Tree-based algorithms are a fundamental component of machine learning, offering intuitive decision-making processes akin to human reasoning. These algorithms construct decision trees, where each branch represents a decision based on features, ultimately leading to a prediction or classification. By recursively partitioning the feature space, tree-based algorithms provide transparent and interpretable models, making them widely utilized in various applications. In this article, we going to learn the fundamentals of tree based algorithms.
What is Tree-based Algorithms?
Tree-based algorithms are a class of supervised machine learning models that construct decision trees to typically partition the feature space into regions, enabling a hierarchical representation of complex relationships between input variables and output labels. Examples of notable are random forests, Gradient Boosting techniques and decision trees, using recursive binary split based on criteria like Gini impurity or information gain etc. These algorithms show versatility in use in classification and regression functions, for robustness against overfitting by ensemble methods and generates more individual trees Ability to allow exploratory analysis of feature importance, which is economical, which contributes to widespread application in various fields like healthcare and natural language processing.
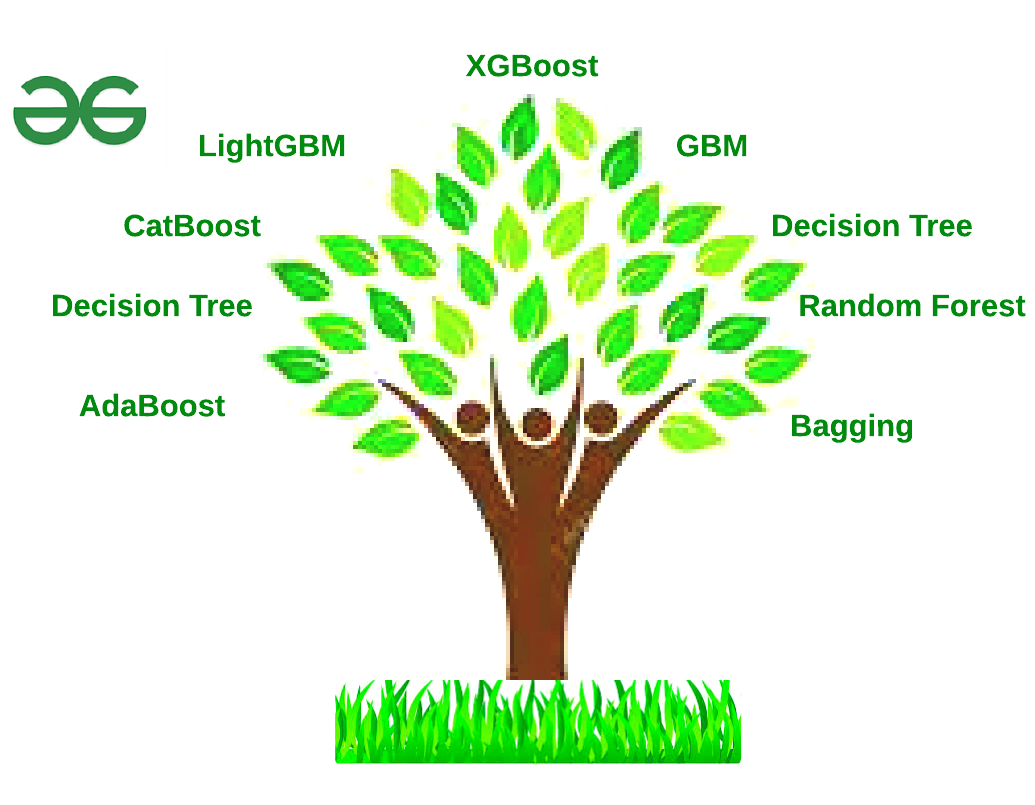
Tree-based ML models
How do Tree-based algorithm work?
The main four workflows of tree-based algorithms are discussed below:
- Feature Splitting: Tree-based algorithms begin by selecting more informative features to split a data set based on a specific criterion, such as Gini impurity or information gain etc.
- Recursive splitting: The selected feature of dataset is used to split the data in two, and the process is repeated for each resulting subset, forming a hierarchical binary tree structure. This recursive splitting until stops a predefined criterion, like a maximum depth or a minimum number of samples per train data, is met as long as it lasts.
- Leaf Node Function: As the tree grows, each terminal node (leaf) is given a predicted outcome based on majority learning (for classification) or the sample value of that node of the (for regression). This activates the tree to capture complex decision boundaries and relationships in the data.
- Ensemble Learning: For ensemble methods like Random Forests and Gradient Boosting Machines, multiple trees are trained independently, and their predictions are combined to obtain the final result. This group approach helps to reduce overfitting, increase generalization, and improve overall model performance by combining the strengths of individual trees and reducing their weaknesses.
Splitting Process
Gini Impurity
Gini impurity is a measure of the lack of homogeneity in a dataset which specifically calculates the probability of misclassifying an instance chosen uniformly at random. The splitting process involves evaluating potential splits based on Gini impurity for each feature. The algorithm selects the split that minimizes the weighted sum of impurities in the resulting subsets, aiming to create nodes with predominantly homogeneous class distributions.
Python3
import graphviz
from sklearn.datasets import load_breast_cancer
from sklearn.tree import DecisionTreeClassifier, export_graphviz
data = load_breast_cancer()
X, y = data.data, data.target
clf = DecisionTreeClassifier(criterion = 'gini' , random_state = 42 )
clf.fit(X, y)
dot_data = export_graphviz(clf, out_file = None , feature_names = data.feature_names)
graph = graphviz.Source(dot_data)
graph.render( "decision_tree" )
|
Output:
decision_tree.pdf
The image decision tree will be stored in decision_tree.pdf.
Entropy
Entropy is a measure of information uncertainty in a dataset. In the context of decision trees, it quantifies the impurity or disorder within a node. The splitting process involves assessing candidate splits based on the reduction in entropy they induce. The algorithm selects the split that maximizes the information gain, representing the reduction in uncertainty achieved by the split. This results in nodes with more ordered and homogenous class distributions, contributing to the overall predictive power of the tree.
Python3
import graphviz
from sklearn.datasets import load_breast_cancer
from sklearn.tree import DecisionTreeClassifier, export_graphviz
data = load_breast_cancer()
X, y = data.data, data.target
clf = DecisionTreeClassifier(criterion = 'entropy' , random_state = 42 )
clf.fit(X, y)
dot_data = export_graphviz(clf, out_file = None , feature_names = data.feature_names)
graph = graphviz.Source(dot_data)
graph.render( "decision_tree2" )
|
Output:
decision_tree2.pdf
The image decision tree will be stored in decision_tree2.pdf.
Information Gain
Information gain is a concept derived from entropy, measuring the reduction in uncertainty about the outcome variable achieved by splitting a dataset based on a particular feature. In tree-based algorithms, the splitting process involves selecting the feature and split point that maximize information gain. High information gain implies that the split effectively organizes and separates instances, resulting in more homogeneous subsets with respect to the target variable. The goal is to iteratively choose splits that collectively lead to a tree structure capable of making accurate predictions on unseen data.
Decision Tree
A decision tree is a visual tool used to guide decision-making by considering different conditions. It resembles an inverted tree with branches and leaves pointing downwards. At each branch, a decision is made based on specific criteria, leading to a conclusion at the end of each branch. Decision trees are valuable for structuring decisions and problem-solving processes. At each branch, you make a choice based on certain conditions, and eventually, you reach a conclusion at the end of a branch. Decision trees are commonly used in various fields, such as business, education, and medicine, to help people make choices and solve problems.
The key-working principle of Decision Tree are discussed below:
- Root node: The root node is the beginning of the decision tree, which represents the entire data set. The resulting tree decision process in the initial state considering all available information for subsequent analysis and classification.
- Branching criteria: During the construction of a decision tree, it automatically identifies the most important features or features to better differentiate the data points. These identified elements become “segmentation criteria”, which guide the creation of branches that encompass different answers to specific questions based on selected attributes.
- Decision nodes: For each decision node in the tree, a feature-specific query is posed. For example, in a data set focused on home buyers, the decision node asks, “Does the annual income exceed $50,000?” The answer to this question indicates subsequent paths, which lead to additional decision nodes.
- Leaf Root: What culminates in walking through the branches is in the leaf, where specific decisions or allocations are made. In a practical context, a leaf node can provide conclusions such as “likely to buy a home” for a set of homebuyer data. These leaf nodes represent the final output of the decision-making process.
- Training a tree: The intelligence of a decision tree comes from its ability to recognize patterns in the training data. It adapts and adapts dynamically to make more informed decisions by identifying complex relationships and dynamics among data set elements.
- Prediction: As new data arrives, the decision tree traverses, answering feature-based queries at each node. This sequential process continues until a leaf node is found, providing a better-informed prediction or decision of a new data point based on known patterns and classification criteria.
Random Forest Algorithm
Random Forest Algorithm combines the power of multiple decision trees to create robust and accurate predictive models. It works on the principle of group learning, where multiple individual decision trees are built independently in which each is trained on a random subset of data reduces ting and increases the generalizability of the model. When a prediction is needed, each tree in the forest gives its vote, and the algorithm combines these votes together by aggregation to give the final prediction. This tree-based approach not only improves the prediction accuracy but also increases the algorithm’s ability to detect noisy data and outliers.
Working of Random Forest Algorithm
- Random Subset: To keep things interesting, each tree in the forest is trained on a different random subset of the data. Each team member seems to get a unique view of part of the overall picture.
- Random Selection: As the team members have different skills, each pole focuses on specific areas during training. This random feature selection adds another layer of diversity to the group, so that they don’t all see the data the same way.
- Majority Voting: When it’s time to predict, each tree votes based on their own logic. It’s like taking a vote from each team member on what they think the outcome should be.
- Majority Rule: The algorithm does not rely solely on single tree views. Instead, it considers the collective intelligence of the entire forest. The final decision is based on a majority vote, allowing for stronger and more reliable predictions.
Gradient Boosting Machines
Gradient Boosting Machine is like a team of little learners that work together to solve a big problem. Each learner starts with some basic knowledge and tries to improve by focusing on the mistakes made by the previous learners. They keep getting better and better at solving the problem until they reach a good solution. This teamwork approach helps Gradient Boosting Machines to tackle complex tasks effectively by combining the strengths of multiple simple learners.
XGBoost
eXtreme Gradient Boosting, often abbreviated as XGBoost, is a sophisticated method in computer science for solving problems through learning. The algorithm combines multiple decision trees to make accurate predictions. The model continuously improve from its past mistakes. It can handle a wide range of tasks, such as categorizing data or predicting values, with high precision and efficiency.
Here’s how XGBoost works:
- XGBoost acts as a team captain overseeing a group of decision-makers, represented by decision trees. Each tree contributes its perspective, and they collectively make the final decision.
- XGBoost is a quick learner that pays close attention to its past errors during training. It adjusts its approach accordingly, similar to a student focusing on improving in areas where they previously struggled.
- To prevent overfitting, XGBoost employs a coach that uses regularization techniques. This ensures that the decision-makers remain focused and don’t overcomplicate things with unnecessary details.
- XGBoost optimizes a specialized function, known as a loss function, to make the best decisions. It constantly evaluates and adjusts its strategy to efficiently navigate through complex problems.
Other ensemble methods
Now we are going discuss other some popular Ensemble methods below:
Adaptive Boosting
AdaBoost, short for Adaptive Boosting, is an ensemble learning algorithm designed to improve the performance of weak learners by iteratively focusing on misclassified instances. It trains a series of weak learners, typically shallow decision trees, on the dataset with adjusted weights. In each iteration, it increases the weights of misclassified instances, emphasizing their correct classification in subsequent rounds. This process continues for a predefined number of rounds, culminating in an ensemble prediction obtained by combining the weak learners based on their individual performance.
AdaBoost operates on four core principles:
- Team Learning: AdaBoost forms a learning team akin to having various mentors with distinct strengths and weaknesses. It creates a series of weak learners, akin to mentors with limited expertise, and combines their insights to form a stronger, more capable team.
- Focused Improvement: After each round of learning, AdaBoost prioritizes areas of mistakes and concentrates on them in the next round, similar to focusing on challenging subjects to enhance overall performance. This targeted approach ensures effective learning and improvement.
- Adaptive Learning: AdaBoost adapts its approach based on past errors, continually refining its strategy akin to a savvy mentor adjusting to student progress. This adaptability ensures the algorithm evolves with the learning process, contributing to the development of a powerful and nuanced model.
- Collective Wisdom: AdaBoost integrates the collective wisdom of the team into a final comprehensive model, akin to mentors collaborating to provide unique insights. This collaborative effort results in a robust and accurate solution adept at handling complex tasks effectively.
LightGBM
LightGBM, or Light Gradient Boosting Machine utilizes a histogram-based learning approach, which bins continuous features into discrete values to speed up the training process. LightGBM introduces the concept of “leaf-wise” tree growth, focusing on expanding the leaf nodes that contribute the most to the overall reduction in the loss function. This strategy leads to a faster training process and improved computational efficiency. Additionally, LightGBM supports parallel and GPU learning, making it well-suited for large datasets. Its ability to handle categorical features, handle imbalanced datasets, and deliver competitive performance has made LightGBM widely adopted in machine learning applications where speed and scalability are critical.
Working principle of LightGBM:
- Gradient Boosting with Light Footprint: LightGBM is like a quick decision-maker in a group project, where it efficiently learns from data by focusing on the areas that need improvement the most. Its gradient boosting technique is optimized to be lightweight, ensuring it doesn’t burden itself with unnecessary details and swiftly processes information.
- Histogram-Based Learning: Imagine LightGBM as a smart reader that quickly grasps the essence of a book without delving into every word. It uses histogram-based techniques to represent and process data efficiently, enabling it to make informed decisions without getting bogged down by excessive data intricacies.
- Leaf-Wise Tree Growth: LightGBM is resource-savvy, growing its decision-making trees in a leaf-wise fashion. This means it strategically expands the parts of the tree that contribute most to improving accuracy, enhancing efficiency by avoiding unnecessary tree growth. It’s like building a treehouse by adding branches where they matter most.
- Optimized Training Process: LightGBM is your high-speed companion on the machine learning journey, employing an optimized training process. By focusing on what truly matters and avoiding unnecessary computations, it ensures a swift learning experience, akin to a streamlined highway that takes you efficiently to accurate predictive outcomes.
CatBoost
CatBoost, developed by Yandex, stands out as a potent gradient boosting framework tailored for seamless handling of categorical features. It employs a symmetric tree structure and a blend of ordered boosting and oblivious trees, streamlining the management of categorical data without extensive preprocessing. Unlike conventional methods, CatBoost integrates “ordered boosting” to optimize the model’s structure and minimize overfitting during training. Furthermore, it boasts automatic processing of categorical features, eliminating the need for manual encoding. With advanced regularization techniques to curb overfitting and support for parallel and GPU training, CatBoost accelerates model training on large datasets, offering competitive performance with minimal hyperparameter tuning.
CatBoost’s efficiency lies in its unique handling of categorical features, eliminating the need for manual preprocessing. It combines oblivious trees and ordered boosting to directly incorporate categorical variables during training, capturing intricate data relationships seamlessly. Additionally, its symmetric tree structure dynamically adjusts tree depth, mitigating overfitting by adapting to data complexity. With advanced regularization methods like the “Ctr” complexity term, CatBoost controls model complexity and ensures robustness. The ordered boosting strategy optimizes tree sequences, enhancing the model’s structure and learning process, while support for parallelization and GPU acceleration facilitates efficient training on vast datasets, underscoring CatBoost’s scalability and real-world performance.
Advantages of Tree-Based Algorithms
The advantages of Tree-Based algorithms are discussed below:
- Easy to Understand: Tree-based algorithms are like having a smart friend who explains things in a simple way. They create decision trees that help computers make choices step by step, making it easy for us to follow how decisions are made.
- Versatile Problem Solvers: These algorithms are super versatile, like all-in-one problem solvers. Whether it’s figuring out categories or solving more complex problems, tree-based algorithms can handle a bunch of different tasks, making them reliable helpers for computers.
- Good with Patterns: Just as some people are great at solving puzzles, tree-based algorithms are excellent at finding patterns in data. They can see connections between things that might seem unrelated, helping computers make sense of complex information.
- Transparent Decision-Making: Unlike some traditional ML algorithms, tree-based ones are transparent decision-makers. They show us exactly how they reach a conclusion, like an open book. This transparency makes them trustworthy and easy to work with, giving us insights into how computers make smart decisions.
Disadvantages of Tree-Based Algorithms
Some of the disadvantages of Tree-Based algorithms are discussed below:
- Overfitting issue: Sometimes tree-based algorithms can get a little too lucky to learn from the data they see. This is called overfitting, where too much attention is paid to the details of training and struggles in the face of unseen new data.
- Sensitivity to small changes: A tree-based algorithm can seem a little mechanically simple. Sometimes small changes in the data can lead to large changes in the model, making it skewed. This sensitivity suggests that it may not be the best choice where the data is noisy or constantly changing.
- High complexity: While decision trees are great at simplifying decision making, when grouped together like Random Forests or Gradient Boosting, things can get a little more complicated. These large groups of trees can be difficult to maintain and understand, making them less straightforward in an implementation.
Share your thoughts in the comments
Please Login to comment...