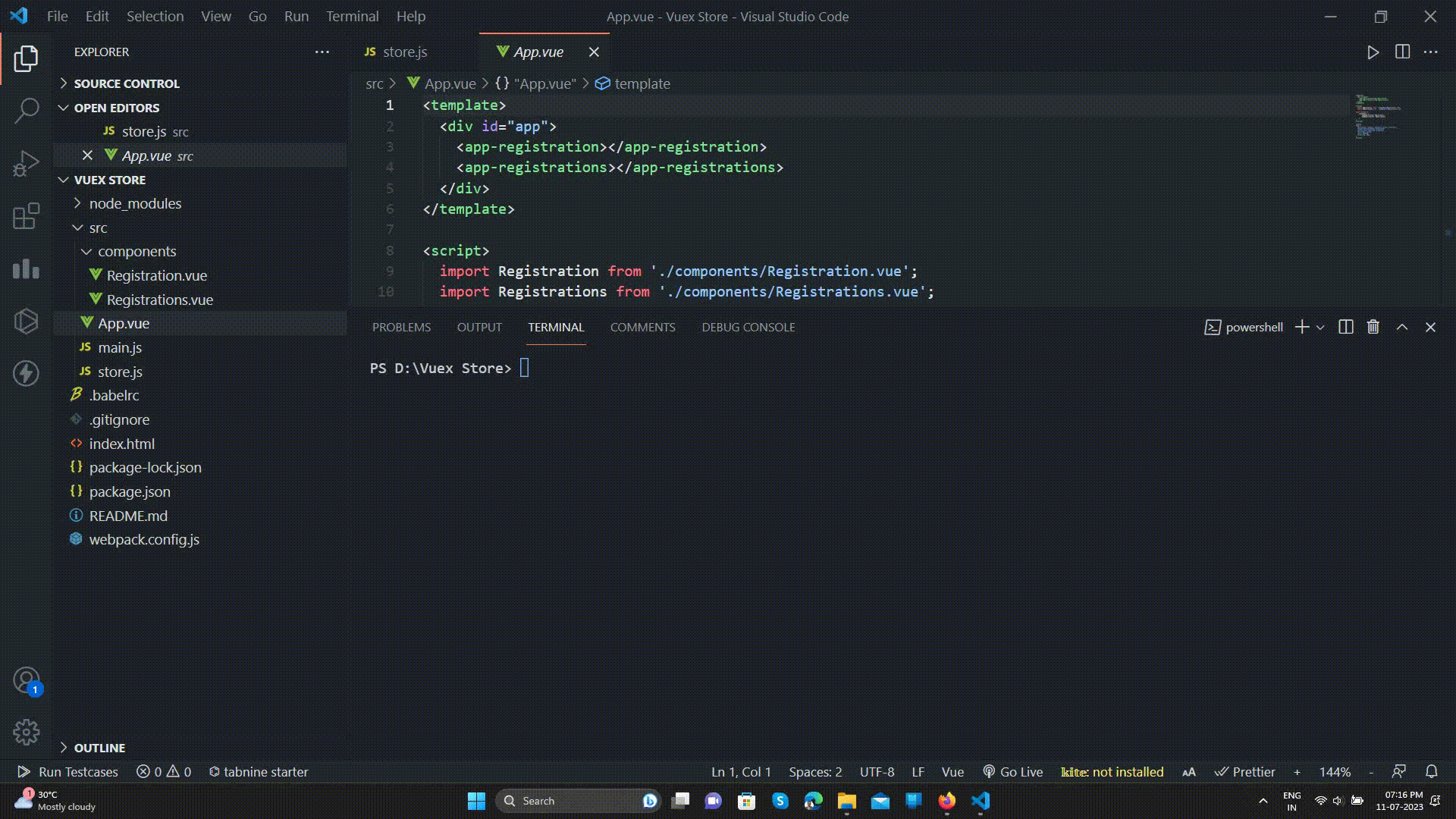
Plain Global Object
Plain Global Object points to the use of Global JavaScript Object which we used to store and manage the application’s state. As compared to Vuex, Plain Global Object does not offer any built-in state management mechanisms. Perhaps, we can manually define a global object that can handle changes to it. However, Global Object does not trigger on its own and updates the application. We need to handle updates and make sure about consistency manually. Managing complex states and organizing the code can be challenging tasks while working with Plain Global Objects.
Example: Below is the example of the Plain Global Object along with the Code Snippet and the dedicated Output.
Javascript
const cart = {
items: [],
addItem(item) {
this .items.push(item);
},
removeItem(itemId) {
this .items =
this .items.filter(item => item.id !== itemId);
},
getCartTotal() {
return this .items.reduce((total, item) =>
total + item.price, 0);
},
clearCart() {
this .items = [];
}
};
cart.addItem({ id: 1, name: 'GFG_DSA' , price: 10 });
cart.addItem({ id: 2, name: 'GFG_C++' , price: 20 });
{ id: 2, name: 'Product 2' , price: 20 }]
console.log(cart.items);
console.log(cart.getCartTotal());
cart.removeItem(1);
console.log(cart.items);
console.log(cart.getCartTotal());
cart.clearCart();
console.log(cart.items);
console.log(cart.getCartTotal());
|
Explanation: The above examples show the states of the plain global object called ‘cart‘ and the calculated total after each purchase. The ‘cart‘ object defines a shipping cart and has the properties and methods to manage it. After adding 2 items to the cart.getCartTotal() returns 30. After removing an item from the cart, it returns 20. After clearing the cart, the cart becomes empty and returns 0.
Output
[
{ id: 1, name: 'GFG_DSA', price: 10 },
{ id: 2, name: 'GFG_C++', price: 20 }
]
30
[ { id: 2, name: 'GFG_C++', price: 20 } ]
20
[]
0
Differences between Vuex Store & Plain Global Object
Parameter
|
Vuex Store
|
Plain Global Object
|
Purpose |
Vuex Store is used as a State Management Library for Vue.js applications. |
Plain Global Object is used for State Storage. |
Centralized Store |
There is a Centralised Store in the Vuex Store. |
There is no Centralised Store in Plain Global Object. |
Built-in Mechanisms |
There are built-in mechanisms like mutation, actions, and getters. |
No built-in mechanisms support is been seen here. |
Testing |
Vuex Store supports testing using Vue.js testing utilities. |
In Plain Global Object, testing is implemented manually. |
Code Organization |
Vuex Store offers structure and modularization for state logic in code. |
In Plain Global Object, the structure and organization of code are done manually. |
Dependency |
Prerequisites: Vue.js Framework |
Prerequisites: Framework-Indepeneent, can be used in a JS Project. |
Share your thoughts in the comments
Please Login to comment...