What are Class Components in React?
Last Updated :
04 Mar, 2024
React class components are the bread and butter of most modern web apps built in ReactJS. These components are simple classes (made up of multiple functions that add functionality to the application) and are based on the traditional class-based approach. All class components are child classes for the Class Component of ReactJS.
Class Components Features:
- The older approach in React.
- Manages state using this.state and lifecycle methods.
- Tends to be more verbose and requires more code.
- Accesses props and state using this keyword.
Advantages of class components:
- State Access: Class components have access to a state that determines the current behavior and appearance of the component.
- setState() Function: The state in class components can be modified using the
setState()
function.
- Multiple Modifications: One or more variables, arrays, or objects defined in the state can be modified simultaneously using the
setState()
function.
Steps to Create Class Components in React And Installing Module:
Step 1: Create a React application using the following command:
npx create-react-app foldername
Step 2: After creating your project folder i.e. foldername, move to it using the following command:
cd foldername
Step 3: Install the necessary package in your application using the following command.
npm install bootstrap
Project Structure:
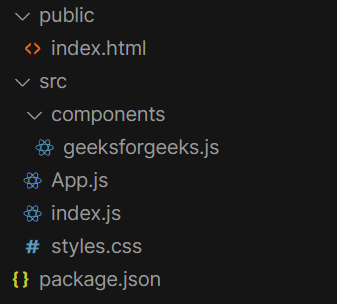
Example 1: Below is an example of class components in React.
Javascript
import React from "react" ;
class GeeksforGeeks extends React.Component {
render() {
return (
<div>
<h2>What are Class Components in React?</h2>
<p>GeeksforGeeks is a computer science portal for geeks.</p>
</div>
);
}
}
export default function App() {
return (
<div className= "App" >
<h2 style={{ color: "green" }}>GeeksforGeeks</h2>
<GeeksforGeeks />
</div>
);
}
|
Step to run the app:
npm start
Output:
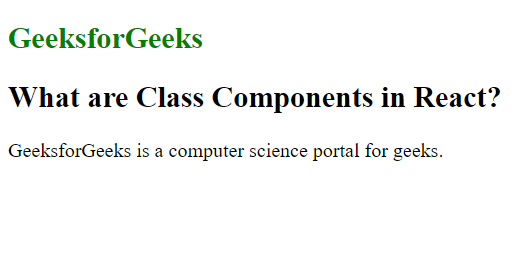
Output
Example 2: In this example, we will see multiple class components, You need to install bootstrap to see the button color.
Javascript
import React from "react" ;
class GeeksforGeeks extends React.Component {
render() {
return (
<div>
<h2>How to create a Class component in React?</h2>
<p>GeeksforGeeks is a computer science portal for geeks.</p>
</div>
);
}
}
class GeeksforGeeks2 extends React.Component {
render() {
return (
<center>
<div style={{ backgroundColor: "yellow" }}>
<button className= "btn btn-primary" >
{ " " }
I am coming from another class component
</button>
</div>
</center>
);
}
}
export default function App() {
return (
<div className= "App" >
<h2 style={{ color: "green" }}>GeeksforGeeks</h2>
<GeeksforGeeks />
<GeeksforGeeks2 />
</div>
);
}
|
Output:

Share your thoughts in the comments
Please Login to comment...