Sum of the series 1 + (1+3) + (1+3+5) + (1+3+5+7) + …… + (1+3+5+7+…+(2n-1))
Last Updated :
27 Sep, 2022
Given a positive integer n. The problem is to find the sum of the given series 1 + (1+2) + (1+2+3) + (1+2+3+4) + …… + (1+2+3+4+…+n), where i-th term in the series is the sum of first i odd natural numbers.
Examples:
Input : n = 2
Output : 5
(1) + (1+3) = 5
Input : n = 5
Output : 55
(1) + (1+3) + (1+3+5) + (1+3+5+7) + (1+3+5+7+9) = 55
Naive Approach: Using two loops get the sum of each i-th term and then add those sum to the final sum.
C++
#include <bits/stdc++.h>
using namespace std;
int sumOfTheSeries( int n)
{
int sum = 0;
for ( int i = 1; i <= n; i++) {
int k = 1;
for ( int j = 1; j <= i; j++) {
sum += k;
k += 2;
}
}
return sum;
}
int main()
{
int n = 5;
cout << "Sum = "
<< sumOfTheSeries(n);
return 0;
}
|
Java
import java.util.*;
class GFG {
static int sumOfTheSeries( int n)
{
int sum = 0 ;
for ( int i = 1 ; i <= n; i++)
{
int k = 1 ;
for ( int j = 1 ; j <= i; j++)
{
sum += k;
k += 2 ;
}
}
return sum;
}
public static void main(String[] args)
{
int n = 5 ;
System.out.println( "Sum = " +
sumOfTheSeries(n));
}
}
|
Python3
def sumOfTheSeries(n):
sum = 0
for i in range ( 1 , n + 1 ):
k = 1
for j in range ( 1 , i + 1 ):
sum + = k
k + = 2
return sum
n = 5
print ( "Sum =" , sumOfTheSeries(n))
|
C#
using System;
class GFG {
static int sumOfTheSeries( int n)
{
int sum = 0;
for ( int i = 1; i <= n; i++) {
int k = 1;
for ( int j = 1; j <= i; j++) {
sum += k;
k += 2;
}
}
return sum;
}
public static void Main()
{
int n = 5;
Console.Write( "Sum = " +
sumOfTheSeries(n));
}
}
|
php
<?php
function sumOfTheSeries( $n )
{
$sum = 0;
for ( $i = 1; $i <= $n ; $i ++) {
$k = 1;
for ( $j = 1; $j <= $i ; $j ++) {
$sum += $k ;
$k += 2;
}
}
return $sum ;
}
$n = 5;
echo "Sum = "
. sumOfTheSeries( $n );
?>
|
Javascript
<script>
function sumOfTheSeries(n)
{
let sum = 0;
for (let i = 1; i <= n; i++) {
let k = 1;
for (let j = 1; j <= i; j++) {
sum += k;
k += 2;
}
}
return sum;
}
let n = 5;
document.write( "Sum = " + sumOfTheSeries(n));
</script>
|
Output:
Sum = 55
Time complexity: O(n2)
Auxiliary space: O(1)
Efficient Approach:
Let an be the n-th term of the given series.
an = (1 + 3 + 5 + 7 + (2n-1))
= sum of first n odd numbers
= n2
Refer this post for proof of the above formula. Now,
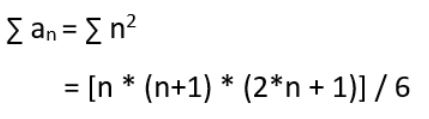
Refer this post for proof of the above formula.
C++
#include <bits/stdc++.h>
using namespace std;
int sumOfTheSeries( int n)
{
return (n * (n + 1) / 2) * (2 * n + 1) / 3;
}
int main()
{
int n = 5;
cout << "Sum = " << sumOfTheSeries(n);
return 0;
}
|
Java
import java.io.*;
class GfG {
static int sumOfTheSeries( int n)
{
return (n * (n + 1 ) / 2 ) *
( 2 * n + 1 ) / 3 ;
}
public static void main (String[] args)
{
int n = 5 ;
System.out.println( "Sum = " +
sumOfTheSeries(n));
}
}
|
Python3
def sumOfTheSeries( n ):
return int ((n * (n + 1 ) / 2 ) *
( 2 * n + 1 ) / 3 )
n = 5
print ( "Sum =" , sumOfTheSeries(n))
|
C#
using System;
class GfG {
static int sumOfTheSeries( int n)
{
return (n * (n + 1) / 2) *
(2 * n + 1) / 3;
}
public static void Main()
{
int n = 5;
Console.Write( "Sum = " +
sumOfTheSeries(n));
}
}
|
PHP
<?php
function sumOfTheSeries( $n )
{
return ( $n * ( $n + 1) / 2) *
(2 * $n + 1) / 3;
}
$n = 5;
echo "Sum = "
. sumOfTheSeries( $n );
?>
|
Javascript
<script>
function sumOfTheSeries(n)
{
return (n * (n + 1) / 2) *
(2 * n + 1) / 3;
}
let n = 5;
document.write( "Sum = " +
sumOfTheSeries(n));
</script>
|
Output:
Sum = 55
Time complexity: O(1)
Auxiliary space: O(1)
Share your thoughts in the comments
Please Login to comment...