TypeScript Return Type Annotations
Last Updated :
25 Oct, 2023
In this article, we are going to learn about the Return Type Annotations in Typescript. TypeScript is a popular programming language used for building scalable and robust applications. One of the features of Typescript is Return Type Annotations which helps to explicitly declare the type of value that a function is expected to return. Return type annotations are added to the function’s signature and come after the parameter list using a colon (:) followed by the desired return type.
Syntax:
function functionName(parameters: parameterType): returnType {
// Body of function
}
Where-
- functionName is the name of the function.
- parameters is a list of function parameters with their types.
- returnType is the type that the function is expected to return.
Example 1: In this example, ‘multiply’ is the function name. ‘a’ and ‘b’ are parameters with type numbers. number specifies that the function is expected a number as a return value. We can change that return type according to our need e.g.: string, boolean, function, object, array, null, and void.
Javascript
function multiply(a: number, b: number): number {
return a * b;
}
console.log(multiply(7, 2))
|
Output:
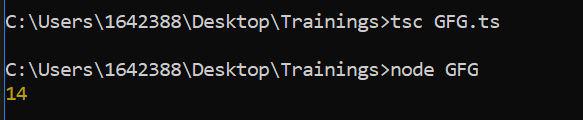
Example 2: In this example, we will see Promise as the return type. It indicates that the function returns a promise that resolves to a specific type.We define a function fetchData that returns a Promise<string>. This means that fetchData is expected to return a promise that will resolve to a string. We call fetchData() and then use the .then() method to handle the resolved value.
Javascript
async function fetchData(): Promise<string> {
return "Hi Geek!! Data fetched successfully" ;
}
fetchData().then((result) => {
console.log(result);
});
|
Output: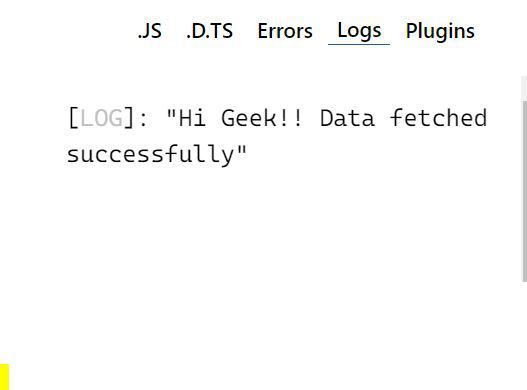
Share your thoughts in the comments
Please Login to comment...