Scalar Types in GraphQL Schema
Last Updated :
01 Dec, 2023
GraphQL is a powerful open-source Query Language for APIs. In 2012 it was first developed by a team of developers in Facebook and then it was later made public to the general people by making it open-source. Now it is being maintained by the GraphQL community.
GraphQL is most commonly known for its single endpoint query which allows the user to define a single endpoint to fetch all the information needed. The benefit of using single endpoint queries is that they help the user in reducing fetching too much data or less amount of data than required. GraphQL datatypes are lists, objects, enums, interfaces, and primitive types which are also known as scalar types(built-in primitive types).
Let us see in this article, how the different scalar types can be used in the GraphQL schema.
Prerequisites:
Below are the prerequisites that we need to install before executing the GraphQL query on our laptop or desktop.
Scalar Types in GraphQL
Scalar types are used to represent simple values. GraphQL query is built using GraphQL Shema. Scalar types are used as building blocks in defining the GraphQL schema. Scalar types are atomic as they hold a single value and it does not contain any subfields like collections or lists. The response of the GraphQL query is represented in a tree-like structure where the scalar type forms the leaf nodes in the tree.
Common Built-in Scalar Types
- Int: Represents a 32-bit signed integer. For Example: 55
- Float: Represents a floating-point value. For Example: 15.6
- String: Represents a sequence of characters. For Example: “program”
- Boolean: Represents a true or false value. For Example: True or False
- ID: Represents a unique identifier, often used for fetching specific objects. For Example: userID=1001
We can also define custom scalar types when we want to represent specific data formats, such as dates, timestamps, or other custom atomic values.
Using Built-in Scalar Types
In the below example, create a GraphQL schema to define basic information about a person.
Step 1: Define the Schema
- To define the GraphQL schema, Create a file and save it with .graphql extension.
- For example we can name the file as schema.graphql.
Javascript
type Query {
person: Person
}
type Person {
studentID :ID
name: String
age: Int
isStudent: Boolean
weight: Float
}
|
Step 2: Implement Resolvers
Let’s set up the server, save the file as server.js and we will implement resolvers.
Javascript
const express = require( 'express' );
const { graphqlHTTP } = require( 'express-graphql' );
const { buildSchema } = require( 'graphql' );
const fs = require( 'fs' );
const path = require( 'path' );
const schema = buildSchema(fs.readFileSync(path.join(__dirname, 'schema.graphql' ), 'utf8' ));
const root = {
person: () => {
return { studentID:1,name: 'John Doe' , age: 15, isStudent: true ,weight:55.5};
},
};
const app = express();
app.use(
'/graphql' ,
graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true ,
})
);
app.listen(4000, () => {
});
|
Step 3: Start the Server
- To start the server run the below command in the terminal.
node server.js

Step 4: Test the query in GraphiQL interface.
- To test the query, execute the below query in the GraphiQL interface.
{
person {
studentID
name
age
isStudent
weight
}
}
Output:
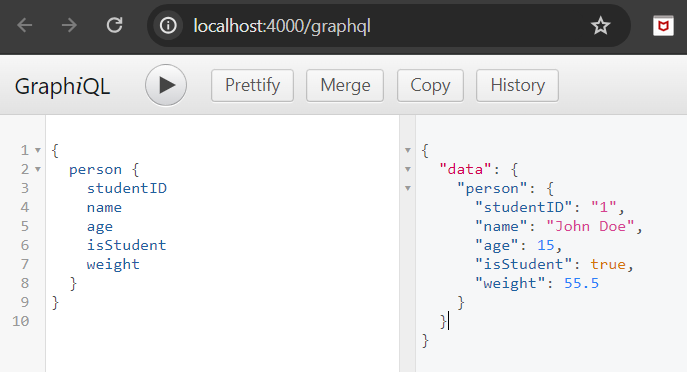
Output
Custom Scalar Type
In this example, we will create a custom scalar type to represent dates. We’ll define a task type with a custom date scalar type.
Step 1: Define the Schema
- To define the GraphQL schema, Create a file and save it with .graphql extension.
- For example we can name the file as schema.graphql.
Javascript
scalar Date
type Query {
task: Task
}
type Task {
title: String
dueDate: Date
}
|
Step 2: Implement Resolvers
Let’s set up the server, save the file as server.js and we will implement resolvers.
Javascript
const express = require( 'express' );
const { graphqlHTTP } = require( 'express-graphql' );
const { buildSchema,GraphQLScalarType, Kind } = require( 'graphql' );
const fs = require( 'fs' );
const path = require( 'path' );
const DateType = new GraphQLScalarType({
name: 'Date' ,
description: 'Custom Date scalar type' ,
parseValue(value) {
return new Date(value);
},
serialize(value) {
return value.toISOString();
},
parseLiteral(ast) {
if (ast.kind === Kind.STRING) {
return new Date(ast.value);
}
return null ;
},
});
const schema = buildSchema(fs.readFileSync(path.join(__dirname, 'schema.graphql' ), 'utf8' ));
schema._typeMap.Date = DateType;
const root = {
task: () => {
return {
title: 'Complete the project' ,
dueDate: new Date( '2023-11-30T08:00:00.000Z' ),
};
},
};
const app = express();
app.use(
'/graphql' ,
graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true ,
})
);
const PORT = process.env.PORT || 4000;
app.listen(PORT, () => {
console.log(`GraphQL server is running on http:
});
|
Step 3: Start the Server
To start the server run the below command in the terminal
node server.js

Step 4: Test the query in GraphiQL interface.
To test the query, execute the below query in the GraphiQL interface.
{
task {
title
dueDate
}
}
Output:
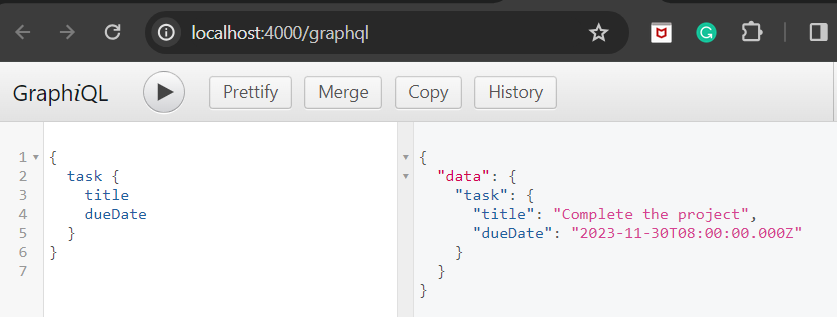
Output
Conclusion
GraphQL is a powerful API query language known for its single endpoint, preventing unnecessary data fetching. Scalar types, representing basic values, form the core of GraphQL schemas. The article demonstrated using common scalar types and creating a custom one for dates, showcasing GraphQL’s flexibility. Its modular design and community support make it a go-to for efficient API development.
Share your thoughts in the comments
Please Login to comment...