Making HTTP Requests from a FastAPI Application to an External API
Last Updated :
26 Mar, 2024
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.7+ based on standard Python type hints. It is designed to be easy to use, efficient, and reliable, making it a popular choice for developing RESTful APIs and web applications. Your FastAPI server may need to fetch data from other external APIs, to perform data aggregations and comparisons. So let’s learn how to send HTTP requests to an external API from a FastAPI application.
Sending HTTP Requests from FastAPI to External APIs
Create a file main.py with the following code:
Python3
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def root():
return {'message': 'Welcome to GeeksforGeeks!'}
Make a request to an external API
Now we will call another API inside our FastAPI application. Currently, we are using a simple test API for demonstration purposes.
https://jsonplaceholder.typicode.com/users
The data returned from the API looks like this:
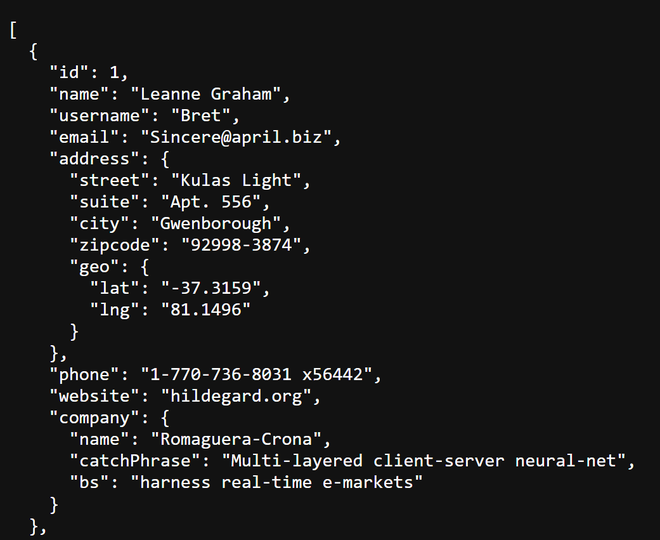
external API call response
Calling an external API from our FastAPI server:
- Synchronous call
- Asynchronous call
Call external api from Web API Synchronously
If the API call is synchronous, it means that the code execution will block (or wait) for the API call to return before continuing. We will use the requests module in python, to make synchronous call to the external test API.
Open the terminal and use the following command to install the requests module.
pip install requests
Open the main.py file that we had created before. Here we will import the requests module.
import requests
Now we will make another endpoint: /get_firstuser . This will call the test API and synchronously return the name and username of the first user in the response. So our main.py file looks something like this.
Python3
from fastapi import FastAPI
import requests
app = FastAPI()
@app.get("/")
def root():
return {'message': 'Welcome to GeeksforGeeks!'}
@app.get('/get_firstuser')
def first_user():
api_url = "https://jsonplaceholder.typicode.com/users"
all_users = requests.get(api_url).json()
user1 = all_users[0]
name = user1["name"]
email = user1["email"]
return {'name': name, "email": email}
Call external api from Web API Asynchronous
If the API call is asynchronous, the program doesn’t wait for the response. Instead, it continues executing other code while the API call is in progress. When the response is ready, the program will handle it. We will use httpx module in python to make the asynchronous API call.
Open the terminal and use the following command to install the httpx module.
pip install httpx
Open the main.py file that we had created before. Here we will import the httpx module.
import httpx
Now we will make another endpoint: /get_seconduser. This will asynchronously return the name and username of the second user in the response. So our main.py file looks something like this.
Python3
from fastapi import FastAPI
import requests
import httpx
app = FastAPI()
@app.get("/")
def root():
return {'message': 'Welcome to GeeksforGeeks!'}
@app.get('/get_firstuser')
def first_user():
api_url = "https://jsonplaceholder.typicode.com/users"
all_users = requests.get(api_url).json()
user1 = all_users[0]
name = user1["name"]
email = user1["email"]
return {'name': name, "email": email}
@app.get('/get_seconduser')
async def second_user():
api_url = "https://jsonplaceholder.typicode.com/users"
async with httpx.AsyncClient() as client:
response = await client.get(api_url)
all_users = response.json()
user2 = all_users[1]
name = user2["name"]
email = user2["email"]
return {'name': name, "email": email}
Testing the API
Now open the terminal in your directory and run the server with the following command:
uvicorn main:app --reload
Now you can open the browser and call your API at http://localhost:8000. If you enter http://127.0.0.1:8000/get_firstuser, the FastAPI server makes a call to the external test API and returns the name and email of the first user as response.

FastAPI response for first user
If you enter http://127.0.0.1:8000/get_seconduser, you will be get the name and email of the second user as response.

FastAPI response for second user
You can also refer to this article, which explains the benefit of using asynchronous API calls instead of synchronous API calls.
Share your thoughts in the comments
Please Login to comment...