How to Watch Store Values from Vuex ?
Last Updated :
10 Jan, 2024
VueJS is one of the best frameworks for JavaScript like ReactJS. The VueJS is used to design the user interface layer. It is compatible with other libraries and extensions as well. In this article, we will learn How to watch store values from Vuex.
Approach 1: Using Vue options
In this approach, we’re directly using Vue options like data, computed, and methods to set up the component and watch the store values in Vuex.
Example: The below code example implements the Vue options to watch the store values from Vuex.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >GFG</ title >
</ head >
< body >
< div id = "app" >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
How to watch store
values from vuex?
</ h2 >
< h1 >
Counter (Approach 1):
{{ counter }}
</ h1 >
< button @ click = "increment" >
Increment
</ button >
</ div >
< script src =
</ script >
< script src =
</ script >
< script >
// Vuex Store
const store = new Vuex.Store({
state: {
counter: 0,
},
mutations: {
increment(state) {
state.counter++;
},
},
});
// Vue Instance
new Vue({
el: '#app',
store,
data() {
return {};
},
computed: {
counter() {
return this.$store.
state.counter;
},
},
methods: {
increment() {
this.$store.
commit('increment');
},
},
});
</ script >
</ body >
</ html >
|
Output:
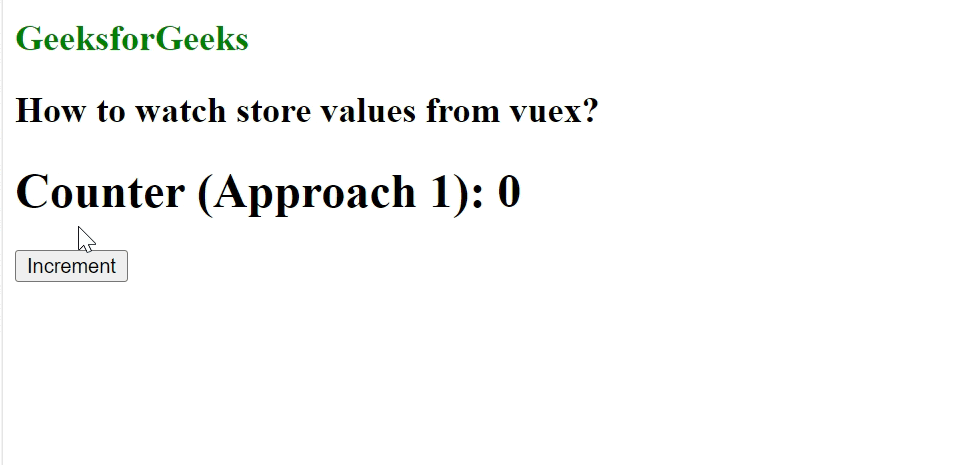
Approach 2: Using Components
In this approach, we encapsulate the counter functionality into a separate Vue component (counter-component) to promote code modularity and watch the store values from Vuex.
Example: The below example implements the components approach to watch the store values from Vuex.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content=" width = device -width,
initial-scale = 1 .0">
< title >GFG</ title >
</ head >
< body >
< div id = "app" >
< counter-component />
</ div >
< script src =
</ script >
< script src =
</ script >
< script >
// Vuex Store
const store = new Vuex.Store({
state: {
counter: 0,
},
mutations: {
increment(state) {
state.counter++;
},
},
});
// Counter Component
Vue.component('counter-component', {
template: `
< div >
< h2 style = "color: green;" >
GeeksforGeeks
</ h2 >
< h2 >
How to watch store
values from vuex?
</ h2 >
< h1 >
Counter (Approach 2):
{{ counter }}
</ h1 >
< button @ click = "increment" >
Increment
</ button >
</ div >
`,
computed: {
counter() {
return this.$store.
state.counter;
},
},
methods: {
increment() {
this.$store.
commit('increment');
},
},
});
// Vue Instance
new Vue({
el: '#app',
store,
});
</ script >
</ body >
</ html >
|
Output:
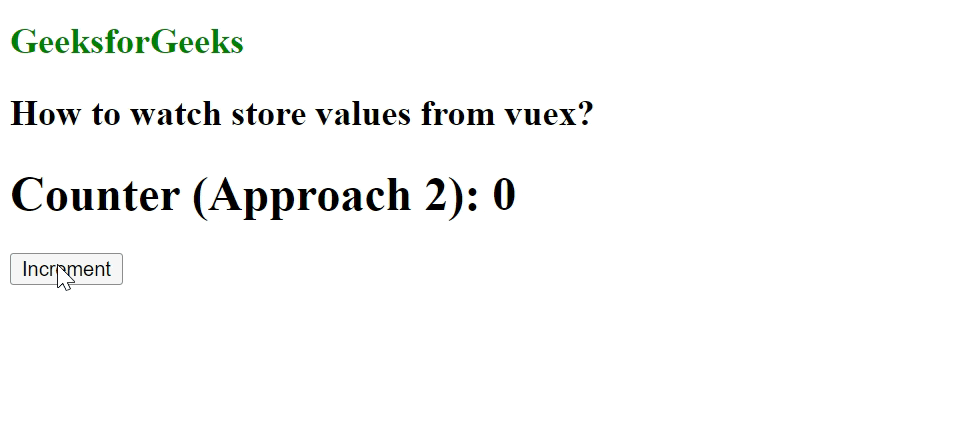
Conclusion
In this article , we will learnt about different approaches of How to watch store values from vuex. Both approaches achieve the same result—displaying a counter and allowing it to be incremented using Vuex. Choose the approach that aligns with your application structure and organization preferences.
Share your thoughts in the comments
Please Login to comment...