Programming languages have transformed the way we think about building systems and software. One such language is Rust, which is becoming more and more popular and impactful as the days go by. It is known for building robust systems capable of executing performance-intensive tasks. Moreover, it is regarded as a language that encourages memory safety.
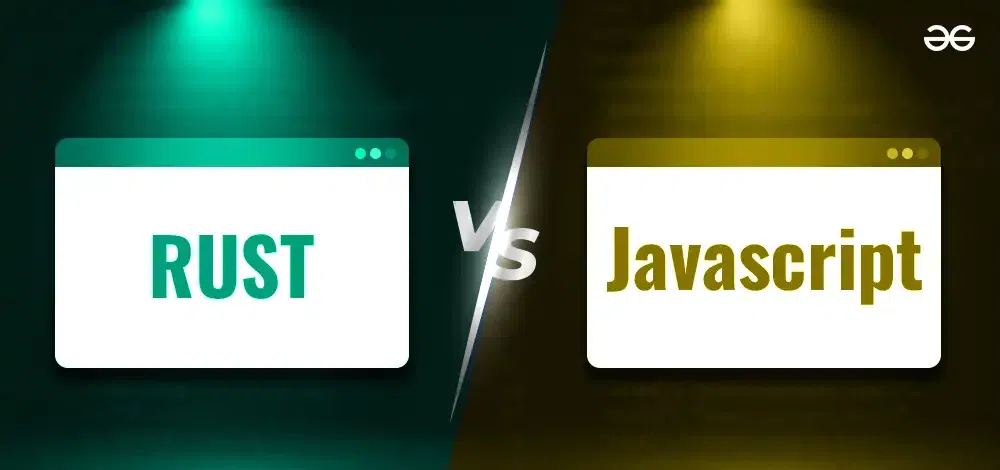
In recent years, Rust has been compared with JavaScript, an old but really powerful scripting language, also regarded as the “language of the web.” Both languages are great enough to perform their respective tasks but provide different approaches to different concepts. This article will discuss what Rust and JavaScript are and the differences between the two languages in detail.
What is Rust?
Rust is an immensely popular systems programming language that is fast and reliable. It is often used in sectors where high performance is a must. Therefore, it is used to create backend systems, operating systems, device drivers, kernels, and CLI tools. Along with this, it is also used in the cryptocurrency and blockchain fields, and now it is being used in the space of web development too.
Rust is a statically typed language developed at Mozilla Research in 2010, and it requires function arguments to have explicit types, which helps avoid bugs. It does not have garbage collection. It has a built-in package manager called Cargo and includes built-in support for concurrency.
Some examples of software written in Rust include:
- Next.js compiler
- Backend code of search feature in GitHub
- The online grading system of Coursera
What is JavaScript?
JavaScript is a single-threaded programming language used extensively in the field of web development. “Single-threaded” means the instructions are sequentially executed one by one. It also supports asynchronous programming, which enables developers to build highly interactive web applications.
JavaScript was created by Brendan Eich in 1995, and since then, it has powered loads of websites and millions of developers use it around the world. As a result, it has a huge and well-established community. Earlier, JavaScript used to run on the client but with Node.js, it can run on servers too.
Millions of developers use JavaScript and the field of web development is incomplete without this language. Giant companies like Google, Facebook, YouTube, Amazon, eBay, and LinkedIn use JavaScript in some form or another.
Rust vs JavaScript – The difference
Let’s take a look at what differentiates Rust from JavaScript. We will look at multiple departments to compare them.
1. Memory management
Rust uses the concept of “ownership” and a set of rules to manage memory in the program. Here, the developer has most of the control over memory management but Rust helps to check some of the rules. If the rules don’t comply, the program will not be compiled. Each value in Rust has an owner and when the owner moves out of scope, the memory is deallocated.
On the other hand, memory management in JavaScript happens automatically. This means memory is automatically allocated when the objects are created, and it is freed when the objects are not used anymore. This is the process of garbage collection and it is performed by a garbage collector.
The garbage collection mechanism has a couple of algorithms:
2. Variables and mutability
In Rust, all the variables are immutable by default. If the variable needs to be mutable, the keyword “mut” can be added in front of the variable. On the other hand, constants in Rust are always immutable and cannot be made mutable by adding “mut” in front of them.
In JavaScript, there are three ways a developer can declare variables:
- Var: Globally scoped variable, its value can be re-assigned and the variable can be redeclared.
- Let: Block scoped variable, its value can be changed once declared but the variable cannot be redeclared in the same scope.
- Const: Cannot be assigned a new value. The value cannot be changed once declared.
For simplicity, a let variable in Rust behaves like a const variable in JavaScript. A let with a mut keyword in Rust is similar to a let variable in JavaScript.
3. Functions
In Rust, functions are defined using the fn keyword before the name of the function. Since Rust is a statically typed language, it is required to specify the type of the parameters during function declaration. During compile-time, types of these parameters are checked, and that helps in finding any errors related to type.
Functions in JavaScript can be written in either the normal way, i.e., by writing the function keyword before the function name, or by using the arrow function syntax. JavaScript is dynamically typed, so types of parameters are not required. As a result, function parameters in JavaScript can hold values of any type.
4. Concurrency
Concurrency is a concept where multiple tasks with different goals can continue to be executed simultaneously or at the same time.
In Rust, concurrency is handled by the concept of ownership, which is a set of rules to determine how memory is managed in Rust. In addition, it uses the concept of borrowing, where a function transfers the control to another function for a while. Rust also supports the creation of multiple threads, which allows it to run concurrent tasks.
JavaScript uses the concepts of event loops and callbacks to handle concurrency. The event loop is very crucial to performing asynchronous programming in JavaScript as all the operations can be executed in a single thread. JavaScript itself is single-threaded. However, the browser can handle asynchronous operations using features like Web Workers for parallelism. With the browser API, multi-threading can be achieved as the browser APIs can handle tasks without blocking the main thread.
5. Error handling
Error handling is a must when we deal with programming languages. If the error is not handled, the program can crash, leading to a poor user experience.
Rust does not have exceptions. It uses the enum “Result” type to handle recoverable errors that have a couple of variants, “Ok” and “Err,” and it takes 2 parameters, T and E.
- T represents the type of value that is returned in the “OK” variant (success).
- E is the error type that is returned in the case of failure, i.e., the “Err” variant.
Rust
enum Result<T, E> {
Ok(T),
Err(E),
}
|
JavaScript handles errors with a try-catch block. Firstly, the error can be thrown using the “throw” keyword and it is handled in the “catch” block. When the error is thrown, the control of the program reaches the catch block, which receives an error object. The error object contains details about the error, mainly, the type and the error message. It is up to the developer to do a task with the error in the catch block.
Javascript
function print(a) {
if (a < 0) {
throw new Error( "Cannot print a negative number" );
}
return a;
}
try {
let result = print(-2);
console.log(result);
} catch (error) {
console.log(error.message);
}
|
The above code will throw an error and the control of the program will transfer over to the catch block.
6. Modules
A module is simply a file that developers can use to distribute the code and increase its maintainability.
Rust doesn’t recognize a file as a module just because it is a file. It requires the developers to explicitly build the module tree. This means that to use any file inside another file, developers need to define it as a submodule. This can be done with the “mod” keyword. Also in Rust, by default everything is private, so if we want to use a function, we have to declare it as public. Rust, items (functions, structs, etc.) are private by default at the module level, but they can be explicitly marked as public.
Rust
mod config;
fn main() {
config::print_config_name();
println!( "main.rs" );
}
pub fn print_config_name() {
println!( "config.rs" );
}
|
JavaScript uses special terms such as “export” and “import” to use different files or call a function from another file into the code.
- Export is used when the variables and functions are needed to be used in another file.
- Import is used when those functions and variables are to be used in the current file.
Javascript
export function displayName (name) => {
console.log(name);
};
import {displayName} from "./displayName.js" ;
displayName( "John Doe" );
|
Comparison summary
Here’s a table depicting how both languages compare with each other:
Characteristics |
Rust |
JavaScript |
Syntax |
Strict |
Not strict |
Memory management |
Manual |
Automatic |
Typing |
Statically typed |
Dynamically typed |
Data types |
Larger number of data types |
A smaller number of data types |
Mutability |
Variables are immutable by default |
Primitive data types are immutable and non-primitive data types are mutable |
Concurrency |
Concepts of ownership and borrowing |
Concepts of event loop and asynchronous programming |
Error handling |
No exceptions |
Throws exceptions |
Community |
Relatively smaller compared to JavaScript |
Well-established and massive community |
Ecosystem |
Cargo is used, growing day by day |
Millions of libraries are already used by various developers |
Applications |
Performance-heavy systems |
Web development, server-side scripting |
Conclusion
Both Rust and JavaScript are heavyweights in various departments, and both are capable of building robust and efficient products. Performance-heavy systems are built using Rust, while feature-loaded software, websites, and advanced servers are built using JavaScript.
In this article, we discussed what Rust and JavaScript are, how they compare different functionalities and concepts, and the comparison summary in tabular form. While both languages are capable enough, it is essential to understand the project goals and requirements to choose a language between the two.
Share your thoughts in the comments
Please Login to comment...