The DOM stands for ‘Document Object Model’, it’s a programming interface for HTML (Hypertext Markup Language) and XML (Extensible Markup Language) documents. DOM represents the structure of a document which means a hierarchical tree-like structure, where each existing element in the document are represented as a node in the tree. It also provides properties and methods, including dynamically structured to manipulate the content and styling of Web Pages by interacting with nodes that allow developer changes in the document.
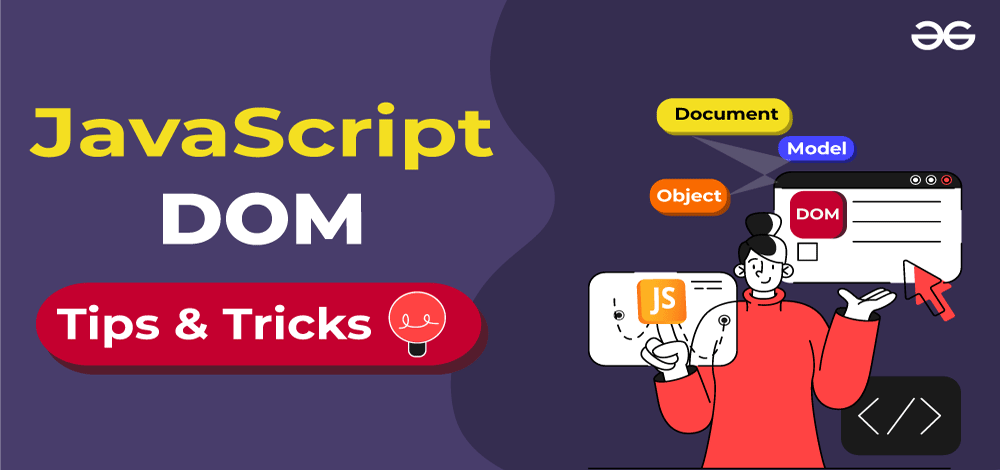
Example:
Imagine you are a developer and building a blog website that includes so many blogs, posts, and comments where users can post the comments and put their reviews. Now you as a developer want to dynamically display the latest comments on the page as well without refreshing the page. So here you can go with the DOM to achieve this shown below.
HTML
< div id = "commentsContainer" >
< h2 >Comments</ h2 >
< ul id = "commentsList" ></ ul >
</ div >
< button id = "postComment" >Post Comment</ button >
|
Javascript
const commentsListElement = document.getElementById( 'commentsList' );
const postCommentButton = document.getElementById( 'postComment' );
postCommentButton.addEventListener( 'click' , function () {
const comment = document.createElement( 'li' );
comment.textContent = 'New comment' ;
commentsListElement.appendChild(comment);
});
|
JavaScript DOM Tips and Tricks
JavaScript is a very powerful programming language for developers that allows us to create dynamic and interactive web pages with full responsiveness. One of the key elements of JavaScript is the Document Object Model (DOM) which represents the structure of the whole HTML document and provides a better way to interact with it programmatically. By utilizing the DOM effectively, it can enhance the developer’s ability to manipulate and controlling of web pages. In this blog, you going to explore 10 JavaScript DOM tips and tricks that every developer should know which will make it easy to analyze features in the context of programming to skill the next level.
1. Selecting DOM Elements
In web pages, when we make changes to elements we need to select them first. If we use JavaScript it makes easier work for the developer because it provides simple methods to do this, like ‘getElementById()’, ‘getElementByClassName()’, and ‘querySelector()’. Let’s go with an example.
Example:
Javascript
const headerElement = document.getElementById( 'header' );
const buttons = document.getElementsByClassName( 'btn' );
const mainElement = document.querySelector( 'main' );
|
2. Changing Element Attributes
This feature allows you to modify element attributes dynamically by using methods, like ‘getAttribute()’, ‘setAttribute()’, and ‘removeAttribute()’ to access, change an element attribute or remove attributes like ‘src’, ‘href’. By using these attribute manipulation methods (‘getAttribute’, ‘setAttribute’, and ‘removeAttribute’) by utilizing you will have the flexibility in accessing, modifying, and removing the attributes of elements dynamically. This could be very useful and essential while adapting the element behaviors, also in changing URLs, or manipulating the custom data attributes which are based on user interaction or in the specific application logic, the following example demonstrates how to get the value, Set the value, and Remove the value of an attribute.
Example:
Javascript
const image = document.getElementById( 'my-image' );
const source = image.getAttribute( 'src' );
console.log(source);
const link = document.querySelector( 'a' );
const button = document.getElementById( 'my-button' );
button.removeAttribute( 'disabled' );
|
3. Handling Form Inputs
Handling form inputs means that working with forms in web development is a common task. Where JavaScript helps you to access and validate the form input fields. It uses the properties like ‘value’ or ‘checked’ to get and set the input field values. Additionally, the ‘submit()’ method can also be used to submit the form as well, it will be more clear by the following example.
Example:
Javascript
const nameInput = document.getElementById( 'name' );
const name = nameInput.value;
console.log(name);
const emailInput = document.getElementById( 'email' );
emailInput.value = 'example@example.com' ;
const form = document.getElementById( 'my-form' );
form.addEventListener( 'submit' , function (event) {
event.preventDefault();
});
|
4. Styling Elements Working with CSS (Cascading Styling Sheet) Classes
The Cascading Styling Sheet (CSS) gives a way to style our web pages, with JavaScript you can easily add or remove CSS classes dynamically. It provides help with such methods as the ‘classList’ property providing methods as ‘add()’, ‘remove()’, ‘toggle()’, and ‘contains()’ which help you to manage the CSS classes. You can also use properties like ‘backgroundColor’, ‘font size’, or ‘display’. This will give you interactive animated effects on your web page.
Example:
Javascript
const element = document.getElementById( 'my-element' );
element.classList.add( 'highlight' );
const button = document.querySelector( '.btn' );
button.classList.remove( 'active' );
const div = document.querySelector( 'div' );
div.classList.toggle( 'visible' );
const box = document.getElementById( 'my-box' );
box.style.backgroundColor = 'blue' ;
const heading = document.querySelector( 'h1' );
heading.style.fontSize = '24px' ;
const image = document.getElementById( 'my-image' );
image.style.display = 'none' ;
|
5. Moving through the DOM
When it’s required to find the specific elements based on their relationships, we need to navigate through DOM tree-like structure. As a developer, you should know these methods like ‘parentNode’, ‘childNode’, ‘nextSibling’, and ‘previousSibling’ which will demonstrate a view for you to move up, down, and sideways through the DOM.
Example:
Javascript
const paragraph = document.getElementById( "my-paragraph" );
const parentElement = paragraph.parentNode;
const container = document.querySelector( '.container' );
const childNodes = container.childNodes;
const heading = document.querySelector( 'h1' );
const nextSibling = heading.nextSibling;
const paragraph = document.getElementById( "my-paragraph" );
const previousSibling = paragraph.previousSibling;
|
6. Efficient DOM Manipulation
It’s very important to reduce unnecessary reflows from the working DOM. To achieve this, we need to perform multiple DOM (Document Object Model) manipulations simultaneously by creating a document fragment and manipulating elements within it, also then appending the fragment to the Document Object Model (DOM). This helps in reducing the number of times the browser is updating the page’s layout as well as improves performance. Let’s explore the following example.
Example:
Javascript
const fragment = document.createDocumentFragment();
for (let i = 0; i < 1000; i++) {
const newElement = document.createElement( 'div' );
newElement.textContent = 'Element ' + i;
fragment.appendChild(newElement);
}
const container = document.getElementById( 'my-container' );
container.appendChild(fragment);
|
Example:
Shopping Cart Updates, when someone as a user adds items to their shopping cart, their developer creates directly manipulating the DOM for each addition instead of this you can create a document fragment that will update it with the new items as well. Once all the items are added to the cart you can append the fragment to the DOM easily into a single operation, which will reduce reflows and enhance the performance.
7. Event Delegation – Filtering Dynamic Content
In the context of the website, there will be a list of items that can be filtered by different categories, here you can use event delegation for handling the filter buttons over there. If you attach a single event listener to the parent container and check the event target, then you could dynamically filter the content without attaching such individual event listeners with there each respective filter button. So, it’s a better way instead of attaching the event listeners to individual elements, you may go with event delegation, which involves attaching a single event listener to the parent element and also using event bubbling to handle events on child elements. This will enhance performance, especially when dealing with a large number of created elements dynamically.
Example:
Javascript
const parentElement = document.getElementById( 'parent' );
parentElement.addEventListener( 'click' , function (event) {
if (event.target.matches( '.child' )) {
console.log( 'Child element clicked!' );
}
});
|
8. Handling Asynchronous Operations
When the developer is working with asynchronous operations, like fetching the data or making AJAX calls, async/wait, or callback functions for ensuring the proper handling of asynchronous flow and preventing blocking of the main thread. This avoids freezing the browser and enhances the smoother user experience. If the developer masters these important tricks, the developer can write more performant, maintainable, and efficient JavaScript code when working with the document object model.
Example:
Javascript
.then(response => response.json())
.then(data => {
console.log(data);
})
. catch (error => {
console.error(error);
});
|
Example:
Dynamic content loading, when we are loading content dynamically from a server, like fetching additional posts from a social media new feed, you can use async/wait, or callbacks to handle the asynchronous operations. This feature allows you to display and load new content without any blockage of the main thread.
9. Exploring Browser APIs
While working with DOM manipulation in JavaScript beyond its basics modern web browsers are available that provide many APIs and allow developers to interact with different sorts of aspects and criteria of web pages. Let’s explore two main popular browser APIs – the Geolocation API and the Web Storage API.
A. Geolocation API
This Geolocation API will allow you to retrieve the user’s geographical location, which will enable the developer to create a location-aware web application. Here’s an example for you where this API is supported by the user’s browser using ‘navigator. geolocation’ that will support a ‘getCurrentPosition()’ for retrieving the user’s current position.
Example:
Javascript
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(
function (position) {
const latitude = position.coords.latitude;
const longitude = position.coords.longitude;
},
function (error) {
console.error( 'Error:' , error.message);
}
);
} else {
console.error( 'Geolocation is not supported by this browser.' );
}
|
B. Web Storage API
This Web Storage API is a very useful feature that allows you to store the data locally in the user’s browser by providing the following two mechanisms – ‘local storage’ and ‘sessionStorage’ for persistent and session-based storage respectively.
Example:
Javascript
localStorage.setItem( 'username' , 'John' );
const username = localStorage.getItem( 'username' );
localStorage.removeItem( 'username' );
|
By taking advantage of these such helpful APIs like Geolocation API and the Web Storage API, you can add very interesting and exciting features to your websites which enables the location-based functionalities which will improve the user’s experience as well as a developer go with a smooth implementation, this makes more interactive and personalized web applications.
10. Traversing the DOM
Traversing the Document Object Model (DOM) for a developer is a very essential skill for any JavaScript developer working with DOM manipulation methods. By traversing the specific elements through your DOM tree and accessing elements based on their relationships. Traversing the DOM is an important trick and tip for every developer as he/she can make a selection of efficient elements, can do element manipulation, and has access to navigate the tree by using such methods like ‘parentNode’, ‘childhood’, ‘nextSibling’, and ‘previous sibling’ will allow you to move up, down and sideways within your DOM tree, the developer will iterating over elements, also can do complex DOM manipulations.
Example:
Javascript
const childElement = document.querySelector( 'li' );
const parentElement = childElement.parentNode;
console.log(parentElement);
const parentElement = document.getElementById( 'parent' );
const childElements = parentElement.childNodes;
console.log(childElements);
const heading = document.querySelector( 'h2' );
const nextSibling = heading.nextSibling;
console.log(nextSibling);
const listItem = document.querySelector( 'li' );
const previousSibling = listItem.previousSibling;
console.log(previousSibling);
|
Important Points for JavaScript DOM
- Some points to be noted are that a developer should know about the JavaScript DOM tricks and tips for better performance and more interactive web applications. Developers can use dynamic form interaction that includes a multi-step form to handle the form validation and navigation.
- Developers can use Interactive image galleries to handle the thumbnail clicks. By using event delegation that will attach a single event listener to the gallery container so that the developer can update the main images based on the clicked thumbnails.
- Developers can have updates of Real-time Data in the live chat application or a displaying real-time dashboard data. The developer can use WebSocket API or AJAX request to fetch data from the server for retrieving real-time data, DOM manipulation updates the UI in real-time which provides the user with the latest information without the need of manual page refreshing.
Conclusion
JavaScript DOM is crucial for every web developer to master itself in it who looks for interactive and creates dynamic web pages. The tips and tricks discussed above in this blog will provide a solid foundation to you for working with Document Object Model (DOM) efficiently.
While we access DOM elements, modify the content, manipulate the CSS classes, create the elements and append the elements, handling the events by using event delegation, traversing the DOM, also handling the asynchronous operations, and optimizing the performance, also using the data attributes, and working with various forms, the web developer can unlock the full potential of JavaScript and unleash the web development.
As a delve developer into the world of web development, the mastery of JavaScript’s DOM will become valuable and embrace the spirit of exploration and experimentation, while pushing the boundaries of what you can achieve with the DOM and elevate your development skills to new heights. So, keep exploring and expanding your knowledge to deep dive into the techie’s world of JavaScript’s DOM.
By doing so, you as a developer will be able to captivate your users, deliver seamless interactions, and create web applications that will leave a lasting impression.
FAQs on JavaScript DOM
Q1. What are the top JavaScript DOM Tips and Tricks?
Answer:
- Selecting DOM Elements
- Changing Element Attributes
- Handling Form Inputs
- Styling Elements Working with CSS (Cascading Styling Sheet) Classes
- Moving through the DOM
- Efficient DOM Manipulation
- Event Delegation – Filtering Dynamic Content
- Handling Asynchronous Operations
- Exploring Browser APIs
- Traversing the DOM
Q2. Should I learn DOM before JavaScript?
Answer:
No, It is generally recommended to learn JavaScript before learning the Document Object Model (DOM).
Q3. What to learn after DOM in JavaScript?
Answer:
After learning the DOM in JavaScript, we can learn many frameworks and libraries like React, Angular, and Vue.js. It’s a personal choice of what we want to learn.
Share your thoughts in the comments
Please Login to comment...