GraphQL is a powerful open-source Query Language for APIs. It is most commonly known for its single endpoint query which allows the user to define a single endpoint to fetch all the information needed. Schemas in GraphQL are the blueprints for its APIs and define what the request-response structure would look like. In this article, we will learn about GraphQL Schemas, and we will create some ourselves.
What is GraphQL Schema
A GraphQL Schema serves as a blueprint for the API that defines what data can be queried from the server, and what are the different types of that data. They define a contract of API between a server and a client.
A GraphQL Schema is divided into basic components like below:
1. Types
The types of fields that we can fetch or query on the server. It represents the different types available in GraphQL that we can specify on the various fields to be queried upon using GraphQL API.
Examples:
Int - integer data type
String - string data type
Boolean - boolean data type
2. Fields
The different properties that can be queries upon in the API. They represent the different properties available that can be queries upon the GraphQL API.
Example:
In the below example, “id” and “username” are the fields which we can query from the server
type User {
id: ID!
username: String!
}
3. Queries
The type for the GraphQL Query API endpoint that gets called to fetch data from the server. They are the entrypoints for fetching data from the server, and the Query type is defined at the root of the GraphQL query
Example:
type Query {
getUser(id: ID!): User
}
4. Mutations
The type for the operations that define a change in the data. They are the root types for the operations that modify the data on the server.
Example:
type Mutation {
createUser(username: String!): User
}
5. Subscriptions
The types for the operations that allows clients to get real-time updates from the server. They are the root types for the operations from which the clients can receive real-time updates from the server.
Example:
type Subscription {
userAdded: User
}
How to Write a GraphQL Schema?
Now let’s create a GraphQL Schema inside a GraphQL Server to server requests to a GraphQL API
Step 1: Creating a Node.js Server
We will create a basic node.js server, and in later steps, we will create a GraphQL API with a schema inside the server.
First, run the below command to initialise an npm project
npm init -y
Now, install the dependencies for creating an Apollo Server using the below command –
npm i apollo-server
Now, create a new file called server.js, where we will write the logic of creating a GraphQL server, and we will also add the GraphQL Schema Types in the same file. The final project structure should look like below
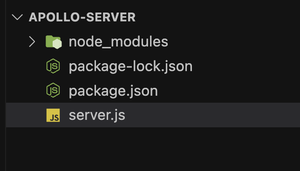
Step 2: Defining Schema Type
In this step, we will define the GraphQL Schema Type of the User and the Query, so when the endpoint gets hit, the end user can ask the query to return some or all of the types in the response data. In this example, we will define a user with types of just id, name, and age, to keep it simple.
Javascript
const typeDefs = gql`
type User {
id: ID!
name: String!
age: Int!
}
type Query {
getUser(id: ID!): User
}
`;
|
Step 3: Creating a Resolver to Serve the Requests
In this step, we will create a GraphQL resolver to serve the requests that are hit to the GraphQL endpoint. Since in the above step, we defined the type for only one query `getUser`, we will create the resolver for the same. And we will just return a static user object with name “John Doe”, and age as “20”, as we are not creating a DB connection here.
Javascript
const resolvers = {
Query: {
getUser: (_, { id }) => {
return { id, name: 'John Doe' , age: 28 };
},
},
};
|
Step 4: Creating and Starting the Apollo Server
In this step, we will create and start our Apollo Server on port 4000.
Javascript
const server = new ApolloServer({ typeDefs, resolvers });
server.listen().then(({ url }) => {
console.log(`Server running at ${url}`);
});
|
Step 5: Integrating the Above Code Together in a Single File
In this step, we will put all the above pieces of code together into a single file, server.js, that we created in the beginning. Let’s start our server by running the below command –
node server.js
You will see the output of the server running –
Server running at http://localhost:4000/
Now, open the graphQL playground here, and execute the below query to fetch the data on the basis of provided schema
Javascript
query {
getUser(id: "1" ) {
id
name
age
}
}
|
Output:
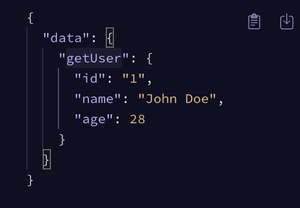
Conclusion
In this article, we learned about the GraphQL Schema and its various components. We also created our own GraphQL server with a Schema inside and learned how the Schema defines an API contract between the client and server. Through its key components such as types, fields, queries, mutations, and subscriptions, GraphQL Schema allows developers to precisely specify the data types, properties, and operations available for interaction.
Share your thoughts in the comments
Please Login to comment...