Testing FastAPI Application
Last Updated :
06 Dec, 2023
The web framework in Python that is used for creating modern and fast APIs is called FastAPI. Once we have created the FastAPI, there is a need to test if the API is working fine or not according to the requirements. In this article, we will discuss the various ways to test the FastAPI application.
Testing FastAPI Application
Below are the methods by which we can test FastAPI applications:
- Using TestClient library
- Using Requests library
Using TestClient Library
The HTTPX-based library which is used to call the asynchronous FastAPI application is known as the TestClient library. In this method, we will see how we can test FastAPI applications using the TestClient library.
Syntax
client = TestClient(app)
def function_name():
response = client.get(“/”)
assert response.status_code == status_code
assert response.json() == {“msg”: “Message_FastAPI”}
Here,
- function_name: It is the name of the function which you want to call while testing.
- status_code: It is the HTTP status code which we want to display to user along with message.
- Message_FastAPI: It is the messages shown to user along with the response.
Example: In this example, we are creating a function which returns a certain message and then we are matching that message with other message defined using TestClient.
Python3
from fastapi import FastAPI
from fastapi.testclient import TestClient
app = FastAPI()
@app .get( "/" )
async def read_main():
return { "msg" : "Welcome to Geeks For Geeks" }
client = TestClient(app)
def test_read_main():
response = client.get( "/" )
assert response.status_code = = 200
assert response.json() = = { "msg" : "Welcome to Geeks For Geeks" }
|
Now, open the terminal and run the following command to test the FastAPI application created.
pytest main.py
Output
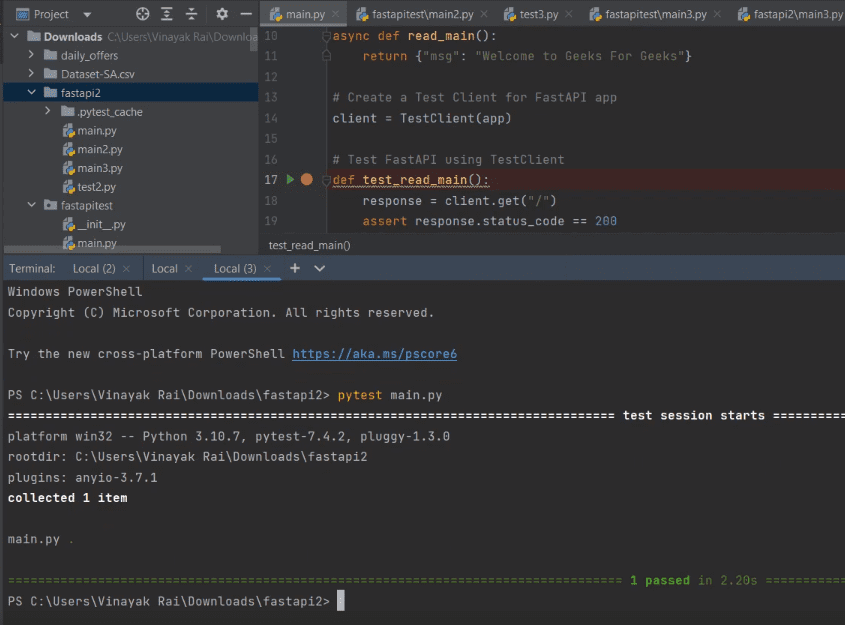
Using Requests Library
The library which is used to send HTTP requests using Python is known as requests library. In this method, we will see how we can test FastAPI applications using requests library. For testing FastAPI applications using requests library, the user needs to create a separate test file.
Syntax
import requests
print(requests.get(“http://127.0.0.1:8000/”).json())
Example: In this example, we have created a function with two messages, msg and test_msg, which we are checking if these messages are same, then ‘Test Passed’ message is displayed, else ‘Test Failed’ message is displayed.
Python3
from fastapi import FastAPI
app = FastAPI()
@app .get( "/" )
async def read_main():
msg = "Welcome to Geeks For Geeks"
test_msg = "Welcome to Geeks For Geeks"
if msg = = test_msg:
return { "msg" : "Test Passed" }
else :
return { "msg" : "Test Failed" }
|
Now, open the terminal and run the following command to run your FastAPI application. This command will also let the app to reload in case of any changes made in app.
uvicorn main:app --reload
test.py: This file is used created to test the above main file program.
Output
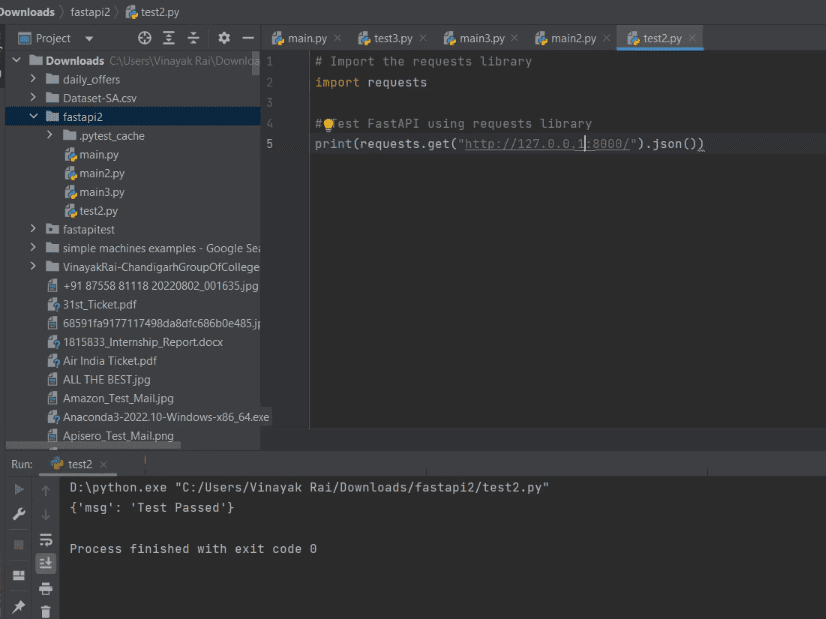
Share your thoughts in the comments
Please Login to comment...