SAP ABAP | Understanding Object
Last Updated :
30 Nov, 2023
SAP ABAP (Advanced Business Application Programming) is a high-level programming language created by the German software company SAP SE. ABAP is primarily used for developing and customizing applications within the SAP ecosystem, which includes enterprise resource planning (ERP) systems and other business software solutions. C++ is used to implement the ABAP kernel. A procedural and object-oriented programming model are both supported by the hybrid programming language ABAP.
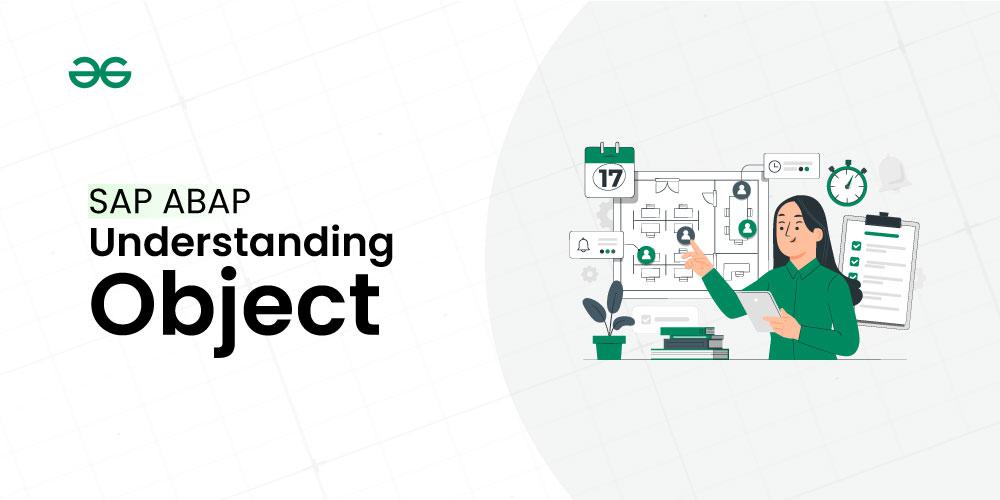
SAP ABAP | Understanding Object
What is an Object in SAP ABAP:
An object is a type of variable with unique traits and actions. An object’s features or attributes are used to describe its state, whereas behaviors or methods indicate the actions performed by an object in SAP ABAP. An object is a pattern or a class instance. It represents a physical entity, such as a person, or a programming entity, such as variables and constants. Accounts and students are two instances of real-world things. However, computer hardware and software are instances of programming entities.
Properties of Objects in SAP ABAP:
- There is a state.
- Has a distinct identity.
- The behavior may or may not be displayed.
For efficient and flexible programming in SAP ABAP (Advanced Business Application Programming), an understanding of objects is essential. The foundation of the Object-Oriented Programming (OOP) paradigm is objects, which give code a means to be organized and structured.
Creating an Object in SAP ABAP:
The following steps are typically included in the production of an object:
- Making a reference variable with a class reference. Which has the following syntax:
- DATA: <object_name> TYPE REF TO <class_name>.
- Making an object out of a reference variable. Which has the following syntax:
- CREATE Object: <object_name>.
Syntax:
REPORT ZDEMO_OBJECT.
CLASS Class1 Definition.
Public Section.
DATA: text1(45) VALUE 'GFG SAP ABAP Objects.'.
METHODS: Display1.
ENDCLASS.
CLASS Class1 Implementation.
METHOD Display1.
Write:/ 'This is the Display method.'.
ENDMETHOD.
ENDCLASS.
START-OF-SELECTION.
DATA: Class1 TYPE REF TO Class1.
CREATE Object: Class1.
Write:/ Class1->text1.
CALL METHOD: Class1->Display1.
Output:
GFG SAP ABAP Objects
This is the Display method.
Example of Creating and Using an Object in SAP ABAP:
CLASS lcl_account DEFINITION.
PUBLIC SECTION.
DATA: account_number TYPE i,
balance TYPE f,
account_holder TYPE string.
METHODS: constructor,
deposit_balance IMPORTING amount TYPE f,
withdraw_balance IMPORTING amount TYPE f,
get_balance RETURNING VALUE(balance) TYPE f.
ENDCLASS.
CLASS lcl_account IMPLEMENTATION.
METHOD constructor.
" Initialize object attributes
ENDMETHOD.
METHOD deposit_balance.
" Add amount to the balance
ENDMETHOD.
METHOD withdraw_balance.
" Subtract amount from the balance
ENDMETHOD.
METHOD get_balance.
" Return the current balance
ENDMETHOD.
ENDCLASS.
DATA(account1) = NEW lcl_account( ).
account1->deposit_balance(1000.00).
WRITE: / 'Account Balance:', account1->get_balance( ).
Functionality of an Object in SAP ABAP:
- Qualities: Identity, Behavior, and State: Three essential properties of an object in SAP ABAP are its state, identity, and behavior. Identity sets one object apart from another, behavior outlines the functions or methods that an object can carry out, and state reflects the data or properties of the object.
- Programming vs Real-World Entities: Objects are entities having properties and actions in the actual world. Objects in programming are representations of real-world entities that let programmers create systems models that closely resemble the real components and interactions.
- Characteristics and Condition: An object’s attributes specify the characteristics or information that make up its state. An object representing an account, for instance, might have properties like the account number, amount, and account holder name in a banking application.
- Object as Data Type Defined by the User: Objects are user-defined data types in SAP ABAP. This implies that programmers have the ability to design custom data types that combine behavior and data into a single unit. This improves the readability, maintainability, and reusability of the code.
- Practical Applications: Objects find practical applications in various SAP scenarios, such as modeling business processes, handling data entities, and facilitating communication between different system components.
- Best Practices: When working with objects in SAP ABAP, adhere to best practices like encapsulation, inheritance, and polymorphism. These principles enhance code maintainability, flexibility, and scalability.
Some Recent Articles Related to SAP ABAP OOPS concepts:
Conclusion:
Understanding objects in SAP ABAP is integral to building robust and efficient applications. Leveraging the OOP paradigm allows developers to create modular, scalable, and maintainable code, contributing to the overall success of SAP projects.
Share your thoughts in the comments
Please Login to comment...