String manipulation is a fundamental skill in SAP ABAP programming language, and it’s crucial for dealing with various types of data processing, text formatting, and building dynamic content. This article aims to guide you through mastering string manipulation in SAP ABAP, providing insights, best practices, and code examples.
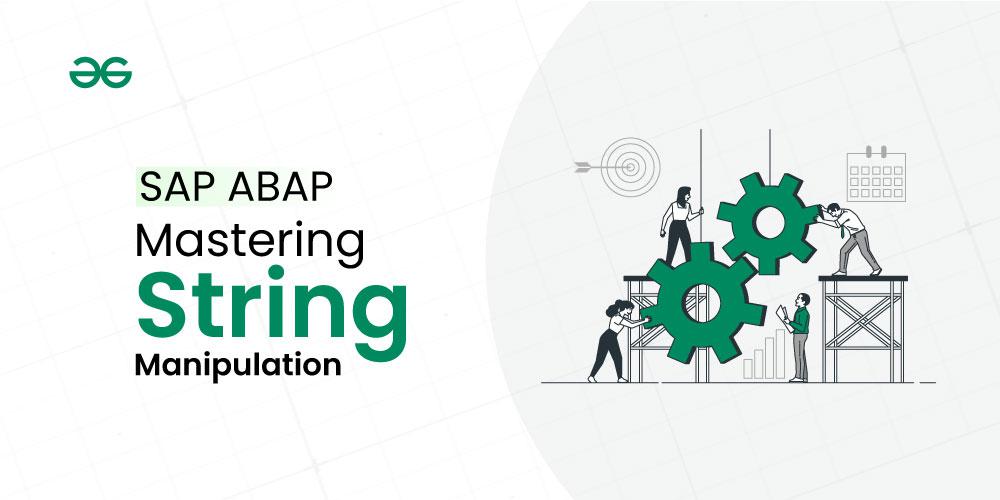
SAP ABAP | Mastering String Manipulation
Introduction to String Manipulation in SAP ABAP
String manipulation involves performing operations on strings, which are sequences of characters. In SAP ABAP, strings are typically used to store and process textual information, such as names, addresses, or any other text-based data. Mastering string manipulation is essential for tasks like data cleansing, formatting, and creating dynamic outputs.
Basic String Operations in SAP ABAP
Here are a few basic string operations in SAP ABAP:
1. Concatenation of String in SAP ABAP:
Concatenation of strings in SAP ABAP involves combining multiple strings into a single string. The CONCATENATE statement is commonly used for this purpose.
Example 1:
DATA(s1) TYPE string VALUE 'HELLO',
(s2) TYPE string VALUE 'WORLD' ,
(s3) TYPE string .
CONCATENATE s1 s2 INTO s3.
Output:
HELLOWORLD
In this example, s3 will hold the value “HELLO WORLD“
Example 2:
DATA: s1(10) VALUE 'John',
s2(10) VALUE 'USA',
s3(10) VALUE 30,
s4(30)
CONCATENATE s1 s2 s3 INTO s4
WRITE: / 'Full Information:', s4.
Output:
JohnUSA30
In this example, s4 will contain a string with concatenated information about a person, including their name, country, and age.
2. Substrings of String in SAP ABAP:
Extracting a portion of a string is known as creating a substring. The SUBSTRING statement allows you to get a specific part of a string based on the starting position and length.
DATA: str(30) VALUE 'GeeksForGeeks'.
DATA: str1(5) ,
str2(3),
str3(5).
str1 = str(5).
str2 = str+ 5(3).
str3 = str + 8(5).
WRITE: / str1.
WRITE: / str2.
WRITE: / str3.
Output:
Geeks
For
Geeks
3. Searching and Replacing of String in SAP ABAP:
3.1 Searching for Substrings of String in SAP ABAP :
You can check if a substring exists within a string using the CS and NS keywords.
Example 1:
DATA: str1(30) VALUE 'SAP ABAP',
str2(30) VALUE 'SAP'.
SEARCH str1 FOR str2.
IF sy-subrc =0.
WRITE:/ 'YES FOUND'.
ELSE
WRITE:/ 'NOT FOUND'.
ENDIF.
Output:
YES FOUND
3.2 Replacing Substrings of String in SAP ABAP:
To replace occurrences of a substring within a string, you can use the REPLACE statement.
DATA: str1(30) VALUE 'GFG WORLD',
str2(30) VALUE 'GFG'.
REPLACE 'SAP' WITH str2 INTO str1
WRITE: / str1
Output:
SAP WORLD
4.1 Uppercase and Lowercase of String in SAP ABAP:
Converting a string to uppercase or lowercase is straightforward using the CONVERT statement.
DATA(lv_original_string) TYPE string VALUE 'Hello, ABAP World!'.
DATA(lv_lowercase_string) TYPE string.
CONVERT TEXT lv_original_string INTO LOWER CASE lv_lowercase_string.
WRITE: / 'Original String:', lv_original_string,
/ 'Lowercase String:', lv_lowercase_string.
Output:
Original String: Hello, ABAP World!
Uppercase String: HELLO, ABAP WORLD!
In this example, lv_lowercase_string will contain the uppercase version of lv_original_string.
4.2 Padding of String in SAP ABAP:
Padding involves adding characters to the beginning or end of a string to achieve a desired length.
DATA(lv_string) TYPE string VALUE '123'.
DATA(lv_padded) TYPE string.
lv_padded = lv_string && '00'.
WRITE: / 'Original String:', lv_string,
/ 'Padded String:', lv_padded.
Output:
Original String: 123
Padded String: 12300
Here, lv_padded will have two zeroes appended to the end of lv_string.
5. Advanced Operations of String in SAP ABAP
5.1 Splitting Strings of String in SAP ABAP:
Splitting a string into components is useful when dealing with delimited data, such as CSV files. The SPLIT statement can be employed for this purpose.
DATA(lv_string) TYPE string VALUE 'apple,orange,banana'.
DATA lt_parts TYPE TABLE OF string.
SPLIT lv_string AT ',' INTO TABLE lt_parts.
WRITE: / 'Original String:', lv_string,
/ 'Split Parts:'.
LOOP AT lt_parts INTO DATA(lv_part).
WRITE: / lv_part.
ENDLOOP.
Output:
Original String: apple,orange,banana
Split Parts:
apple
orange
banana
In this example, the original string ‘apple,orange,banana’ is split at each comma, resulting in the internal table lt_parts containing individual parts. The WRITE statement is then used to display both the original string and the individual parts in the internal table.
5.2 Regular Expressions of String in SAP ABAP:
For more advanced string manipulation tasks, regular expressions can be employed. ABAP supports regular expressions through the FIND REGEX and REPLACE ALL OCCURRENCES OF REGEX statements.
DATA(lv_string) TYPE string VALUE 'Hello, ABAP World!'.
DATA(lv_result) TYPE abap_bool.
lv_result = lv_string CP '*ell*'.
WRITE: / 'Original String:', lv_string,
/ 'Pattern Match Result:', lv_result.
Output:
Original String: Hello, ABAP World!
Pattern Match Result: 1
In this example, the original string ‘Hello, ABAP World!’ is checked for the pattern *ell*. Since the pattern is found in the string, the result is 1 (true).
5.3 Length of a String in SAP ABAP:
Determining the length of a string can be done using the STRLEN function.
DATA(lv_string) TYPE string VALUE 'Hello, ABAP World!'.
DATA(lv_length) TYPE i.
lv_length = STRLEN( lv_string ).
WRITE: / 'Original String:', lv_string,
/ 'String Length:', lv_length.
Output:
Original String: Hello, ABAP World!
String Length: 18
In this example, the original string ‘Hello, ABAP World!’ is used, and the length of the string (number of characters) is calculated using the STRLEN function. The result, which is the length of the string, is then displayed using the WRITE statement. In this case, the string has 18 characters.
5.4 Count Occurrences of String in SAP ABAP:
To count the occurrences of a substring within a string, you can use the COUNT statement.
DATA(lv_string) TYPE string VALUE 'Hello, ABAP World!'.
DATA(lv_count) TYPE i.
lv_count = COUNT( lv_string FOR 'l' ).
WRITE: / 'Original String:', lv_string,
/ 'Count of ''l'':', lv_count.
Output:
Original String: Hello, ABAP World!
Count of 'l': 3
In this example, the original string ‘Hello, ABAP World!’ is used, and the number of occurrences of the character ‘l’ is calculated using the COUNT function.
5.5 Leading and Trailing Spaces of String in SAP ABAP:
Removing leading and trailing spaces from a string can be achieved using the CONCATENATE statement.
DATA(lv_string) TYPE string VALUE ' Hello, ABAP World! '.
DATA(lv_trimmed) TYPE string.
CONCATENATE lv_string INTO lv_trimmed RESPECTING BLANKS.
WRITE: / 'Original String:', lv_string,
/ 'Trimmed String:', lv_trimmed.
Output:
Original String: Hello, ABAP World!
Trimmed String: Hello, ABAP World!
In this example, the original string has multiple consecutive blanks. The CONCATENATE statement with the RESPECTING BLANKS addition trims the extra spaces and creates a new string (lv_trimmed) where consecutive blanks are reduced to a single blank.
5.6 Date to String of String in SAP ABAP:
Formatting a date into a string can be done using the WRITE statement with the TO keyword.
DATA(ld_date) TYPE sy-datum.
DATA(lv_date_string) TYPE string VALUE '12/31/2023'.
CONVERT DATE lv_date_string INTO ld_date.
WRITE: / 'Original String:', lv_date_string,
/ 'Converted Date:', ld_date.
Output:
Original String: 12/31/2023
Converted Date: 20231231
In this example, the original string ’12/31/2023′ is converted to a date format using the CONVERT DATE statement. The converted date is then displayed using the WRITE statement. Please replace the example string with the actual date string you are working with.
5.7 Time to String in SAP ABAP
Similarly, formatting a time into a string can be achieved.
DATA(lt_timestamp) TYPE timestamp.
DATA(lv_time_string) TYPE string VALUE '12:34:56'.
CONVERT TIME lv_time_string INTO TIME STAMP lt_timestamp TIME ZONE sy-zonlo.
WRITE: / 'Original String:', lv_time_string,
/ 'Converted Time:', lt_timestamp.
Output:
Original String: 12:34:56
Converted Time: 12:34:56
In this example, the original string ’12:34:56′ is converted to a time format using the CONVERT TIME statement. The converted time is then displayed using the WRITE statement.
Conclusion
Mastering string manipulation in SAP ABAP is essential for effectively working with textual data. Whether you need to concatenate strings, search for substrings, or apply advanced regular expressions, a solid understanding of string manipulation techniques will significantly enhance your ABAP programming skills. Regular practice and experimentation with various string operations will help you become proficient in handling diverse data scenarios in SAP ABAP.
Share your thoughts in the comments
Please Login to comment...