JQuery Integration in GraphQL
Last Updated :
04 Apr, 2024
jQuery integration in GraphQL refers to the practice of using jQuery, a popular JavaScript library that interacts with GraphQL servers. This integration simplifies the process of sending and receiving data asynchronously, which is crucial for building dynamic web applications.
In this article, We will learn about What is JQuery Integration in GraphQL, their Prerequisites and How to Setting Up the Server and Client.
What is JQuery Integration in GraphQL?
- jQuery integration in GraphQL refers to the use of jQuery, a popular JavaScript library, to interact with a GraphQL server.
- jQuery simplifies the process of making asynchronous requests to the server, which is a key aspect of working with GraphQL, as GraphQL queries are typically sent over HTTP as POST requests.
- In a jQuery integration, we would typically use jQuery’s AJAX function to send GraphQL queries to the server and process the responses.
- This allows us to build dynamic web applications that fetch data from a GraphQL server without needing to reload the entire page.
Prerequisites for Integrating jQuery with GraphQL
Before understanding the integration of jQuery with GraphQL, ensure we have the following prerequisites:
- Familiarity with GraphQL Principles: It’s essential to have a solid understanding of GraphQL fundamentals, including schemas, queries, mutations, and resolvers. This foundational knowledge will aid in effectively interacting with GraphQL endpoints.
- Proficiency in jQuery and Asynchronous Requests: To work effectively with GraphQL servers, it’s important to have a good understanding of jQuery and AJAX. This knowledge helps in sending and receiving data asynchronously, making communication with GraphQL servers much simpler.
- Setup of a GraphQL Server: Set up a GraphQL server with a well-defined schema and resolver functions. Ensure the server is equipped with middleware to handle GraphQL queries effectively.
- Exploration of GraphQL Endpoints: Explore your GraphQL server using tools like GraphQL or similar graphical interfaces. This exploration will help us to understand the available queries, mutations, and their corresponding responses. Additionally, it provides insights into request headers and parameters, helping in easy integration with jQuery.
Setting Up the Server
Setting up the server for GraphQL integration involves a series of straightforward steps:
Step 1: Install Dependencies
Begin by ensuring we have all the necessary dependencies installed for our project. Navigate to our desired directory and create a new folder named jquery-server-app.
Step 2: Schema Creation
Inside the jquery-server-app folder, create a file named schema.graphql. This file will define the structure of our GraphQL queries. Add the following code to schema.graphql:
type Query {
greeting: String
sayHello(name: String!): String
}
This schema defines two queries: greeting and sayHello. The sayHello query accepts a name parameter and returns a string.
Step 3: Resolver Implementation
Next, create a file named resolvers.js within the jquery-server-app folder. In this file, define the resolver functions for our queries. Here’s an example implementation:
const Query = {
greeting: () => 'Hello GraphQL From TutorialsPoint !!',
sayHello: (root, args, context, info) => `Hi ${args.name} GraphQL server says Hello to you!!`
};
module.exports = { Query };
In this code snippet, greeting and sayHello are resolver functions. The sayHello resolver accesses the name parameter passed in the query arguments to personalize the greeting.
Step 4: Run the Server
Create a file named server.js in the jquery-server-app folder. Refer to the Environment Setup Chapter for guidance on setting up this file. Once configured, execute the command npm start in our terminal to start the server. The server will run on port 9000.
Testing with GraphQL
To test the functionality of our GraphQL server, open our browser and navigate to http://localhost:9000/graphiql. Here, we can interactively query our server. Try entering the following query:
{
greeting,
sayHello(name: "Mohtashim")
}
We should receive a response similar to:
{
"data": {
"greeting": "Hello GraphQL From TutorialsPoint !!",
"sayHello": "Hi Mohtashim GraphQL server says Hello to you!!"
}
}
Setting Up the Client
Step 1: Create a New Folder
To begin, let’s create a new folder named jquery-client-app outside the current project folder. This folder will contain all the client-side files for our jQuery application.
Step 2: Create an HTML Page
Now, let’s create an HTML page named index.html within the jquery-client-app folder. This page will serve as the entry point for our jQuery integration. Here’s the code for index.html:
<!DOCTYPE html>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
<script>
$(document).ready(function() {
$("#btnSayhello").click(function() {
const name = $("#txtName").val();
console.log(name);
$("#SayhelloDiv").html('loading....');
// Update the message for the Say Hello button
$("#SayhelloDiv").html("<h1>Hello, I'm a robot. How can I help you?</h1>");
});
$("#btnGreet").click(function() {
$("#greetingDiv").html('loading....');
// Update the message for the Greet button
$("#greetingDiv").html("<h1>Hi, I'm doing your work.</h1>");
});
});
</script>
</head>
<body>
<h1>JQuery Client</h1>
<hr />
<section>
<button id="btnGreet">Greet</button>
<br /> <br />
<div id="greetingDiv"></div>
</section>
<br /> <br /> <br />
<hr />
<section>
Enter a name: <input id="txtName" type="text" value="john" />
<button id="btnSayhello">Say Hello</button>
<div id="SayhelloDiv"></div>
</section>
</body>
</html>
Output:
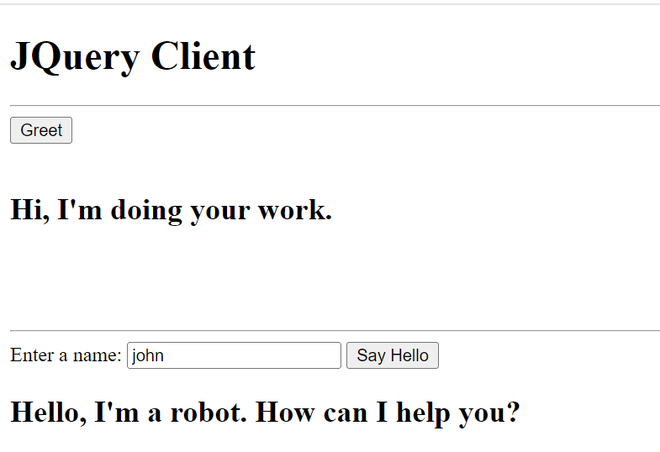
Html Page
Conclusion
Overall, integrating jQuery with GraphQL offers a powerful combination for web developers. By combining the simplicity and versatility of jQuery with the flexibility of GraphQL, developers can create interactive and efficient web applications. Understanding the fundamentals of both jQuery and GraphQL is essential for successfully integrating the two technologies, enabling developers to build responsive and dynamic web experiences.
Share your thoughts in the comments
Please Login to comment...