TypeScript in operator narrowing Type
Last Updated :
18 Oct, 2023
In this article, we will learn about the ‘in‘ operator narrowing Type in Typescript. In TypeScript, the ‘in’ operator is used to narrow or refine the type of an object within a conditional statement or block. It checks whether a specific property or key exists within an object, and if it does, it narrows the type of that object to include that property.
Syntax
if (propertyKey in object) {
// Narrowed type where object
// includes propertyKey
} else {
// Narrowed type where object
// does not include propertyKey
}
Where,
- propertyKey: This is a string literal or string variable representing the property or key you want to check for in the object.
- object: This is the object in which you want to check for the existence of the property indicated by propertyKey.
Example 1: In this example, The printPersonInfo function takes an argument person, which is of type Person. A person is an interface with a name property and an optional age property. Inside the function, we use the in operator to check whether the age property exists in the person object. If the age property exists (‘age’ in person evaluates to true), TypeScript narrows the type of person inside the if block to include the age property. We can then safely assess person.age.If the age property does not exist (‘age’ in person evaluates to false), TypeScript narrows the type inside the else block to exclude the age property.
Javascript
interface Person {
name: string;
age?: number;
}
function printPersonInfo(person: Person) {
if ( 'age' in person) {
console.log(`Name: ${person.name}, Age: ${person.age}`);
} else {
console.log(`Name: ${person.name}, Age not provided`);
}
}
const GeeksforGeeks: Person =
{ name: 'GeeksforGeeks' , age: 30 };
const Geek: Person = { name: 'Geek' };
printPersonInfo(GeeksforGeeks);
printPersonInfo(Geek);
|
Output:

Example 2: In this example, the Human interface has three properties: name, canSwim, and canFly. Both canSwim and canFly are optional properties. The described HumanAbilities function takes a human argument of type Human. Inside the function, we use the in operator to check whether canSwim and canFly properties exist in the human object. Depending on whether ‘canSwim’ in humans and ‘canFly’ in humans are true or false, different code blocks are executed. The human object’s type is narrowed based on the presence or absence of the canSwim and canFly properties, and the function describes the human’s abilities accordingly.
Javascript
interface Human {
name: string;
canSwim?: boolean;
canFly?: boolean;
}
function describeHumanAbilities(human: Human) {
if ( 'canSwim' in human) {
console.log(`${human.name} can swim.`);
} else {
console.log(`${human.name} cannot swim.`);
}
if ( 'canFly' in human) {
console.log(`${human.name} can fly.`);
} else {
console.log(`${human.name} cannot fly.`);
}
}
const john: Human = { name: 'A' , canSwim: true };
const sarah: Human = { name: 'B' , canFly: true };
const mike: Human = { name: 'C' };
describeHumanAbilities(john);
describeHumanAbilities(sarah);
describeHumanAbilities(mike);
|
Output:
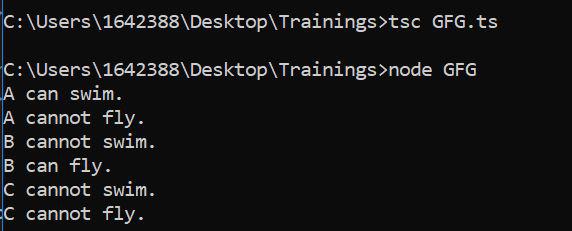
Reference: https://www.typescriptlang.org/docs/handbook/2/narrowing.html#the-in-operator-narrowing
Share your thoughts in the comments
Please Login to comment...