Using styled API in ReactJS
Last Updated :
28 Dec, 2020
Styled components in ReactJS is a CSS-in-JS library that can be used for a better UI design. This API allows us to create a styled component and apply all styling properties. For using Styled API in React we need to install styled components.
Prerequisites:
Steps to use styled components in ReactJS:
Step 1: Before moving further, firstly we have to install the styled component, by running the following command in your project directory, with the help of terminal in your src folder or you can also run this command in Visual Studio Code’s terminal in your project folder.
npm install --save styled-components
Step 2: After installing the modules, now open your App.js file which is present inside your project’s directory, under src folder.
Step 3: Now import React and styled modules.
Step 4: In your App.js file, add this code snippet to import React and styled modules.
import React from 'react';
import styled from 'styled-components';
Below is a sample program to illustrate the use of styled-components:
Example 1: Changing the background color of the button to green.
App.js:
Javascript
import React from 'react' ;
import styled from 'styled-components' ;
const Button = styled.a`
background-color:green;
color: white;
padding: 1rem 2rem;
margin-top:100px;
width: 150px;
display: block;
`
const App = () => {
return (
<center><Button>GeeksforGeeks</Button></center>
)
}
export default App;
|
Output:
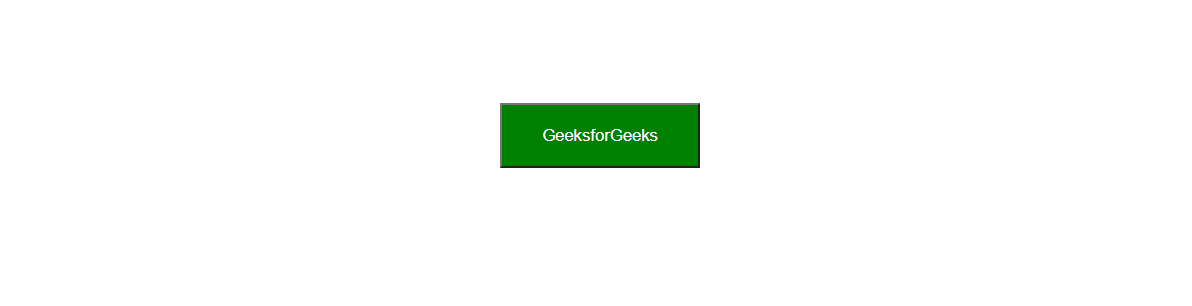
Example 2: Adding a border to the button.
App.js:
Javascript
import React from 'react' ;
import styled from 'styled-components' ;
const Button = styled.a`
background-color:green;
color: white;
padding: 1rem 2rem;
margin-top:100px;
width: 150px;
display: block;
border:8px solid black;
`
const App = () => {
return (
<center><Button>GeeksforGeeks</Button></center>
)
}
export default App;
|
Output:
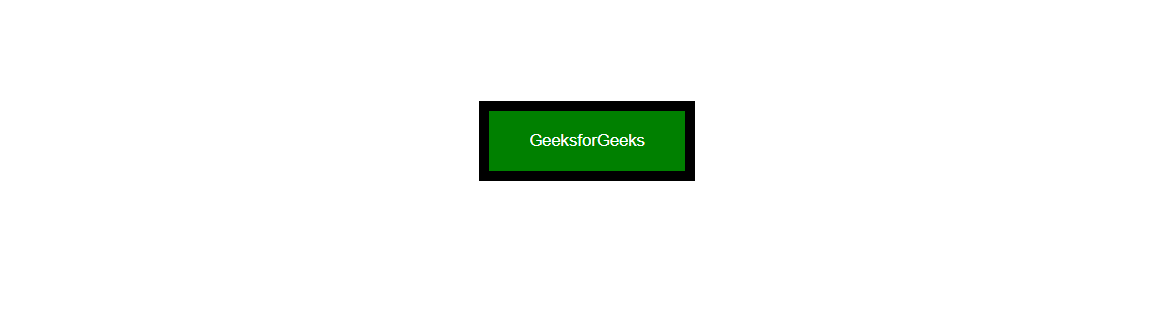
Hence, using the above-mentioned steps, we can use the Styled-components to import and change the style of components in React.
Share your thoughts in the comments
Please Login to comment...