TypeScript Non-null Assertion Operator (Postfix !) Type
Last Updated :
02 Nov, 2023
TypeScript non-null assertion operator (!) is used to assert that a value is non-null and non-undefined, even when TypeScript’s strict null checks are enabled. This operator tells TypeScript to treat the expression as if it’s guaranteed to have a value, eliminating compile-time null and undefined checks.
Syntax
const nonNullValue: Type = value!;
Parameters
- nonNullValue is the variable where you want to store the non-null value.
- Type is the expected type of the value.
- value is the variable or expression that you’re asserting to be non-null and non-undefined using the! operator.
Example 1: In this example,
- We have a function greetUser() that takes a name parameter, which can be a string or null.
- Inside the function, we use the non-null assertion operator name! to assert that the name is not null or undefined.
- We then use formattedName to hold the non-null value of the name, allowing us to treat it as a string.
- We log a greeting message that includes the user’s name if provided, or “GeeksforGeeks” if the name is null.
Javascript
function greetUser(name: string | null ) {
const formattedName: string = name!;
console.log(
`Hello, ${formattedName || 'GeeksforGeeks' }!`);
}
greetUser( "Akshit" );
greetUser( null );
|
Output:
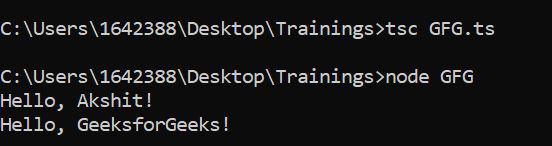
Example 2: In this example:We define a User type representing a user object with potentially nullable properties. We create a user object with a name property (non-nullable) and an email property (nullable). We use the non-null assertion operator ! to assert that the email property is non-null when accessing it. This is done because TypeScript considers user.email to be potentially undefined due to the email? property definition in the User type.
We then log both the userName and userEmail variables. TypeScript will not raise any errors related to nullability because of the non-null assertion operator.
Javascript
type User = {
name: string;
email?: string;
};
const user: User = {
name: "GeeksforGeeks" ,
};
const userName: string = user.name;
const userEmail: string = user.email!;
console.log(`User Name: ${userName}`);
console.log(`User Email: ${userEmail}`);
|
Output:
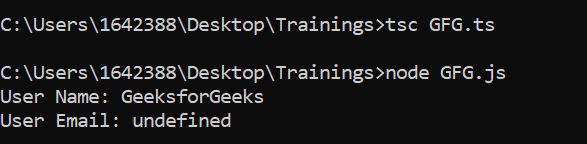
Reference: https://www.typescriptlang.org/docs/handbook/release-notes/typescript-2-0.html#non-null-assertion-operator
Share your thoughts in the comments
Please Login to comment...