Web API Mutation Observer
Last Updated :
06 Oct, 2023
Mutation Observer is a web API (web APIs are APIs provided by the browser itself) that is used to observe (i.e.; detect) the changes of the target HTML element within a web page. For example, when the user wants to know which element has recently been removed and which element has been added to the DOM, we can use Mutation Observer. In dynamic websites, we know that elements are added, removed, or modified dynamically, this API serves as a tool for tracking and reacting to these changes. In this article, we will see the Mutation Observer in the Web API, along with understanding its benefits, use cases, instance methods, & etc.
Syntax
// Create observer API instance
observer=new MutationObserver( intersectionCallback, config );
// Define intersectionCallback() function to
// do operations when mutation happens.
function intersectionCallback( entries, observer )
// Call observe() method to observe the target HTML element.
observer.observe( targetElement );
Benefits of Observer API
- Mutation observers work asynchronously, so they don’t block the main thread of JavaScript and keep the website responsive/performing for user interactions.
- This API provides information about the mutated elements that helps us to observe the element in an efficient manner.
- Observing multiple elements parallelly is possible with the help of this API so it makes the code clean and more useful for larger applications.
- Event listener’s callback functions are triggered when an event occurs, so their triggering depends on the occurrence of events, but in the case of mutation observer, we can detect changes without the occurrence of events.
- Different types of mutations of the same element or of different elements can be detected at the same time. This can’t be possible without the help of other methods.
Now we know why mutation observer is a more convenient way of observing mutations in the DOM elements, let’s know about its use cases before diving deeper into real-world examples of detecting a DOM mutation.
Use cases of Observeation API
- Detect the presence of specific elements (e.g.; add banners ) and take action if they are removed by ad blockers.
- detect any type of DOM modifications
- Used to implement auto-save functionality in online code editors (e.g.’; Leetcode, Hackerrank, GFG, etc).
- Detect UI updates of multiple elements on real real-time basis.
- Monitor input field for changes and validate user input in real-time, showing them error messages and feedback if required.
- Besides the above-mentioned use cases, limitless new functionalities can be implemented with the help of mutation observers.
Instance Methods
- MutationCallback Function: It is the callback function that is called automatically when a mutation of the target elements happens. It receives 2 parameters: first is a list of all the MutationObserverEntry objects of all the targeted elements that are to be observed. The MutationObserverEntry object has multiple properties to check whether mutations happened or not.
- Config: It tells the observer what we want to observe. The following options can be provided within the config parameter.
- childList:true -> This parameter is used to observe changes in the target or target’s direct children only. If True is set, then the callback function be triggered when nodes are added, updated or removed from the target or its direct children only.
- subtree: true -> used to detect changes in the entire subtree of the target element.
- attributes: true -> used to detect changes in the target element’s specified attributes.
- attributeFilter: [array of attributes] -> used to observe mutation only when the specified attributes of the target element change.
- characterData: true -> used to observe mutations in textContent, innerHTML, innerText, or nodeValue of the target element.
- observe() method: when the observe method is invoked, mutation observer API starts monitoring mutations of the target element.
- entries: It is an array of MutationObserverEntry objects maintained by observer API for each targeted element. MutationObserverEntry object holds useful information about all of the targeted elements whether they are mutating or not.
Some possible approaches to use Mutation Observer API are:
Detecting style change in target HTML element
We have a single <div> element (our target ). Initially, the color of this <div> is red, then we are going to change its color from red to blue and then blue to green in the intervals of 4 sec and 6 sec. So our objective is to detect the moment when mutation of colors happens using mutation observer API.
Example: First we will change the color of the target within the specified interval using the SetTimeout() function. Now to detect the change, we will use attributes and attributeFilter property of the config option object because we are only changing the color property of the style of the target element.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
MutationObserver Example
</ title >
< style >
#colorDiv {
width: 100px;
height: 100px;
/* Giving red color initially to the box */
background-color: red;
}
</ style >
</ head >
< body >
< div id = "colorDiv" ></ div >
< script >
function mutationCallback(entries, observer) {
const mutation = entries[0];
if (mutation.type === 'attributes'
&& mutation.attributeName === 'style') {
const colorDiv = document
.getElementById('colorDiv');
const computedStyle = getComputedStyle(colorDiv);
const currentColor = computedStyle.backgroundColor;
console.log('Color changed to:', currentColor);
}
}
// Create a mutation observer object
const observer =
new MutationObserver(mutationCallback);
// Start observing the target
// node for attribute changes
const config = {
attributes: true, attributeFilter: ['style']
};
observer.observe(colorDiv, config);
// Simulate changing the color after 3 seconds
setTimeout(() => {
colorDiv.style
.backgroundColor = 'blue';
}, 4000);
setTimeout(() => {
colorDiv.style
.backgroundColor = 'black';
}, 6000);
</ script >
</ body >
</ html >
|
Explanation: Initially the color was red. After 4 sec, it changed from red to blue, and then after 2 more seconds, changed to green. MutationObserver API is fully able to detect the changes successfully and print color values onto the console.
Output:
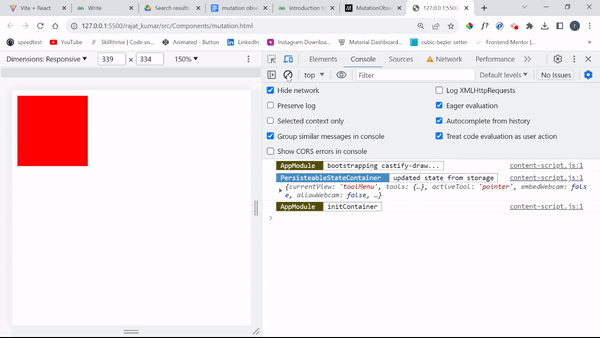
Color change detection using Mutation Observer API
We are given an editable paragraph text. When we add a new line to the text paragraph and hit ENTER, then our program should detect this mutation of adding a new line in the given paragraph and should log the changed paragraph on the console. Detection of the first line of a given paragraph is not required.
Example: we will detect only the changes made directly to the target element (i.e.; paragraph ). If the change is in childList==true, then check when the user is going to hit ENTER (i.e.; line break ). If a line break is found, it means the user has completed adding the data into the current line and entered into a new line. so now we can update our current paragraph data and log the same on the console.
HTML
<!DOCTYPE html>
< html >
< head >
< title >
MutationObserver Example
</ title >
< meta charset = "utf-8" >
</ head >
< body >
< p contenteditable oninput = "" >
Change this text & look at the
console after pressing ENTER
</ p >
< script >
// Selecting the target element
let target = document.querySelector('p');
let observer = new MutationObserver(
function (mutations) {
// As we have to observe only a single element ,
// so taking mutations[0] into mutation
mutation = mutations[0];
// When mutation type is childlist then we
// will be checking for line break
if (mutation.type === 'childList') {
let target_values = [].slice
.call(target.children)
.map(function (node) {
return node.innerHTML
})
.filter(function (s) {
// When user has hit ENTER, then this
// condition is going to hold true
if (s === '< br >')
return false;
return true;
});
// Logging the updated target list
// stored inside target_values
console.log(target_values);
}
});
// Taking only childlist in config parameter
let config = {
childList: true,
}
// Calling observe() method using observer instance
// this trigger observing mutation of the target element
observer.observe(target, config);
</ script >
</ body >
</ html >
|
Output:
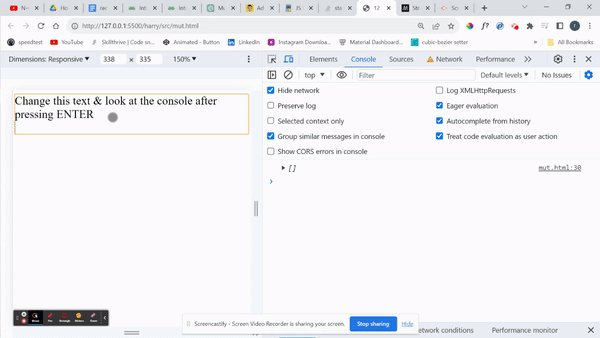
Detecting paragraph mutation using API
Javascript provides some other observer APIs like Intersection Observer, Resize Observer, etc. Each observer API has different use cases and is introduced to provide some kind of optimizations over the traditional way of observing DOM elements.
Share your thoughts in the comments
Please Login to comment...