This language has come a long way and overcome numerous challenges in software engineering, for example, those stemming from its changing nature; thus reasserting its resilience and flexibility. Stroustrup originally developed C++ as an extension of C programming language during the late 1970s to ensure efficiency and low-level control while being more abstract.
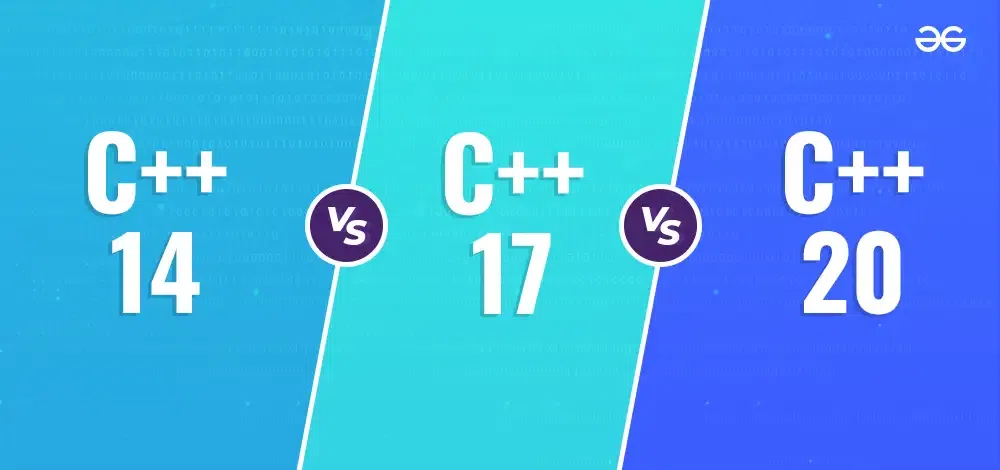
These functions have introduced new features, improved performance, and optimizations in various iterations. Such iterations are necessary to keep abreast with the times and tackle emerging requirements and issues regarding contemporary software development
1. C++14 Programming Language
C++14 was another important milestone in this evolution in language design that was released in 2014. Unlike previous versions like C++11 which had breakthrough features; it didn’t introduce any new developments but rather included many of them that facilitate faster work by programmers, better coding, and more powerful programming
Key Features
1. Generic lambdas
The use of generic lambdas by developers is instrumental in making shortest codes look more expressive. Utilizing generic lambda functions leads to the creation of ones that can work on different types of inputs without specifically stating template parameters.
auto sum = [](auto a, auto b) { return a + b; };
2. Relaxation of constexpr Restrictions
The use of the constexpr specifier in C++11 indicated that a function or variable could be evaluated during compilation, but C++14 relaxed some of the rules that governed a constexpr function, thereby making it more flexible.
constexpr int square(int x) {
return x * x;
}
3. Binary Literals:
With help from 0b prefix, binary literals are supported in C++14 as they allow direct representation of binary numbers within source code.
int binaryNumber = 0b1010;
4. Automatic Return Type Deduction for Functions
Introduced in C++11 and continued in C++14, auto return type deduction for functions makes the compiler to deduce the return type from a function’s return statement.
auto add(int a, int b) {
return a + b; // Compiler deduces return type as int
}
Apart from others, these characteristics were responsible for making C++, as a language, more expressive and also helped in reducing the length of C++ code as well as maintaining it.
2. C++17 Programming Language
C++14 was made better by C++17 that came out in 2017 and included several more features meant to improve its capabilities and solve some problems that programmers regularly encounter.
Key Features
1. Inline Variables
In C++17, inline specifier was added to allow variables to be defined and initialized in header files without violating One Definition Rule (ODR).
inline int x = 10;
2. Structured Bindings
With structured bindings, objects can be divided into their constituent parts so as to make application with complex structures like structs and tuples simpler.
std::tuple<int, std::string> data{42, "hello"};
auto [number, message] = data;
3. constexpr if Statements
Additionally, there are constexpr if statements which introduce conditional execution during compile time based on template parameters and other compile-time constants which is another feature introduced by C++17.
template<typename T>
void printSize(T value) {
if constexpr (sizeof(T) <= 4) {
std::cout << "Size is less than or equal to 4 bytes\n";
} else {
std::cout << "Size is greater than 4 bytes\n";
}
}
4. Fold Expressions
Fold expressions provide an elegant syntax for combining a pack of variadic template arguments together with a binary operator.
template<typename... Args>
auto sum(Args... args) {
return (args + ...);
}
These changes were part of a bigger effort to modernize C++, among other things so as to improve productivity of developers even further.
3. C++20 Programming Language
Also, another advancement called C++20 came with simplified, readable codes, enhancing its capability as a language again in 2020 so as to make it fast.
Key Features
1. Concepts
Concepts became more expressive and easier to use in C++20 via concepts feature that allows developers to impose constraints on their template parameters.
template<typename T>
concept Integral = std::is_integral_v<T>;
template<Integral T>
void print(T value) {
std::cout << value << '\n';
}
2. Ranges Library
The Ranges Library is a set of programs which consist of views, algorithms, or adaptors meant for making such boring tasks as range transformation or iteration simple.
std::vector<int> numbers{1, 2, 3, 4, 5};
auto evenNumbers = numbers | std::views::filter([](int x) { return x % 2 == 0; });
3. Coroutines
The support of coroutines in C++20 enables developers to write asynchronous code naturally with the help of co_await and co_yield keywords.
generator<int> fibonacci() {
int a = 0, b = 1;
while (true) {
co_yield a;
std::tie(a, b) = std::make_tuple(b, a + b);
}
}
4. Modules
In C++20, there is another way code can be organized and encapsulated: modules build faster, manage dependencies better, and offer stronger encapsulation compared to traditional header files.
export module mymodule;
export int add(int a, int b) {
return a + b;
}
Differences Between C++14, C++17, and C++20
Feature
|
C++14
|
C++17
|
C++20
|
Generic Lambdas
|
Introduced the ability to create functions that can operate on different data types without specifying them explicitly.
|
Continued support for generic lambdas.
|
Continued support for generic lambdas.
|
Relaxation of constexpr Restrictions
|
Allowed more flexibility in using constexpr for compile-time evaluation of functions and variables.
|
Continued to relax restrictions on constexpr, making it more versatile.
|
Continued relaxation of constexpr restrictions.
|
Binary Literals
|
Introduced support for directly representing binary numbers in the code using the 0b prefix.
|
Continued support for binary literals.
|
Continued support for binary literals.
|
Automatic Return Type Deduction
|
Introduced the auto return type deduction for functions, allowing the compiler to deduce the return type based on the return statement.
|
Continued support for automatic return type deduction.
|
Continued support for automatic return type deduction.
|
Inline Variables
|
N/A
|
Introduced the ability to define and initialize variables directly in header files using the inline specifier.
|
Continued support for inline variables.
|
Structured Bindings
|
N/A
|
Introduced the ability to decompose objects into their individual members, making it easier to work with complex data structures.
|
Continued support for structured bindings.
|
constexpr if Statements
|
N/A
|
Introduced the constexpr if statement, allowing for compile-time conditional execution based on template parameters and other compile-time constants.
|
Continued support for constexpr if statements.
|
Fold Expressions
|
N/A
|
Introduced a concise syntax for applying a binary operator to a pack of variadic template arguments.
|
Continued support for fold expressions.
|
Concepts
|
N/A
|
N/A
|
Introduced the ability to specify constraints on template parameters, improving template usage and error messages.
|
Ranges Library
|
N/A
|
N/A
|
Introduced a comprehensive library for working with ranges of elements, simplifying common tasks like iteration and transformation.
|
Coroutines
|
N/A
|
N/A
|
Introduced support for writing asynchronous code in a more natural and efficient manner.
|
Modules
|
N/A
|
N/A
|
Introduced a new way of organizing and encapsulating code, offering improved build times and dependency management.
|
Conclusion
C++14, C++17 and C++20 evolved in a way that showcases progress and purification in their lifetime; each iteration with new features, improvements and optimizations that are meant to improve development productivity, code readability and general language expressiveness.
Developers can exploit these differences to enable them use all available resources of C++ language for creating efficient, maintainable, and robust applications for today’s software development life cycle. While still undergoing its metamorphosis, C++ remains the backbone of programming, providing the basic building blocks from small utilities to large scale enterprise applications
FAQs on The Key Differences Between C++14, C++17, and C++20
What significance do generic lambdas have in C++14?
Generic lambdas allow the creation of lambda functions that can operate on different data types without needing explicit template parameters; hence the code is shorter and more flexible.
How does C++14 improve constexpr usage compared to C++11?
In contrast to C++11, C++14 provides some degree of relaxation of restrictions on constexpr functions so that they become more flexible for their applications and thus are capable of performing greater compile-time calculations.
Explain what binary literals brought about by C++14 are used for?
There can be binary numbers directly represented within the code by using a prefix 0b when the programers utilize binary literals, so simplifying bitwise operations in addition to increasing readability of code.
What’s about inline variables introduced in C++17?
Optimized definition of variables inside header files is simplified using inline variable which aid in reducing compilation time as well as organization of codes.
How does C++17 structured bindings improve code readability?
Structured bindings fragmentize the object into its parts making codes easier to understand in case of complex data structures.
Share your thoughts in the comments
Please Login to comment...