Python is a widely used high-level, general-purpose programming language. The language offers many benefits to developers, making it a popular choice for a wide range of applications including web development, backend development, machine learning applications, and all cutting-edge software technology, and is preferred for both beginners as well as experienced software developers.
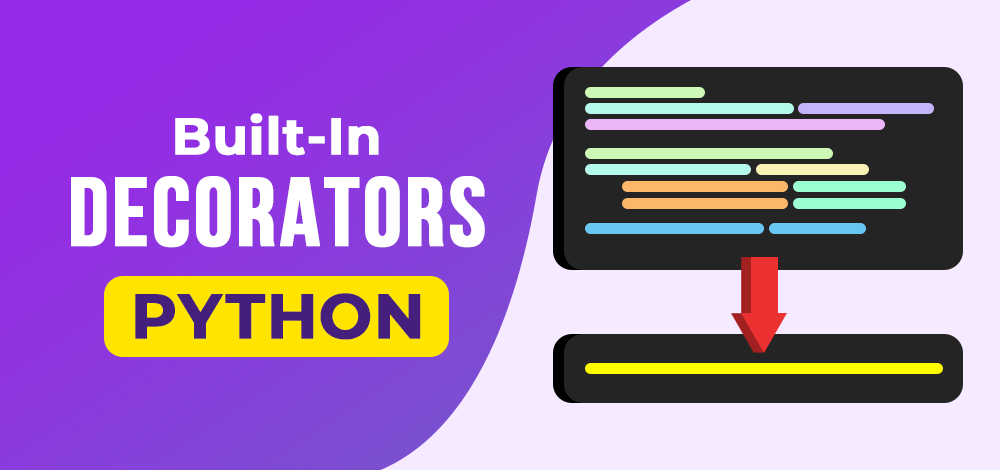
Python decorators are valuable tools for writing clean, versatile, reusable, and maintainable code, and as a result, are used extensively by software developers. Decorators allow you to extend the behavior of a function or class without changing its source code. They are also widely used in many Python frameworks and libraries, such as Flask, Django, and SQLAlchemy. Additionally, there are many awesome built-in Python decorators that make our lives much easier because we can add complicated functionalities to existing functions or classes with just one line of code. Decorators are commonly used in Python for caching, logging, timing, authentication, authorization, and validation. With this being said let’s continue the article on the best Python built-in decorators to assist you in having a much easier time learning and building with Python.
Top 10 Python Built-In Decorators That Optimize PythonCode Significantly
1. @classmethod
Class methods are methods that are bound to a particular class. These methods are not tied to any instances of the class. The ‘@classmethod’ is a built-in decorator that is used to create a class method.
Example:
Python3
class GFGClass:
class_var = 10
@classmethod
def gfg_class_method( cls ):
print ( "This is a Class method" )
print (f "Value of class variable {cls.class_var}" )
GFGClass.gfg_class_method()
|
Output:
This is a Class method
Value of class variable 10
In the above implementation, a class GFGClass and a class method gfg_class_method are defined. The @classmethod decorator is used to indicate that this is a class method. The cls parameter in the method definition refers to the class itself, through which the class-level variables (example: class_val) and methods can be accessed. When gfg_class_method() method is called it logs the message, “This is a Class method” followed by the value of the class_var.
Also Read: @classmethod in Python
2. @abstractmethod
Abstract methods are declared inside an Abstract class without any method definition. This method is meant to be implemented by the base class who implements the parent abstract class. The @abstractmethod decorator provided by abc module is used to implement abstract methods.
Python3
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area( self ):
pass
class Square(Shape):
def __init__( self , side):
self .side = side
def area( self ):
return self .side * * 2
square = Square( 10 )
print (square.area())
|
Output:
100
In the above implementation, The Shape class is defined as an abstract base class by inheriting from ABC and using the @abstractmethod decorator to declare the area() method as abstract. The Square class is a concrete subclass of Shape that implements the area() method to calculate the area of a square. By inheriting from Shape, Square must implement all of the abstract methods declared in Shape. In this case, the Square class implements area() by squaring the length of one side of the square.
Also, Read: @abstractmethod in Python
3. @staticmethod
Static methods are simply utility methods that are not bound to a class or an instance of the class. The ‘@staticmethod‘ is a built-in decorator that is used to create a static method.
Example:
Python3
class GFGClass:
@staticmethod
def gfg_static_method():
print ( "This is a static method" )
GFGClass.gfg_static_method()
|
Output:
This is a static method
In the above implementation, a class GFGClass and a static method gfg_static_method are defined. The @staticmethod decorator is used to indicate that this is a static method. Unlike class methods, static methods do not have a special first parameter that refers to the class itself. Static methods are often used for utility functions that do not depend on the class or the instance of the class. When the gfg_static_method() method is called on the GFGClass class, the message “This is a static method” is logged to the console.
Also, Read: @staticmethod in Python
4. @atexit.register
The ‘@atexit.register’ decorator is used to call the function when the program is exiting. The functionality is provided by the ‘atexit’ module. This function can be used to perform the clean-up tasks, before the program exits. Clean-ups are the tasks that have been done in order to release the resources such as opened files or database connections, that were being used during the program execution.
Example:
Python3
import atexit
@atexit .register
def gfg_exit_handler():
print ( "Bye, Geeks!" )
print ( "Hello, Geeks!" )
|
Output:
Hello, Geeks!
Bye, Geeks!
In the above implementation, a function gfg_exit_handler() is defined which uses the @atexit.register decorator that registers it to be called when the program is exiting. When we run the program, the message “Hello, Geeks!” is logged into the console. When completes its execution either or by being interrupted, the gfg_exit_handler() function is called and the message “Bye, Geeks!” is logged to the console. The gfg_exit_handler() function can be useful for performing cleanup tasks or closing resources (such as files or database connections) when a program is exiting.
Also, Read: @atexit.register in Python
5. @typing.final
The ‘@final’ decorator from the typing module is used to define the final class or method. Final classes cannot be inherited and in a similar way, final methods cannot be overridden.
Example:
Python3
import typing
class Base:
@typing .final
def done( self ):
print ( "Base" )
class Child(Base):
def done( self ):
print ( "Child" )
@typing .final
class Geek:
pass
class Other(Geek):
pass
|
In the above implementation, the @typing.final decorator is used to indicate that a method or class should not be overridden or subclassed, respectively. The Base class has a done() method marked as final using @typing.final. The Child is a subclass of Base that attempts to override the done() method, which raises an error when the code is executed. Similarly, the Geek class is marked as final using @typing.final, indicating that it should not be subclassed. The Other class attempts to subclass Leaf, which also raises an error when the code is executed.
Using @typing.final can help prevent unintended changes to critical parts of your code and make it more robust by enforcing constraints on how it can be used.
Also Read: @typing.final in Python
6. @enum.unique
Enumeration or Enum is a set of unique names that have a unique value. They are useful if we want to define a set of constants that have a specific meaning. The ‘@unique’ decorator provided by enum module is used to ensure that enumeration members are unique.
Example:
Python3
from enum import Enum, unique
@unique
class Days(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 2
|
In the above implementation, an enum class ‘Days’ is defined using the class ‘Enum‘. The class has 4 members, MONDAY, TUESDAY, WEDNESDAY, and THURSDAY, with values of 1,2,3,2 respectively.
However, because TUESDAY and THURSDAY both have the value 2, the @unique decorator raises a ValueError with the message “duplicate values found in <enum ‘Days’>: THURSDAY -> TUESDAY”. This error occurs because the @unique decorator ensures that the values of the enumeration members are unique, and in this case, they are not.
Also, Read: @enum in Python
7. @property
Getters and Setters are used within the class to access or update the value of the object variable within that class. The ‘@property’ decorator is used to define getters and setters for class attributes.
Example:
Python3
class Geek:
def __init__( self ):
self ._name = 0
@property
def name( self ):
print ( "Getter Called" )
return self ._name
@name .setter
def name( self , name):
print ( "Setter called" )
self ._name = name
p = Geek()
p.name = 10
print (p.name)
|
Output:
Setter called
Getter Called
10
In the above implementation, the Geek class has a private attribute ‘_name‘. The program defines the getter method ‘name‘ which allows the name to be retrieved using the dot notation. The @name.setter decorator is used to create the setter method for setting the ‘_name’ value. The @property and @name.setter decorators define the “getter” and “setter” methods for class attributes, which can make it easier to work with class data.
Also, Read: @property in Python
8. @enum.verify
Introduced in Python 3.11, The ‘@enum.verify‘ decorator in Python is used to ensure that the values of the members of an enumeration follow a particular pattern or constraint. Some predefined constraints provided by the enum include,
- CONTINUES: The CONTINUES modules help ensure that there is no missing value between the highest and lowest value.
- UNIQUE: The UNIQUE modules help ensure that each value has only one name
Example:
Python3
from enum import Enum, verify, UNIQUE, CONTINUOUS
@verify (UNIQUE)
class Days1(Enum):
MONDAY = 1
TUESDAY = 2
WEDNESDAY = 3
THURSDAY = 2
@verify (CONTINUOUS)
class Days2(Enum):
MONDAY = 1
TUESDAY = 2
THURSDAY = 4
|
In the above implementation, two different enumeration classes, Days1 and Days2 are defined using the Enum class from the enum module and the verify() decorator is applied to each class.
In the Days1 class, the verify() decorator with the UNIQUE argument is used to ensure that each member of the Days1 enumeration class has a unique value. However, as TUESDAY and THURSDAY have the same value, a ValueError is raised with the message: “ValueError: aliases found in <enum ‘Days’>: THURSDAY -> TUESDAY“.
In the Days2 class, the verify() decorator with the CONTINUOUS argument is used to ensure that each member of the Days2 enumeration class forms a continuous sequence starting from the first member. However, since the value of 3 is missing and 4 is directly used, a ValueError is raised with the message: “ValueError: invalid enum ‘Days’: missing values 3“.
Also Read: @enum in Python
9. @singledispatch
A function with the same name but different behavior with respect to the type of argument is a generic function. The ‘@singledispatch’ decorator in Python is used to create a generic function. This allows an individual to create an overloaded function that can work with multiple types of parameters.
Example:
Python3
from functools import singledispatch
@singledispatch
def geek_func(arg):
print ( "Function Call with single argument" )
@geek_func .register( int )
def _(arg):
print ( "Function Called with an integer" )
@geek_func .register( str )
def _(arg):
print ( "Function Called with a string" )
@geek_func .register( list )
def _(arg):
print ( "Function Called with a list" )
geek_func( 1 )
geek_func([ 1 , 2 , 3 ])
geek_func( "geek" )
geek_func({ 1 : "geek1" , 2 : "geek2" })
|
Output:
Function Called with an integer
Function Called with a list
Function Called with a string
Function Call with single argument
In the above implementation, a function called geek_func() is defined using the @singledispatch decorator. The @singledispatch decorator tells Python that this function should be dispatched based on the type of its first argument. Four additional implementations of geek_func() are defined using the @geek_func.register decorator. Each implementation is decorated with the type of argument it handles. When geek_func() is called with an argument of a particular type, the implementation decorated with the corresponding type is called. When the function call does not match any of the registered implementations, it defaults to the initial implementation.
Also, Read: @singledispatch in Python
10. @lru_cache
This decorator returns the same as lru_cache(maxsize=None) by creating a thin wrapper around a dictionary lookup for the function arguments. Because it never needs to remove old values, this is smaller and faster than lru_cache() with a size limit.
Example:
Python3
from functools import lru_cache
@lru_cache (maxsize = 128 )
def fibonacci(n):
if n < 2 :
return n
else :
return fibonacci(n - 1 ) + fibonacci(n - 2 )
start_time = time.time()
result = fibonacci( 10 )
print (result)
print ( "--- %s seconds ---" % (time.time() - start_time))
start_time = time.time()
result = fibonacci( 10 )
print (result)
print ( "--- %s seconds ---" % (time.time() - start_time))
|
Output:
55
--- 0.0009987354278564453 seconds ---
55
--- 0.0 seconds ---
Also, Read: @lru_cache in Python
In the above implementation of the Fibonacci sequence, we are using memoization with the lru_cache decorator from the functools module. The Fibonacci sequence is a sequence of numbers in which each number is the sum of the two preceding ones, starting from 0 and 1.
The fibonacci function is taking an integer argument n and returns the n-th number in the Fibonacci sequence and If n is less than 2, then the function returns n itself. Otherwise, it recursively computes the sum of the (n-1)-th and (n-2)-th numbers in the sequence.
Conclusion
Python is a versatile and widely-used programming language, making it a great choice for developers to implement code that is used for a variety of purposes. This article highlights some of the most popular and widely-used decorators available in Python. Decorators are useful abstractions for extending your code with additional functionalities such as caching, automatic retry, rate limiting, logging, and more. These not only provide powerful functionality but also simplify the code. Using decorators can provide developers with powerful tools for writing clear, concise, and efficient code in Python. Whether you are a beginner or an experienced developer, using these decorators will provide a good starting point for improving your code and leveraging the power of Python.
FAQs on Python Decorators
Q1: What is a decorator in Python?
Answer:
Python Decorators are very powerful tools that allow programmers to extend the behavior of a function or class.
Q2: How to create a decorator in Python?
Answer:
A decorator can be created by defining a function that takes another function as an argument and returns a new function that extends or modifies the original function’s behavior.
Q3: Can multiple decorators be used for the same function?
Answer:
Yes! It is possible to have multiple decorators on the same function.
Share your thoughts in the comments
Please Login to comment...