Python is one of the most popular languages for web development nowadays. It has various frameworks like Django, Flask, Hug, CherryPy, and Bottle that help developers write web applications in Python. Among all the frameworks Django is the most used framework to create websites in Python.
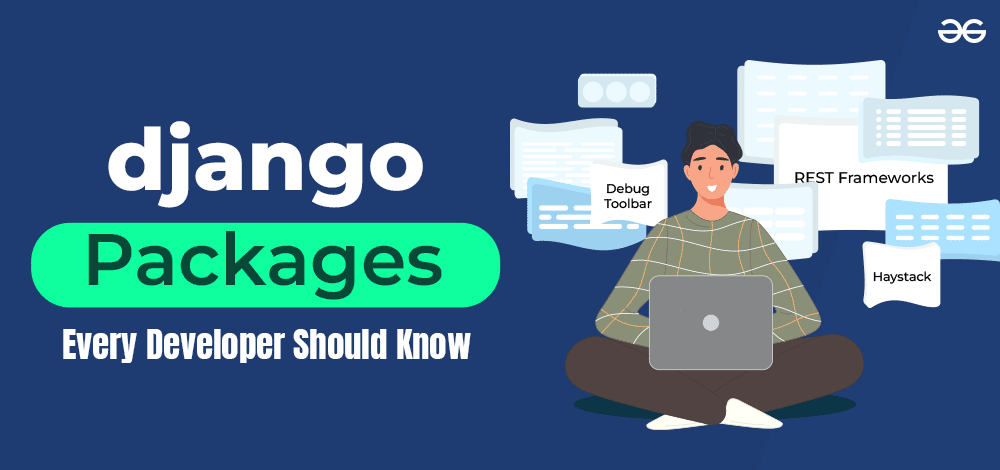
Django is an open-source and free-to-use full-stack web framework. It has several built-in libraries that support MySQL, SQLite, PostgreSQL, and Oracle as databases. It is a secure, stable, and scalable framework that helps us to create useful applications that are secure, stable, and scalable just like the framework.
What is Django?
Django is a high-level Python web framework assisting in the easy and quick building of web applications. It contains a set of components that help create web applications. It has built-in features for everything ranging from database management to admin properties. Various components in the framework work towards achieving a particular task like authentications, running the website, database management, etc. It focuses on reusability, less coding, low coupling, and fast development. Many well-known applications like Instagram, Mozilla, Bitbucket, PBS, NextDoor, etc. use the Django framework.
To learn Django, refer to this article: Getting Started with Django
Top 10 Django Packages
Django is an important web framework for Python which has a large support of packages and libraries. These packages have various functionalities such as providing security and authentication to simplify database management and speeding up development. From small scale applications to large and complex websites Django Packages help developers in making a reliable software application. Here is a list of the top 10 Django Packages every developer should know:
1. Django REST Frameworks
Django REST Frameworks popularly known as DRP is a package within the Django framework used to build Web APIs. Using this framework your work to create REST API yourself is eliminated.
Prerequisites:
- Python (3.6, 3.7, 3.8, 3.9, 3.10)
- Django (2.2, 3.0, 3.1, 3.2, 4.0, 4.1)
Features:
- The usage of Django REST Frameworks makes serialization easier.
- Compatible with both ORM and non-ORM data sources.
- Provides generic classes for performing CRUD operations in a hassle-free manner.
- Creation of REST API endpoints is fastened.
Configuration:
One way of installing the REST framework is pip install. Run the following command on your terminal:
pip install djangorestframework
REST framework can also be installed using the source code on GitHub. Download source code:
git clone https://github.com/encode/django-rest-framework
After installing the package either way mentioned above, add rest_framework to INSTALLED_APPS in the settings.py file:
INSTALLED_APPS = [
# Other installed apps
'rest_framework'
]
2. Django Allauth
Django Allauth is an integrated set of applications addressing authentication, registration, account management, and third-party account authentication. Many other Django apps focus on social authentication but local authentication is not included. Allauth bridges that gap by integrating both local and social authentication in one package.
Prerequisites:
- Python 2.6, 2.7, or 3.3.
- Django (1.4.3+)
Features:
- It supports over 50 authentication providers.
- Auth flow helps customize forms.
- Provides local authentication along with social authentication.
Configuration:
Installing the all auth using pip:
pip install django-allauth
In the settings.py file add installed apps, templates, and authentication backends:
INSTALLED_APPS = [
# Other installed apps
"django.contrib.admin",
"django.contrib.auth",
"django.contrib.contenttypes",
"django.contrib.sessions",
"django.contrib.messages",
"django.contrib.staticfiles",
"django.contrib.sites",
# Social Authentication
"allauth",
"allauth.account",
"allauth.socialaccount",
# Social providers
"allauth.socialaccount.providers.github",
]
TEMPLATES = [
{
...
"DIRS": [str(BASE_DIR.joinpath("templates"))],
...
},
]
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'allauth.account.auth_backends.AuthenticationBackend',
]
3. Django Redis Cache
For medium to large-sized websites, chacking is an important part of reducing the overhead computation which might lead to slower application. Redis is an in-memory database that can be used for caching for better performance by caching all the frequently requested data from the user.
Prerequisites:
- Latest version of Django and Python.
- Redis server installed and running.
Features:
- Pluggable clients, parsers, and serializers.
- Supports infinite timeouts.
- Raw access to Redis client/connection pool.
- Unix sockets are supported by default.
Configuration:
Installing the Redis-cache using pip:
pip install django-redis-cache
In the settings.py file add caches:
CACHES = {
'default': {
'BACKEND': 'django_redis.cache.RedisCache',
'LOCATION': 'redis://localhost:6379/1',
'OPTIONS': {
'CLIENT_CLASS': 'django_redis.client.DefaultClient',
}
}
}
Django Debug Toolbar is a debugging package used to get the debug information of the current request or response. It will help developers in debugging the application in a seamless manner.
Prerequisites:
- Python 3.7, 3.8, 3.9, 3.10 and 3.11.
- Django 2.2, 3.0, 3.1, 3.2, 4.0 and 4.1.
Features:
- Configurable panel view of debugging information. These panels can be expanded to get a detailed overview.
- It shows all SQL queries performed and also provides performance metrics.
Configuration:
Installing the debug toolbar using pip:
pip install django-debug-toolbar
In the settings.py file add installed APSS, middleware, and internal IPS:
INSTALLED_APPS = [
# Other installed apps
'debug_toolbar',
]
MIDDLEWARE = [
# Other middleware
'debug_toolbar.middleware.DebugToolbarMiddleware',
]
INTERNAL_IPS = [
# Local host
'127.0.0.1',
]
5. Django Extensions
Django extensions is a package having a collection of useful extensions for Django. It provides wide range of useful extensions to improve overall productivity.
Prerequisites:
Features:
- Shell_plus provides an enhanced shell feature with preloaded files and variables.
- Show_urls shows all URL patterns defined in the application.
- Assists job scheduling but cron is still required.
Configuration:
Installing the extensions using pip:
pip install django-extensions
In the settings.py file add installed apps:
INSTALLED_APPS = [
# Other installed apps
'django_extensions',
]
Django crispy forms help manage django forms in your application. It provides an easy and consistent way to render forms in your application. It uses form templates that make it easy and quick to manage forms while limiting the code to a single line making the coding part more readable and easy.
Prerequisites:
- Python 3.7 and higher.
- Django 3.2 and higher.
Features:
- FormHelper class helps define the forms rendering behavior.
- Support frontend frameworks like Bootstrap, Tailwind, Bulma, and Foundation.
- Enhance form design by implementing CSS classes to each field’s label and the complete form.
Configuration:
Installing the crispy forms using pip:
pip install django-crispy-forms
In the settings.py file add installed apps and the crispy template pack:
INSTALLED_APPS = [
# Other installed apps
'crispy_forms',
]
CRISPY_TEMPLATE_PACK = 'bootstrap4'
7. Django Haystack
Django Haystack is used to provide modular/unified search features to Django applications. You can add different search backends (such as ElasticSerch, Whoosh, etc.) without having to modify the code.
Prerequisites:
Features:
- Provide strong search functionality for your application.
- Features like spelling suggestions, faceting, and highlighting are also provided.
Configuration:
Installing the haystack using pip:
pip install django-haystack
In the settings.py file add installed apps and haystack connections:
INSTALLED_APPS = [
# Other installed apps
'haystack',
]
HAYSTACK_CONNECTIONS = {
'default': {
'ENGINE': 'haystack.backends.elasticsearch5_backend.Elasticsearch5SearchEngine',
'URL': 'http://localhost:9200/',
'INDEX_NAME': 'haystack',
},
}
Django Cors Headers framework is used to enable Cross-Origin Resource Sharing(CORS) headers in your web application. CORS is a sharing mechanism which helps clients interact with APIs hosted on a different domain.
Prerequisites:
- Python 3.8 to 3.12 support.
- Django 3.2 to 4.2 supported.
Features:
- Gives Javascript in your browser to make requests.
- Required while making cross-domain requests from a web application.
Configuration:
Installing the cors headers using pip:
pip install django-cors-headers
In the settings.py file add installed apps, middleware, and cors origin allowance:
INSTALLED_APPS = [
# Other installed apps
'corsheaders',
]
MIDDLEWARE = [
# Other middleware
'corsheaders.middleware.CorsMiddleware',
]
CORS_ORIGIN_ALLOW_ALL = True
9. Django Guardian
Django Guardian is an important package within Django used to provide permissions to per-objects for Django models. It is applied on top of Django’s authorization backend.
Prerequisites:
Features:
- Using Django Guardian you can create custom permissions and give them to specific users or groups.
- Django Guardian ensures that you have control of every bit of data.
- The feature of revoking permission from a specific user or group is also present.
Configuration:
Installing the guardian using pip:
pip install django-guardian
In the settings.py file add installed apps and authentication backends:
INSTALLED_APPS = [
# Other installed apps
'guardian',
]
AUTHENTICATION_BACKENDS = [
'django.contrib.auth.backends.ModelBackend',
'guardian.backends.ObjectPermissionBackend',
]
10. Django Filter
Django filter is a reusable Django application allowing you to add dynamic QuerySet filtering declaratively from URL parameters.
Prerequisites:
- Latest version of Django.
- All versions of Python.
- The latest version of Django REST Framework.
Features:
- Django Filter is a mature and stable package.
- It used a two-part CalVer versioning scheme.
- Its API can perform functions very similar to ModelForms.
- It can be used to create filter interfaces also.
Configuration:
Installing the filter using pip:
pip install django-filter
In the settings.py file add installed apps:
INSTALLED_APPS = [
# Other installed apps
'django_filters',
]
Django Packages vs. Modules
Many times we use the term package and modules synonymously, but it is important to know the difference between the two terms. A module is a simple Python file having a collection of objects, functions, and variables and saved with a .py extension.
A package on the other hand is a kind of a directory having a collection of modules. Each package has a __init__.py file that helps the interpreter identify it as a package. The package has to be used by explicitly mentioning the package dependency in your code.
Usage of Django helps make your project more manageable, scalable, and reusable and adds an overall value to the project. In this article, we’ll be going through the top 10 Django packages for fast and easy development of your project. Acing these packages will give a great kickstart to your project and career as a Python developer.
Conclusion
The learning and development using Django does not end with these packages. As a developer, you need to understand the use case of each package and choose a package accordingly to fit your needs and ease of development. The above-mentioned packages provide services like storage management, error monitoring, API generation, permissions, authentication, and debugging. Apart from these, other packages provide other functionalities. You should know these packages and use them wherever needed to simplify the process workflow.
FAQs on Top Django Packages
1. What are the top Django Packages?
Here are some of the top Django packages that every developer should know:
- Django REST Frameworks
- Django Allauth
- Django Redis Cache
- Django Debug Toolbar
- Django Extensions
2. What does MVC mean when we define Django?
MVC or Model-View-Controller adds a layer of abstraction between the user and software such that ony relevant details are available to the end user.
3. Can Django be used with preexisting databases?
Yes, Django can be integrated smoothly with prerexisting legacy databases and has several packages that support for this integration.
Share your thoughts in the comments
Please Login to comment...