Sum of array Elements without using loops and recursion
Last Updated :
13 Mar, 2023
Given an array of N elements, the task is to find the Sum of N elements without using loops(for, while & doWhile) and recursion.
Examples:
Input: arr[]={1, 2, 3, 4, 5}
Output: 15
Input: arr[]={10, 20, 30}
Output: 60
Approach: Unconditional Jump Statements can be used to solve this problem.
Unconditional Jump Statements:
Jump statements interrupt the sequential execution of statements, so that execution continues at a different point in the program. A jump destroys automatic variables if the jump destination is outside their scope. There are four statements that cause unconditional jumps in C: break, continue, goto, and return.
To solve this particular problem, goto statement can be useful.
goto Statement:
The goto statement is a jump statement which is sometimes also referred to as unconditional jump statement. The goto statement can be used to jump from anywhere to anywhere within a function.
Syntax:
Syntax1 | Syntax2
----------------------------
goto label; | label:
. | .
. | .
. | .
label: | goto label;
In the above syntax, the first line tells the compiler to go to or jump to the statement marked as a label. Here label is a user-defined identifier which indicates the target statement. The statement immediately followed after ‘label:’ is the destination statement. The ‘label:’ can also appear before the ‘goto label;’ statement in the above syntax.
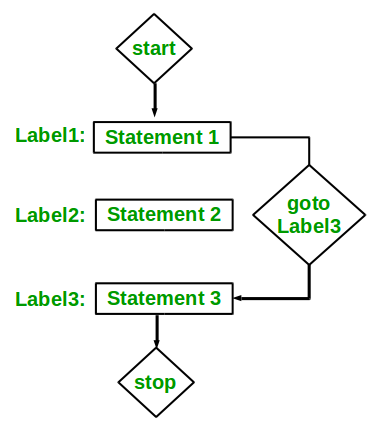
Below is the implementation of the above approach:
C++
#include <iostream>
using namespace std;
int operate( int array[], int N)
{
int sum = 0, index = 0;
label:
sum += array[index++];
if (index < N) {
goto label;
}
return sum;
}
int main()
{
int N = 5, sum = 0;
int array[] = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
cout << sum;
}
|
C
#include <stdio.h>
int operate( int array[], int N)
{
int sum = 0, index = 0;
label:
sum += array[index++];
if (index < N) {
goto label;
}
return sum;
}
int main()
{
int N = 5, sum = 0;
int array[] = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
printf ( "%d" , sum);
}
|
Java
class GFG
{
static int operate( int array[], int N)
{
int sum = 0 , index = 0 ;
while ( true )
{
sum += array[index++];
if (index < N)
{
continue ;
}
else
{
break ;
}
}
return sum;
}
public static void main(String[] args)
{
int N = 5 , sum = 0 ;
int array[] = { 1 , 2 , 3 , 4 , 5 };
sum = operate(array, N);
System.out.print(sum);
}
}
|
Python3
def operate(array, N) :
Sum , index = 0 , 0
while ( True ) :
Sum + = array[index]
index + = 1
if index < N :
continue
else :
break
return Sum
N, Sum = 5 , 0
array = [ 1 , 2 , 3 , 4 , 5 ]
Sum = operate(array, N)
print ( Sum )
|
C#
using System;
class GFG
{
static int operate( int [] array, int N)
{
int sum = 0, index = 0;
label:
sum += array[index++];
if (index < N)
{
goto label;
}
return sum;
}
public static void Main()
{
int N = 5, sum = 0;
int [] array = { 1, 2, 3, 4, 5 };
sum = operate(array, N);
Console.Write(sum);
}
}
|
PHP
<?php
function operate( $array , $N )
{
$sum = 0;
$index = 0;
label:
$sum += $array [ $index ++];
if ( $index < $N )
{
goto label;
}
return $sum ;
}
$N = 5;
$array = array (1, 2, 3, 4, 5);
echo operate( $array , $N );
?>
|
Javascript
<script>
function operate(array, N)
{
let sum = 0, index = 0;
while ( true )
{
sum += array[index++];
if (index < N)
{
continue ;
}
else
{
break ;
}
}
return sum;
}
let N = 5, sum = 0;
let array = [ 1, 2, 3, 4, 5 ];
sum = operate(array, N);
document.write(sum);
</script>
|
Time complexity: O(N) where N is size of given array
Auxiliary space: O(1)
Share your thoughts in the comments
Please Login to comment...