TypeScript Truthiness Narrowing Type
Last Updated :
25 Oct, 2023
In this article, we are going to learn about Truthiness narrowing Type in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. In TypeScript, truthiness narrowing is a concept that allows you to narrow down the type of a variable based on its truthiness (or falseness) in a conditional statement. It’s a type inference mechanism that takes advantage of JavaScript’s truthy and falsy values to determine the possible types of a variable.
Truthy Values: In JavaScript, values that are considered “truthy” are those that are treated as true when evaluated in a boolean context. These values include:
- Non-empty strings (“hello”, “true”, etc.)
- Numbers other than 0 (1, -42, etc.)
- Objects (including arrays and functions)
- true
- Instances of user-defined classes with a valueOf method returning true
False Values: Conversely, “falsy” values are those that are treated as false in a boolean context. These values include:
- Empty strings (“”)
- The number 0 (0)
- null
- undefined
- false
- 0n (the bigint version of zero)
- NaN (Not-a-Number)
Syntax:
if (condition) {
// Inside this block, TypeScript narrows the
// type of a variable.
} else {
// Inside this block, TypeScript knows
// something different about the variable.
}
Where-
- condition: This is the expression or variable that you want to check for truthiness or falsiness.
- Inside the if block: TypeScript narrows the type of a variable based on the truthiness of the condition. If the condition is considered true, TypeScript will narrow the type of the variable accordingly.
- Inside the else block (optional): TypeScript will know something different about the variable compared to the if block, based on the fact that the condition evaluated to false.
Example 1: In the if block, TypeScript narrows down the type of name to string because it checks if name is truthy (i.e., not null or undefined). In the else block, TypeScript understands that name can only be null or undefined because it has already checked for truthiness
Javascript
function greet(name: string | null ) {
if (name) {
console.log(`Hello, ${name.toUpperCase()}!`);
} else {
console.log( "Hello, GeeksforGeeks!" );
}
}
greet( "GeeksforGeeks" );
greet( null );
|
Output: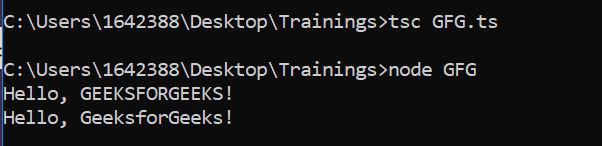
Example 2: In this example, the printType function takes a parameter value. Inside the function, we use typeof to check the type of value. Depending on the type, TypeScript narrows the type of value inside each if block. This allows us to perform type-specific operations safely.
Javascript
function printType(value: any) {
if ( typeof value === 'string' ) {
console.log( "It's a string:" , value.toUpperCase());
} else if ( typeof value === 'number ') {
console.log("It' s a number:", value.toFixed(2));
} else {
console.log( "It's something else:" , value);
}
}
printType( "Hello, GeeksforGeeks!" );
printType(42);
printType( true );
|
Output:
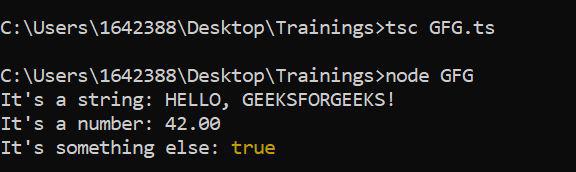
Share your thoughts in the comments
Please Login to comment...